Result evaluation during Code Reading
This sample illustrates the following
- One EAN13 code is read per image using the function F_VN_ReadBarcode
- The execution time of the function is monitored via a watchdog.
- Handling of different return codes of the function.
Explanation
- The function F_VN_ReadBarcode can search and read barcodes of different types in the image. Details of the use of the function can be found in the sample EAN-13 Barcode Reading.
- The handling of the return code of the function is explained using a corresponding query. A distinction is made between the following cases:
- Successful execution of the function, code was found and decoded.
- Successful execution of the function, code is not present in the image or cannot be found / decoded.
- The function itself has no error and was aborted by the watchdog.
- Function has other error.
You can find a detailed explanation of the return codes for Code Reading functions in the overview chapter Code Reading.
Variables
hr : HRESULT;
// Images
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// Barcode
ipCodeDecodedList : ITcVnContainer;
ipCodeContourList : ITcVnContainer;
sCodeAsString : STRING(255);
// Watchdog
hrWD : HRESULT;
tStop : DINT := 50000;
tRest : DINT;
// Color
aColorGreen : TcVnVector4_LREAL := [0, 255, 0];
// Return code
nReturnCode : DWORD;
sReturnCode : STRING;
sResultText : STRING;
Code
// Execute the Barcode Reading Function with EAN13 selected monitored by the Watchdog-Function
hrWD := F_VN_StartRelWatchdog(tStop, S_OK);
hr := F_VN_ReadBarcode(
ipSrcImage := ipImageIn,
ipDecodedData := ipCodeDecodedList,
eBarcodeType := ETcVnBarcodeType.TCVN_BT_EAN13,
hrPrev := hr
);
hrWD := F_VN_StopWatchdog(hrWD, tRest => tRest);
// Handle return-code
IF hr = S_OK THEN
// Prepare code result in string
hr := F_VN_ExportSubContainer_String(ipCodeDecodedList, 0, sCodeAsString, 255, hr);
sResultText := CONCAT('Code: ', sCodeAsString);
ELSE
// Check for succeeded return codes or add specific error handling
CASE hr OF
S_FALSE:
sResultText := 'No code found...';
S_WATCHDOG_TIMEOUT:
sResultText := 'Cancelled by watchdog...';
ELSE
// Extract error-code from HRESULT & react accordingly
nReturnCode := DINT_TO_DWORD(hr) AND 16#FFF;
sReturnCode := DWORD_TO_HEXSTR(nReturnCode, 3, FALSE);
sResultText := CONCAT('Returncode ', sReturnCode);
END_CASE
END_IF
// Draw result image
hr := F_VN_PutTextExp(sResultText, ipImageRes, 50, 100, ETcVnFontType.TCVN_FT_HERSHEY_PLAIN, 4, aColorGreen, 3, TCVN_LT_4_CONNECTED, FALSE, S_OK);
hr := F_VN_TransformIntoDisplayableImage(ipImageRes, ipImageResDisp, hr);
Notice | |
Reserve hr Make sure not to use the variable hr between the call of the Code Reading function and the evaluation as a return value of another function. |
Results
If a code is found as expected, is hr = S_OK
and the result can be output.
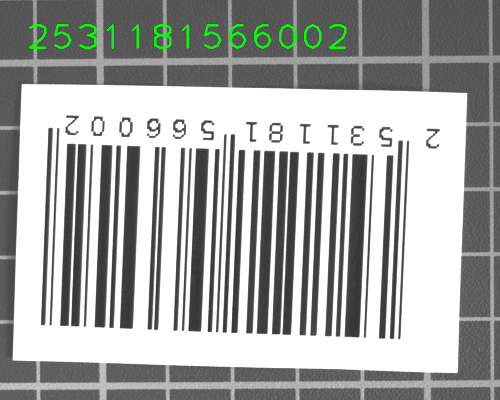
If the expected code cannot be found, the return code is S_FALSE
. If execution is aborted by a watchdog, the code is 16#256
. A corresponding message can be output.
If the function is not executed correctly, the error code can be output. This does not occur in the sample and is only caused by incorrect use of Code Reading functions.