FB_VN_SimpleCameraControl
This FB provides the basic functionality to control a camera or a FileSource.
Do not call the main FB directly. Only use the available methods.
Methods
Name |
Description |
---|---|
Get the current available image (if any). | |
Get the current state of the internal camera control state machine. | |
Reset the camera to initial state (might require multiple calls depending on current state, until S_OK is returned) | |
Start the image acquisition. | |
Stop the image acquisition. | |
Trigger the next image. |
Further information
The function block FB_VN_SimpleCameraControl
combines the common features of FB_VN_GevCameraControl and FB_VN_FileSourceControl. This means that instances of the function block can be linked to both the Image Provider of a camera and the Image Provider of a File Source Control, as in First Steps.
State machine
The following diagram shows the state machine. The main states are marked in blue, the transition states are gray and the error state is red. In contrast to a File Source Control, a camera always also assumes the transition state TCVN_CS_OPENING
during the transition from TCVN_CS_INITIAL
to TCVN_CS_ACQUIRING
and, if necessary, also the main state TCVN_CS_OPENED
. If Init Commands are present and the Initialization Auto Mode AUTOINIT_AFTER_SO
has been selected, the INITIALIZING
state in which the automatic initialization takes place is also briefly assumed. If a transition occurs very quickly, the corresponding state is not reported to the outside, because the subsequent state has already been reached within a cycle. When linked to a File Source Control, these three states are not assumed. The use of the Trigger Image method from the TCVN_CS_OPENED
state is only possible with one camera.
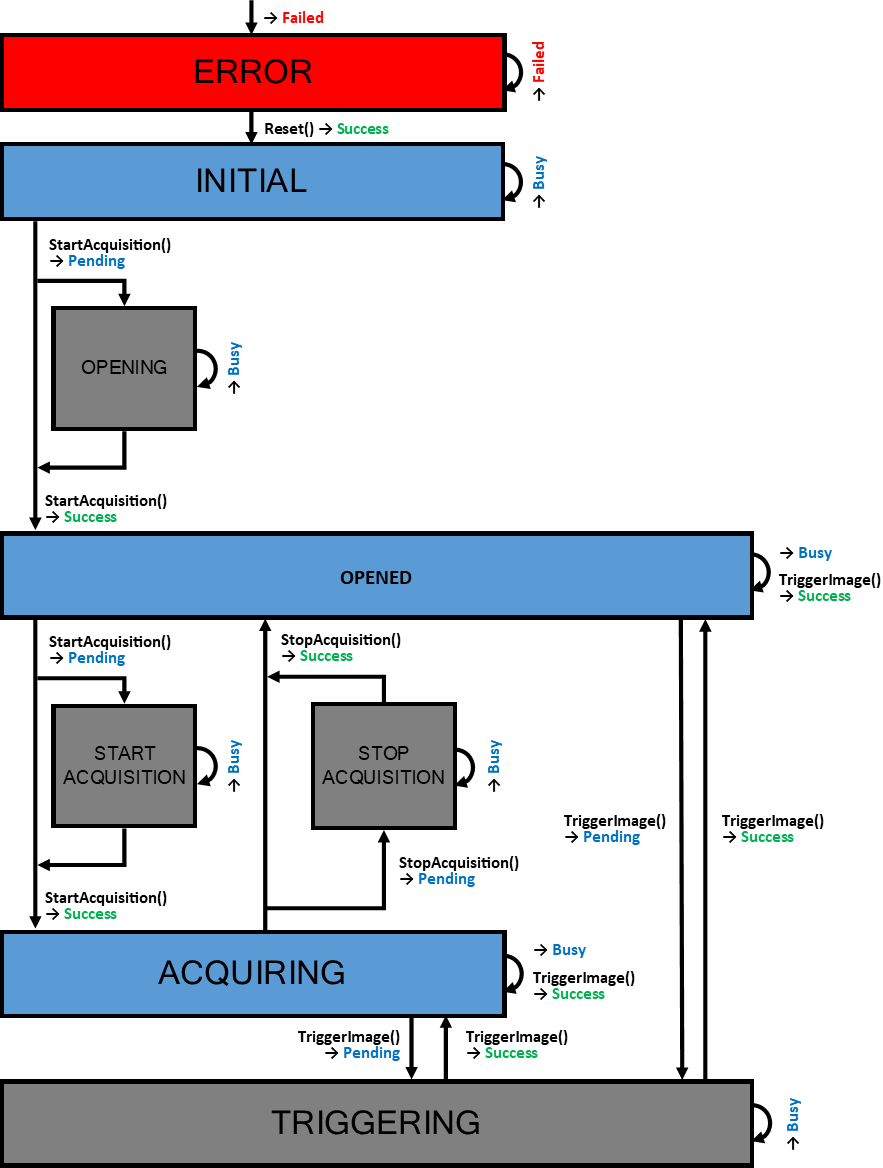
The following methods can be used to control the state machine:
If a method is called in a state for which it is not intended, the error code INVALIDSTATE
(16#712
) is returned.
Application with continuous image acquisition
A state machine for placing a camera in image acquisition mode, handling error states and continuously displaying successfully captured images looks like this, for example:
VAR
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
fbCameraControl : FB_VN_SimpleCameraControl;
eCameraState : ETcVnCameraState;
nNewImageCounter: UINT;
END_VAR
eCameraState := fbCameraControl.GetState();
// CameraControl is in error state, so try to reset the camera connection
IF eCameraState = TCVN_CS_ERROR THEN
hr := fbCameraControl.Reset();
// Camera not yet streaming
ELSIF eCameraState < TCVN_CS_ACQUIRING THEN
hr := fbCameraControl.StartAcquisition();
// Camera streaming
ELSIF eCameraState = TCVN_CS_ACQUIRING THEN
hr := fbCameraControl.GetCurrentImage(ipImageIn);
// Check if new Image was received
IF SUCCEEDED(hr) AND ipImageIn <> 0 THEN
nNewImageCounter := nNewImageCounter + 1;
// Place to call vision algorithms
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, hr);
END_IF
END_IF
In this case, the camera can be configured to continuous image acquisition or to a hardware trigger.
Application with software trigger
A state machine for putting a camera into image acquisition mode, handling error states and triggering image acquisition via software trigger looks like this, for example:
VAR
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
fbCameraControl : FB_VN_SimpleCameraControl;
eCameraState : ETcVnCameraState;
nNewImageCounter: UINT;
bTrigger : BOOL := TRUE;
END_VAR
eCameraState := fbCameraControl.GetState();
// CameraControl is in error state, so try to reset the camera connection
IF eCameraState = TCVN_CS_ERROR THEN
hr := fbCameraControl.Reset();
// Camera trigger image
ELSIF eCameraState = TCVN_CS_TRIGGERING THEN
hr := fbCameraControl.TriggerImage();
// Camera not yet streaming
ELSIF eCameraState < TCVN_CS_ACQUIRING THEN
hr := fbCameraControl.StartAcquisition();
// Camera streaming
ELSIF eCameraState = TCVN_CS_ACQUIRING THEN
IF bTrigger THEN
hr := fbCameraControl.TriggerImage();
IF SUCCEEDED(hr) THEN
bTrigger := FALSE;
END_IF
ELSE
hr := fbCameraControl.GetCurrentImage(ipImageIn);
// Check if new Image was received
IF SUCCEEDED(hr) AND ipImageIn <> 0 THEN
nNewImageCounter := nNewImageCounter + 1;
// Trigger next image
bTrigger := TRUE;
// Place to call vision algorithms
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, hr);
END_IF
END_IF
END_IF
In this case, the camera must be configured to a software trigger (TriggerSource = Software
).
Samples
In many function samples, a FB_VN_SimpleCameraControl
function block is used to load sample images.
Related functions
- FB_VN_SimpleCameraControl for cameras and file sources
- FB_VN_GevCameraControl for GigE Vision cameras
- FB_VN_FileSourceControl for file sources
Required License
TC3 Vision Base
System Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT V3.1. 4024.44 or later | PC or CX (x64) with PL50, e.g. Intel 4-core Atom CPU | Tc3_Vision |