EAN-13 Barcode Reading
This sample illustrates the following
- One EAN13 code is read per image using the function F_VN_ReadBarcodeExp
- The execution time of the function is monitored via a watchdog.
Explanation
- The functions F_VN_ReadBarcode / F_VN_ReadBarcodeExp can search and read barcodes of different types in the image. To speed up the processing time, the barcode type (
eBarcodeType
) can be specified. In this example, these areTCVN_BT_EAN13
. In addition, the processing time can be monitored via a watchdog. The sample Blob Detection with watchdog monitoring provides more comprehensive information on the watchdog. - Compared to F_VN_ReadBarcode, the function F_VN_ReadBarcodeExp, which is used in this example, has the following features:
- in addition to the barcode type, the search direction (
eSearchDirection
) can be specified:
- TCVN_BSD_ANY first searches in horizontal direction and then in vertical direction
- TCVN_BSD_HORIZONTAL only searches in horizontal direction
- TCVN_BSD_VERTICAL only searches in vertical direction - the code section in which the readout took place can be returned.
Variables
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDsply : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDsply : ITcVnDisplayableImage;
// Barcode
ipCodeDecodedList : ITcVnContainer;
ipCodeContourList : ITcVnContainer;
sCodeAsString : STRING(255);
eBarcodeSearchDirection : ETcVnBarcodeSearchDirection := TCVN_BSD_ANY;
eBarcodeType : ETcVnBarcodeType := TCVN_BT_EAN13;
// Watchdog
hrWD : HRESULT;
tStop : DINT := 50000;
tRest : DINT;
// Output
sText : STRING;
// Color
aColorRed : TcVnVector4_LREAL := [255, 0, 0];
Code
// Execute the Barcode Reading Function with EAN13 selected monitored by the Watchdog-Function
// ---------------------------------------------------------------
hrWD := F_VN_StartRelWatchdog(tStop, S_OK);
hr := F_VN_ReadBarcodeExp(
ipSrcImage := ipImageIn,
ipDecodedData := ipCodeDecodedList,
ipContours := ipCodeContourList,
eBarcodeType := eBarcodeType,
nCodeNumber := 1,
eSearchDirection := eBarcodeSearchDirection,
hrPrev := hr);
hrWD := F_VN_StopWatchdog(hrWD, tRest => tRest);
// Check if the function was executed successfully
IF hr = S_OK THEN
// Export Code into String
hr := F_VN_ExportSubContainer_String(ipCodeDecodedList, 0, sCodeAsString, 255, hr);
// Write Code into Result Image
hr := F_VN_PutTextExp(sCodeAsString, ipImageRes, 50, 100, ETcVnFontType.TCVN_FT_HERSHEY_PLAIN, 5, aColorRed,3, TCVN_LT_4_CONNECTED, FALSE, hr);
// Draw Code Contour into Result Image
hr := F_VN_DrawContours(ipCodeContourList, 0, ipImageRes, aColorRed, 3, hr);
ELSE
// Write HRESULT into Result Image
sText := CONCAT('Returncode ', DINT_TO_STRING(hr));
hr := F_VN_PutTextExp(sText, ipImageRes, 50, 100, ETcVnFontType.TCVN_FT_HERSHEY_PLAIN, 5, aColorRed,3, TCVN_LT_4_CONNECTED, FALSE, hr);
END_IF
// Write Code Reading proceeded time into Result Image
sText := CONCAT(CONCAT('Time: ', DINT_TO_STRING(tStop - tRest)), 'us');
hr := F_VN_PutTextExp(sText, ipImageRes, 50, 200, ETcVnFontType.TCVN_FT_HERSHEY_PLAIN, 5, aColorRed,3, TCVN_LT_4_CONNECTED, FALSE, hr);
Results
If TCVN_BSD_ANY is selected as the search direction, both horizontal and vertical EAN13 codes will be detected. When comparing the watchdog time, however, it becomes apparent that horizontal EAN13 codes (first image) are recognized much faster than vertical EAN13 codes (second image), since the code search initially takes place in horizontal direction.
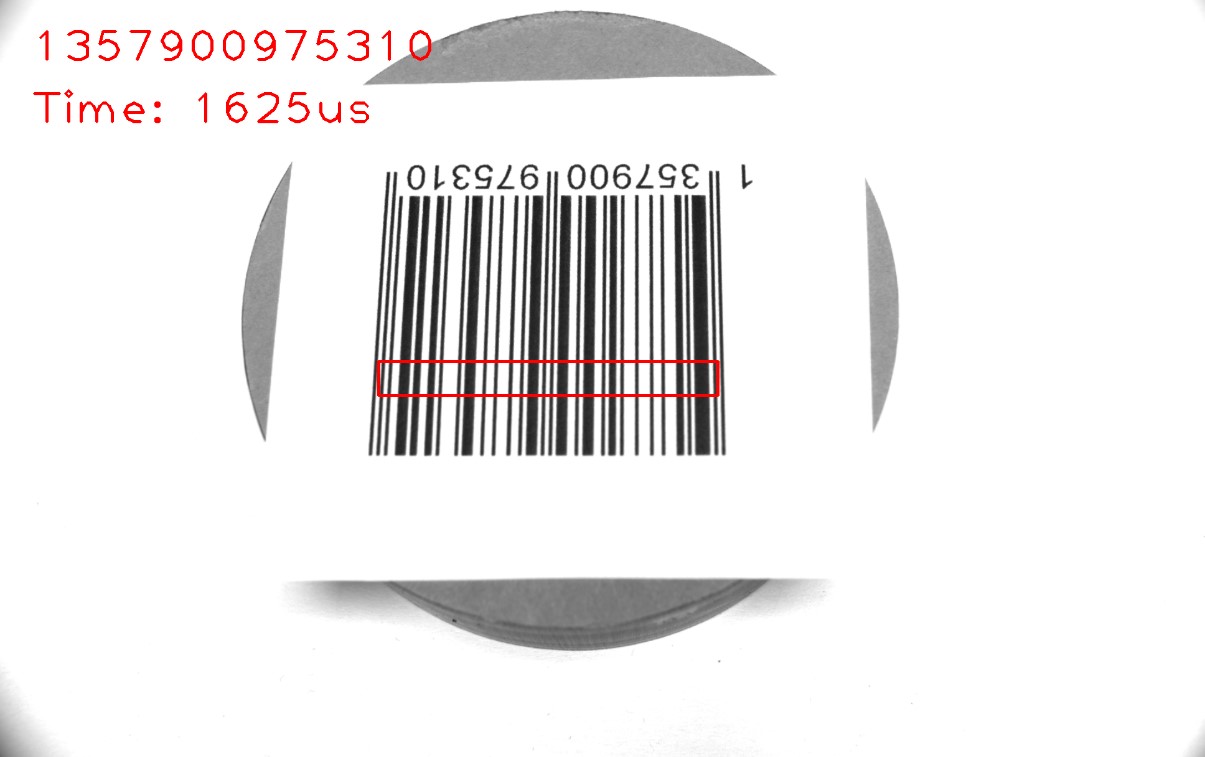
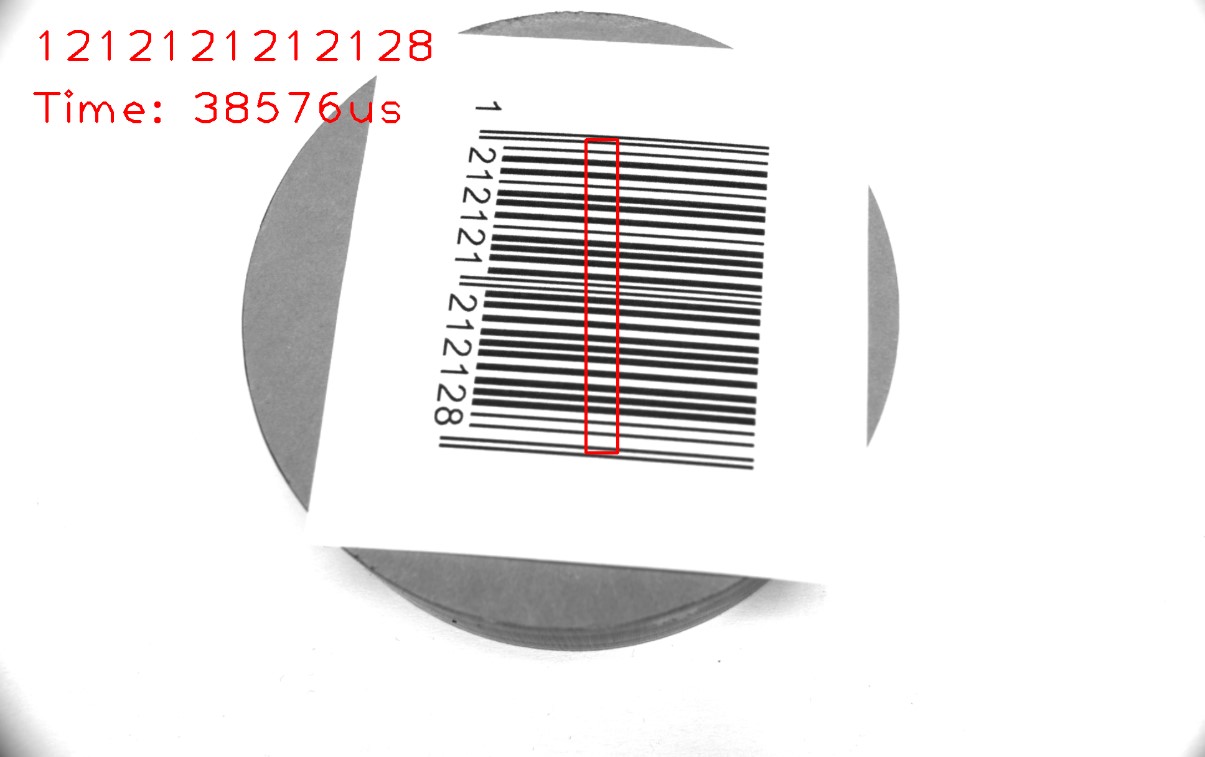
If the search direction is set to TCVN_BSD_VERTICAL, the vertical EAN13 code is recognized much faster (third image). However, in this case there is no search for horizontal codes.
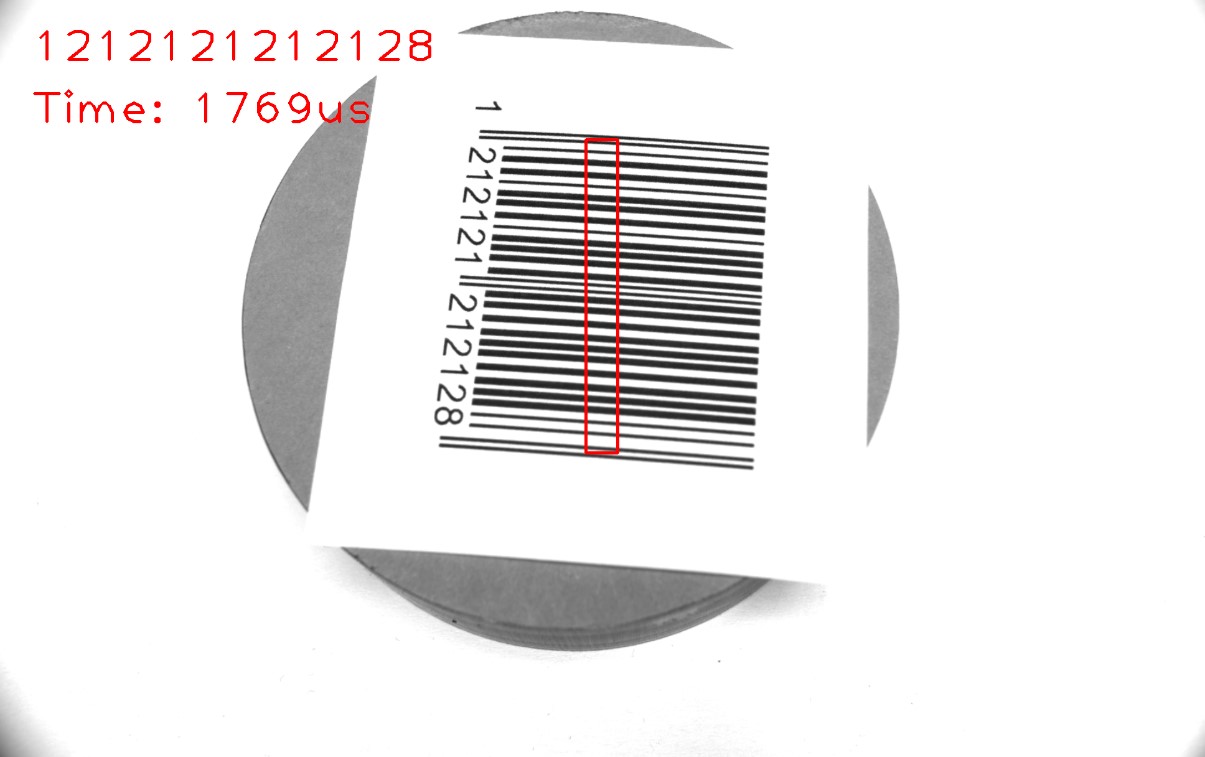