FB_VN_FileSourceControl
This FB provides access to the images send by a File Source instance.
Do not call the main FB directly. Only use the available methods.
Methods
Name |
Description |
---|---|
Gets the current available image (if any). | |
Gets the current available image (if any) and the corresponding file name. | |
Activates the File Source. If not in trigger mode, it will start sending images. | |
Deactivates the File Source. It will stop sending images. | |
Reset the controller to initial state (might require multiple calls depending on current state, until S_OK is returned) | |
Gets the current state of the internal camera control state machine. | |
If in trigger mode, this function triggers the next image in the list. | |
If in trigger mode, this function triggers a specific image in the list. | |
If in trigger mode, this function triggers a specific image in the list by name. |
Further information
The function block FB_VN_FileSourceControl
can control File Source instances with the full range of functions in the PLC. Alternatively, this is also possible with limited functionality with the function block FB_VN_SimpleCameraControl.
State machine
The following diagram shows the state machine. The main states are marked in blue, the transition states are gray and the error state is red.
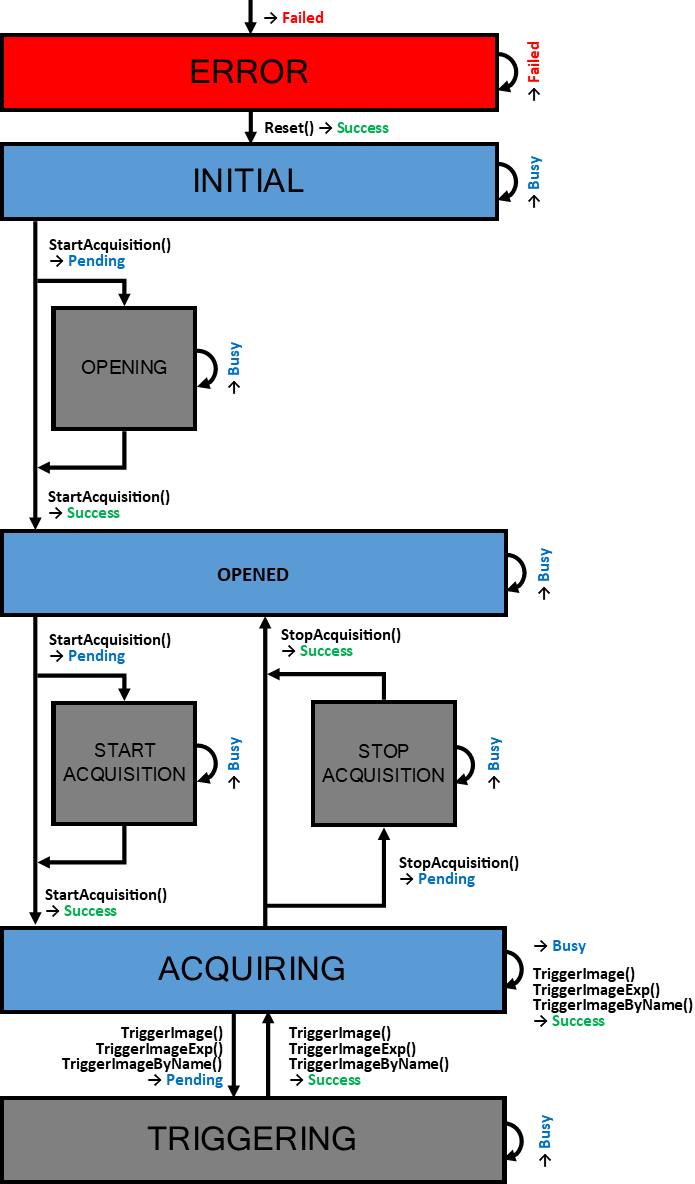
The following methods can be used to control the state machine:
If a method is called in a state for which it is not intended, the error code INVALIDSTATE
(16#712
) is returned.
Application with continuous image acquisition
A state machine that continuously retrieves received images looks like this, for example:
VAR
fbFileSource : FB_VN_FileSourceControl;
eState : ETcVnCameraState;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
hr : HRESULT;
nNewImageCounter: UINT;
END_VAR
eState := fbFileSource.GetState();
CASE eState OF
TCVN_CS_INITIAL, TCVN_CS_OPENING, TCVN_CS_OPENED, TCVN_CS_STARTACQUISITION:
hr := fbFileSource.StartAcquisition();
TCVN_CS_ACQUIRING:
hr := fbFileSource.GetCurrentImage(ipImageIn);
// Check if new Image was received
IF SUCCEEDED(hr) AND ipImageIn <> 0 THEN
nNewImageCounter := nNewImageCounter + 1;
// Place to call vision algorithms
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, hr);
END_IF
TCVN_CS_ERROR:
hr := fbFileSource.Reset();
END_CASE
Application with trigger
A state machine that continuously triggers an image with a special file name looks like this, for example:
VAR
fbFileSource : FB_VN_FileSourceControl;
eState : ETcVnCameraState;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
bTrigger : BOOL := TRUE;
sFileName : STRING := 'FileName.bmp';
hr : HRESULT;
nNewImageCounter: UINT;
END_VAR
eState := fbFileSource.GetState();
CASE eState OF
TCVN_CS_INITIAL, TCVN_CS_OPENING, TCVN_CS_OPENED, TCVN_CS_STARTACQUISITION:
hr := fbFileSource.StartAcquisition();
TCVN_CS_TRIGGERING:
hr := fbFileSource.TriggerImage();
TCVN_CS_ACQUIRING:
IF bTrigger THEN
hr := fbFileSource.TriggerImageByName(sFileName);
IF SUCCEEDED(hr) THEN
bTrigger := FALSE;
END_IF
ELSE
hr := fbFileSource.GetCurrentImage(ipImageIn);
// Check if new Image was received
IF SUCCEEDED(hr) AND ipImageIn <> 0 THEN
nNewImageCounter := nNewImageCounter + 1;
// Trigger next image
bTrigger := TRUE;
// Place to call vision algorithms
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, hr);
END_IF
END_IF
TCVN_CS_ERROR:
hr := fbFileSource.Reset();
END_CASE
As an alternative to the TriggerImageByName(sFileName)
method, the TriggerImage()
can be used to always get the next image from the File Source Control list.
Samples
Related function blocks
- FB_VN_SimpleCameraControl for cameras and file sources
- FB_VN_GevCameraControl for GigE Vision cameras
- FB_VN_FileSourceControl for file sources
Required License
TC3 Vision Base
System Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT V3.1.4024.59 or later | PC or CX (x64) with min. PL50, e.g. Intel 4-core Atom CPU | Tc3_Vision |