Watchdogs
Since the runtime of many image processing algorithms depends on the image content, function calls can take much longer than usual under unfavorable circumstances.
This can be caused, for example, by changing lighting conditions, unexpected objects in the image and other factors that can drastically influence the calculation time of functions such as F_VN_FindContours(). If, for example, only 10 contours can be found under normal conditions, with other lighting conditions there may be 100 or more.
This can lead to cycle overruns, which must be avoided at all costs, as they lead to undefined behavior.
Watchdogs are helpful for this purpose. Watchdogs can abort individual Vision functions or entire code sections with several functions after a certain time. Some TwinCAT Vision functions can be aborted during execution and return the partial results calculated up to that point. If several functions are monitored by a watchdog, all remaining functions within the watchdog range are skipped when the specified time has elapsed. However, the subsequent functions (after the watchdog area) are executed normally again.
There are two functions available to define the start of a watchdog-monitored area, which differ in terms of when the specified time starts to count:
- F_VN_StartAbsWatchdog defines an absolute abort time relative to the start of the task cycle
- F_VN_StartRelWatchdog defines a relative abort time relative to the start of the watchdog area
In both cases, the end of the area is defined by the function F_VN_StopWatchdog, which optionally returns the handling component and the required time.
Notice | |
Watchdog with relative abort time When using |
In order for functions to be monitored by a watchdog, the option "Watchdog stack" must be enabled on the respective executing task.
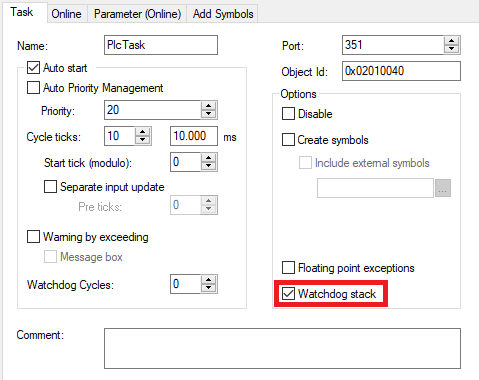
Sample
In the sample below, a watchdog is started with a stop time of 10 ms relative to the call of the watchdog function. If, for example, the cycle time is 20 ms, 4 ms have already passed in the current task cycle when F_VN_StartRelWatchdog is called and the watchdog is started with a stop time tStop of 10 ms relative to the current time, then the watchdog stops the monitored functions (i.e. any function called between the start and stop of the watchdog) after 14 ms of the current cycle have elapsed.
VAR
ipImage : ITcVnImage := 0;
ipContours : ITcVnContainer := 0;
// watchdog runtime info
nFunctionsMonitored : ULINT;
nFractionProcessed : UDINT;
tRest : DINT;
END_VAR
(* imagine some other functions that use 4ms up to here *)
hr := F_VN_StartRelWatchdog(10000, hr); // 10ms
hr := F_VN_Threshold(ipImage, ipImage, 120, 255, TCVN_TT_BINARY, hr);
hr := F_VN_FindContours(ipImage, ipContours, hr);
hr := F_VN_StopWatchdog(nFunctionsMonitored => nFunctionsMonitored, nFractionProcessed => nFractionProcessed, tRest => tRest);
Now two situations are possible:
- Both functions are terminated in time – this is the normal case.
Suppose F_VN_Threshold takes 1 ms and F_VN_FindContours takes 5 ms. When F_VN_StopWatchdog is called, 4 ms remain. The watchdog runtime information is:
nFunctionsMonitored = 2
nFractionProcessed = 100 // in %
tRest = 4000 // in us, equals 4ms
- The watchdog must intervene.
Scenario: The function F_VN_Threshold is not dependent on the image content (only on the number of pixels) and therefore only takes 1 ms, but the lighting conditions have changed unfavorably, so that F_VN_FindContours would take longer than 9 ms. Therefore the watchdog stops F_VN_FindContours, which nevertheless returns the contours found so far. The watchdog runtime information could then be as follows:
nFunctionsMonitored = 2
nFractionProcessed = 70
tRest = -50
In this case, an estimated handling percentage of 70% was calculated when the function was aborted. The remaining time is negative, i.e. the planned stop time was exceeded by 50 us, so that after the function F_VN_StopWatchdog 14050 us have passed since the start of the task cycle, instead of the planned 14000 us. This overrun is due to the fact that the partial results of the function that have already been calculated are to continue to be used. Therefore, on the one hand the algorithm can only be aborted at specific points, on the other hand the previous results must be organized and returned. The maximum overrun generally depends on the specific function and the image content. In a program, the termination time should therefore always be selected such that a safety buffer remains at the end of the task cycle.
Monitored functions
The following functions provide partial results in the event of a watchdog termination:
- F_VN_AdaptiveThreshold(Exp)
- F_VN_ConvertColorSpace
- F_VN_DetectBlobs(Exp)
- F_VN_FindContourHierarchyExp
- F_VN_FindContours(Exp)
- F_VN_HoughCircle(Exp)
- F_VN_LocateCircularArc(Exp)
- F_VN_LocateEdge(Exp)
- F_VN_LocateEdges(Exp)
- F_VN_LocateEllipse(Exp)
- F_VN_MatchTemplate(Exp)
- F_VN_MatchTemplateAndEvaluate(Exp)
- F_VN_MeasureAngleBetweenEdges(Exp)
- F_VN_MeasureEdgeDistance(Exp)
- F_VN_MeasureMinEdgeDistance(Exp)
- F_VN_NormalizeImageForDisplay
- F_VN_ReadDataMatrixCode(Exp)
- F_VN_ReferenceColorSimilarity(Exp)_ITcVnColorModel (_ITcVnMlModel)
- F_VN_ReferenceColorSimilarity(Exp)_TcVnVector3_LREAL
- F_VN_ResizeImage
- F_VN_Threshold
- F_VN_TrainImageColor(Exp(2))
- F_VN_WarpAffine(Exp)
- F_VN_WarpPerspective(Exp)
Other functions cannot be aborted during the execution, but will be skipped if the watchdog time has expired.