Triggering an image by file name
In this sample an image is purposefully triggered on the basis of the file name in the function block FB_VN_FileSourceControl.
Application
The sample displays alternately two images with the file names Image1.bmp and Image2.bmp from the file source in ADS Image Watch. The sample includes the images with corresponding file names. You can replace the sample images with your own images or add them additionally. To do this, change or add to the file names in sFileName
and adjust the number of elements if necessary. Note that all images to be displayed must be added in the File Source Control. By setting bLoop
to false
you stop the automatic switching of images and then you can select the image to be triggered by setting nImageIndex
.
Program
hr : HRESULT;
sFileName : ARRAY [0..1] OF STRING := ['Image1.bmp', 'Image2.bmp'];
fbFileSource : FB_VN_FileSourceControl;
eState : ETcVnCameraState;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
bTrigger : BOOL := TRUE;
bLoop : BOOL := TRUE;
nImageIndex : UINT := 0;
fbFileSource | Function block for controlling the File Source of the type FB_VN_FileSourceControl. |
eState | State of the File Source |
ipImageIn | Input image |
ipImageInDisp | Displayable input image |
bTrigger | Trigger flag |
sFileName | File name – change this variable in order to load a different image. However, this image must be added in the File Source Control. |
hr | Status variable of the type HRESULT. |
In general, the usual state machine is used to operate the file source:
eState := fbFileSource.GetState();
CASE eState OF
<...>
END_CASE
Resetting in case of errors and the starting of the image acquisition take place just as normal in the corresponding states:
TCVN_CS_INITIAL, TCVN_CS_INITIALIZING, TCVN_CS_INITIALIZED, TCVN_CS_OPENING, TCVN_CS_OPENED, TCVN_CS_STARTACQUISITION:
fbFileSource.StartAcquisition();
TCVN_CS_ERROR:
fbFileSource.Reset();
However, so that the file source doesn't load images immediately when the image acquisition is started, the Trigger Mode must be activated in the File Source Control:
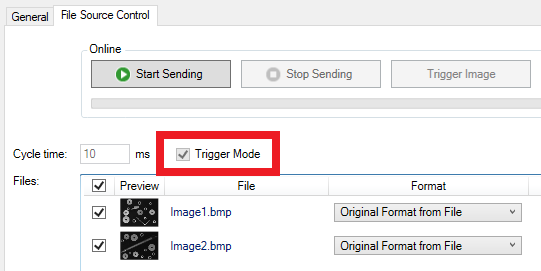
In the ACQUIRING
state, the image with the corresponding file name will now be alternately triggered and then received. The method TriggerImageByName is explicitly used here to trigger the image. This method exists only in the function block FB_VN_FileSourceControl and not in FB_VN_SimpleCameraControl.
TCVN_CS_ACQUIRING:
IF bTrigger THEN
hr := fbFileSource.TriggerImageByName(sFileName[nImageIndex]);
IF SUCCEEDED(hr) THEN
bTrigger := FALSE;
IF bLoop THEN
nImageIndex := nImageIndex + 1;
IF nImageIndex >= 2 THEN
nImageIndex := 0;
END_IF
END_IF
END_IF
ELSE
hr := fbFileSource.GetCurrentImage(ipImageIn);
IF SUCCEEDED(hr) AND ipImageIn <> 0 THEN
bTrigger := TRUE;
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, hr);
END_IF
END_IF
As the File Source temporarily enters the TRIGGERING
state when triggering the image, triggering must continue in this state. This continues until the File Source has fully loaded the image and thus changed its state back to ACQUIRING
. The file name of the image does not need to be transferred again for further triggering; it is sufficient to call the method TriggerImage.
TCVN_CS_TRIGGERING:
fbFileSource.TriggerImage();
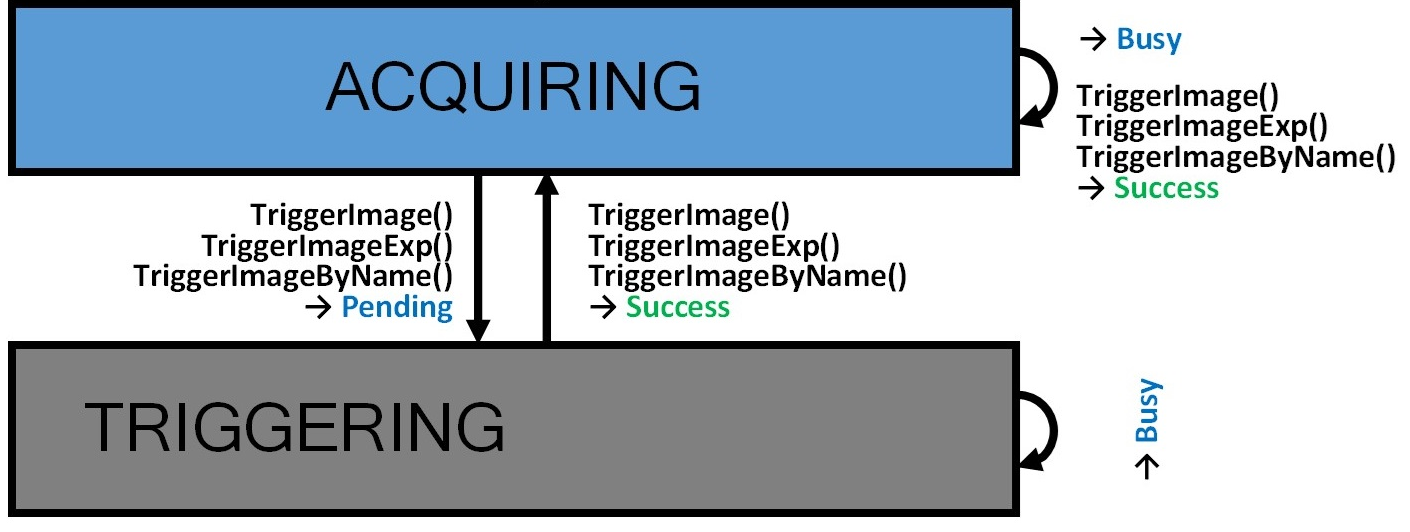
In this sample, the variable bTrigger
is automatically set to TRUE
for demonstration purposes when an image has been successfully loaded. In a real application the trigger would be set at a point that makes sense in terms of the sequence.