Locate Circular Arc
In this example, the function F_VN_LocateCircularArc is used to locate and measure circular arcs. This function returns a TcVnCircularArc structure containing the center, radius and bounding angles as result. To measure complete circles, use the function F_VN_LocateEllipse instead.
The start position of the search should correspond approximately to the expected circle center of the circular arc, which is 400, 300 in the corresponding sample images.
To demonstrate that an approximate position is sufficient here, the corresponding parameter aCenter in the sample was set to 420, 310. The sample illustrates that it is possible to compensate small positional changes of the objects to be examined in the image.
Since the actual radius in the sample images is about 200 pixels and the starting point is deliberately inaccurate, the search radius fRadius was set to 270. In the specific sample, around 230 pixels would have been sufficient, but in practice it is always good to have an additional safety buffer, e.g. if the position of the object deviates more than expected.
For the sample images, the search direction must be selected so that the objects are found correctly in all images. A good direction would be down left, around 2,356 rad (135°). To illustrate that this direction does not have to be exact, fDirection was set to 2.1 rad (120.3°) in the sample.
In addition, a transition from light to dark, starting from the starting point, should be specified as a search criterion. The minimum contrast also has to be specified, above which the function detects an intensity difference between adjacent pixels as an edge.
In case these parameters are not sufficient to achieve good results, the expert function F_VN_LocateCircularArcExp offers a number of additional parameters, which are not considered in this sample.
Variables
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// result
stArc : TcVnCircularArc;
// input parameters (to specify where to start searching for the circular arc)
aCenter : TcVnPoint2_REAL := [420, 310];
fRadius : REAL := 270;
fDirection : LREAL := 2.1;
// drawing
aColor : TcVnVector4_LREAL := [0, 175, 0];
sText : STRING(255);
Code
hr := F_VN_LocateCircularArc(
ipSrcImage := ipImageIn,
stCircularArc := stArc,
aCenterPoint := aCenter,
fSearchRadius := fRadius,
fArcDirectionRad := fDirection,
eEdgeDirection := TCVN_ED_LIGHT_TO_DARK,
fMinStrength := 100,
hrPrev := hr);
// Draw result for visualization
hr := F_VN_ConvertColorSpace(ipImageIn, ipImageRes, TCVN_CST_GRAY_TO_RGB, hr);
hr := F_VN_DrawCircularArc(stArc, ipImageRes, aColor, 2, hr);
sText := CONCAT(CONCAT(CONCAT('Center ', REAL_TO_STRING(stArc.aCenter[0])), ', '), REAL_TO_STRING(stArc.aCenter[1]));
hr := F_VN_PutText(sText, ipImageRes, 420, 25, TCVN_FT_HERSHEY_SIMPLEX, 0.7, aColor, hr);
sText := CONCAT('Radius ', REAL_TO_STRING(stArc.fRadius));
hr := F_VN_PutText(sText, ipImageRes, 420, 50, TCVN_FT_HERSHEY_SIMPLEX, 0.7, aColor, hr);
sText := CONCAT('Angle start ', REAL_TO_STRING(stArc.fStartAngle * 180 / LREAL_TO_REAL(PI)));
hr := F_VN_PutText(sText, ipImageRes, 420, 75, TCVN_FT_HERSHEY_SIMPLEX, 0.7, aColor, hr);
sText := CONCAT('Angle end ', REAL_TO_STRING(stArc.fEndAngle * 180 / LREAL_TO_REAL(PI)));
hr := F_VN_PutText(sText, ipImageRes, 420, 100, TCVN_FT_HERSHEY_SIMPLEX, 0.7, aColor, hr);
// Display source and result image
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, S_OK);
hr := F_VN_TransformIntoDisplayableImage(ipImageRes, ipImageResDisp, S_OK);
Results
For visualization, the circular arc that is found is drawn into the result image using the function F_VN_DrawCircularArc. The underlying numerical values are also displayed as text.
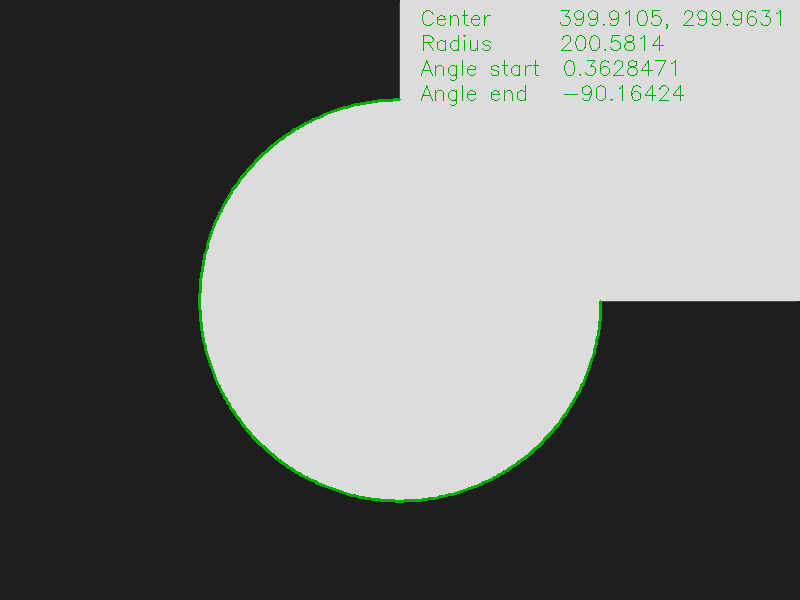
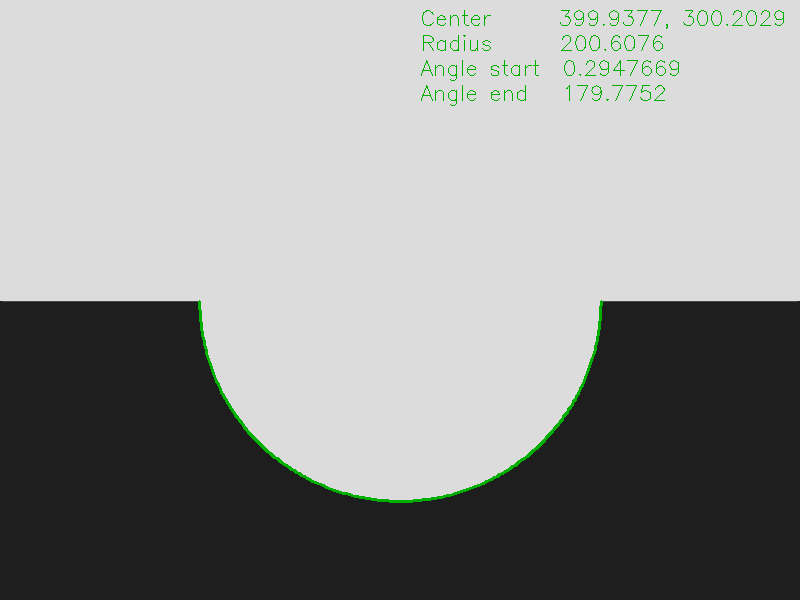
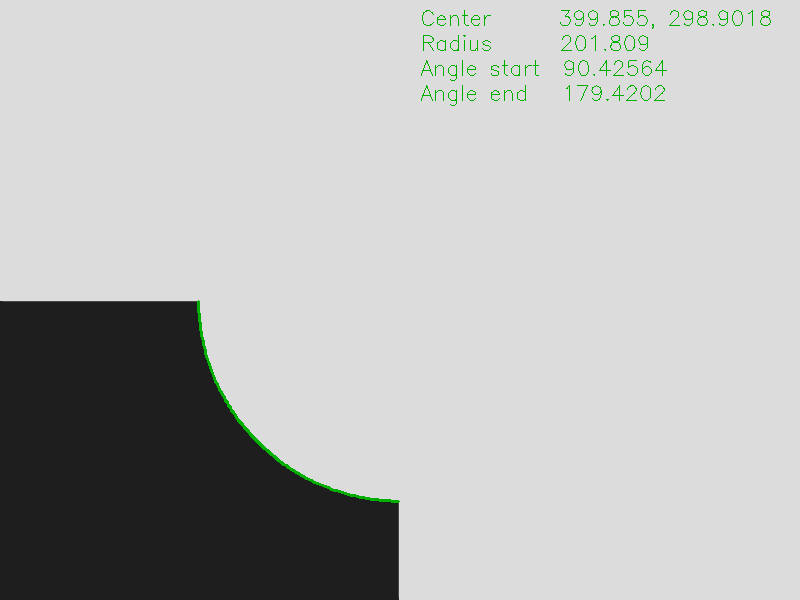