Locate Ellipse
In this sample,
- the function F_VN_LocateEllipseExp is used to locate a circular object in a defined search area
- and the execution time is monitored with a watchdog and limited if necessary.
Explanation
For a basic understanding of the measurement functions and their parameters, please follow the Locate Edge sample first. The function F_VN_LocateEllipseExp differs from F_VN_LocateEdgeExp in that it uses a circular search window instead of a rectangular one. In this sample a large, inaccurate search window is parameterized, since the position of the circular objects changes slightly. The circles can be determined with the same search window in all images.
The parameter bInvertSearchDirection
which was set to TRUE
in this sample, was used to define that the search direction is reversed, i.e. from the outside towards aCenter
. This is necessary because the sample images also contain edges within the ellipse the system is looking for.
The search direction was set to TCVN_ED_LIGHT_TO_DARK
, since in this sample the objects are darker than the background.
The parameter nMaxThickness
, which defines the number of pixels with which fMinStrength
must be reached, was set to "7
".
Variables
hr : HRESULT;
hrFunc : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// result
stEllipse : TcVnRotatedRectangle;
ipContourPoints : ITcVnContainer;
// input parameters
aCenter : TcVnPoint2_REAL := [650, 400];
fRadius : REAL := 300;
fMinRadius : REAL := 100;
fMinStrength : REAL := 30;
nSubPixIter : UDINT := 10;
nSearchLines : UDINT := 40;
eAlgorithm : ETcVnEdgeDetectionAlgorithm := TCVN_EDA_INTERPOLATION;
// Watchdog
hrWD : HRESULT;
tStop : DINT := 15000;
tRest : DINT;
nFraction : UDINT;
// drawing
aColorRed : TcVnVector4_LREAL := [200, 0, 0];
aColorGreen : TcVnVector4_LREAL := [0, 175, 0];
sText : STRING(255);
Code
hrWD := F_VN_StartRelWatchdog(tStop, hr);
hrFunc := F_VN_LocateEllipseExp(
ipSrcImage := ipImageIn,
stEllipse := stEllipse,
aCenter := aCenter,
fSearchRadius := fRadius,
eEdgeDirection := TCVN_ED_LIGHT_TO_DARK,
fMinStrength := fMinStrength,
nMaxThickness := 7,
bInvertSearchDirection := TRUE,
fMinSearchRadius := fMinRadius,
nSubpixelsIterations := nSubpixIter,
nSearchLines := nSearchLines,
fApproxPrecision := 0.0001,
eAlgorithm := eAlgorithm,
ipContourPoints := ipContourPoints,
hrPrev := hr);
hrWD := F_VN_StopWatchdog(hrWD, nFractionProcessed=>nFraction, tRest=>tRest);
// Draw result for visualization
hr := F_VN_ConvertColorSpace(ipImageIn, ipImageRes, TCVN_CST_GRAY_TO_RGB, hr);
sText := CONCAT(CONCAT('Processed ', UDINT_TO_STRING(nFraction)), '%');
hr := F_VN_PutTextExp(sText, ipImageRes, 25, 50, TCVN_FT_HERSHEY_SIMPLEX, 1.3, aColorGreen, 2, TCVN_LT_8_CONNECTED, FALSE, hr);
sText := CONCAT(CONCAT('Time ', DINT_TO_STRING(tStop - tRest)), 'us');
hr := F_VN_PutTextExp(sText, ipImageRes, 25, 100, TCVN_FT_HERSHEY_SIMPLEX, 1.3, aColorGreen, 2, TCVN_LT_8_CONNECTED, FALSE,hr);
hr := F_VN_DrawCircle(REAL_TO_UDINT(aCenter[0]), REAL_TO_UDINT(aCenter[1]), REAL_TO_UDINT(fMinRadius), ipImageRes, aColorRed, 2, hr);
hr := F_VN_DrawCircle(REAL_TO_UDINT(aCenter[0]), REAL_TO_UDINT(aCenter[1]), REAL_TO_UDINT(fRadius), ipImageRes, aColorRed, 2, hr);
hr := F_VN_DrawPoints(ipContourPoints, ipImageRes, TCVN_DS_X, aColorGreen, hr);
hr := F_VN_DrawPoint(REAL_TO_UDINT(stEllipse.aCenter[0]), REAL_TO_UDINT(stEllipse.aCenter[1]), ipImageRes, TCVN_DS_PLUS, aColorGreen, hr);
hr := F_VN_DrawEllipse(stEllipse, ipImageRes, aColorGreen, 1, hr);
// Display source and result image
hr := F_VN_TransformIntoDisplayableImage(ipImageIn, ipImageInDisp, S_OK);
hr := F_VN_TransformIntoDisplayableImage(ipImageRes, ipImageResDisp, S_OK);
Results
The function returns the TcVnRotatedRectangle
structure stEllipse
, which defines the ellipse that was found by its center aCenter
, its height and width stSize.fHeight
/ stSize.fWidth
and its angle fAngle
.
Optionally, one additional edge point per search line ipContourPoints
can be returned from which the ellipse was approximated.
For visualization, the search area is first drawn into the image in the form of two red circles around the specified center point. The ellipse that was found is drawn as a green line and the corresponding center as a green „+“. The individual measuring points from ipContourPoints
are drawn as green x. In addition, the processing percentage and the required computing time are shown at the top left. For the parameters used in this sample, the result for the input image LocateEllipse_T.bmp looks like this:
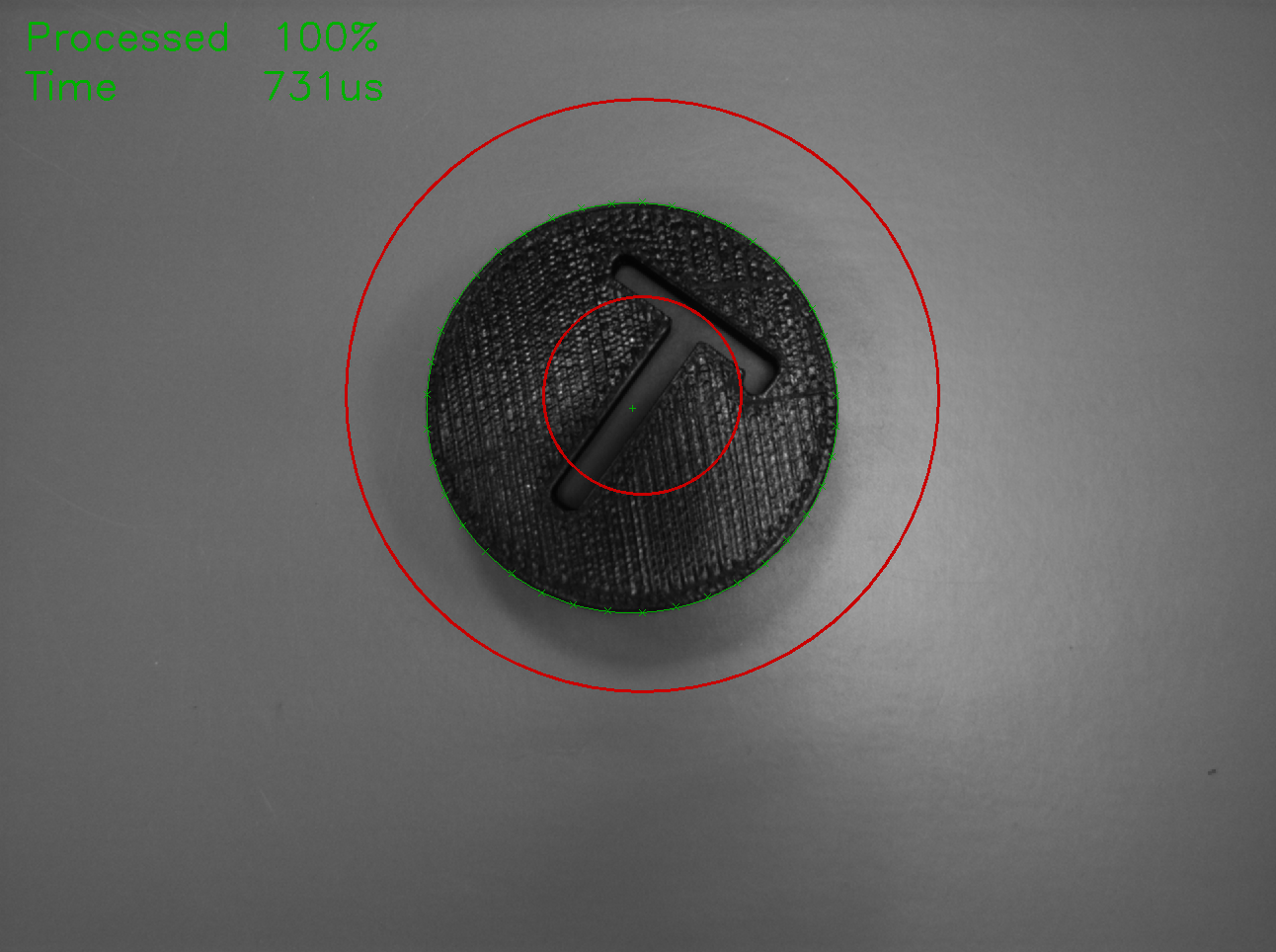
If the number of search lines is increased from 40 to 180, the required computing time increases linearly as expected:
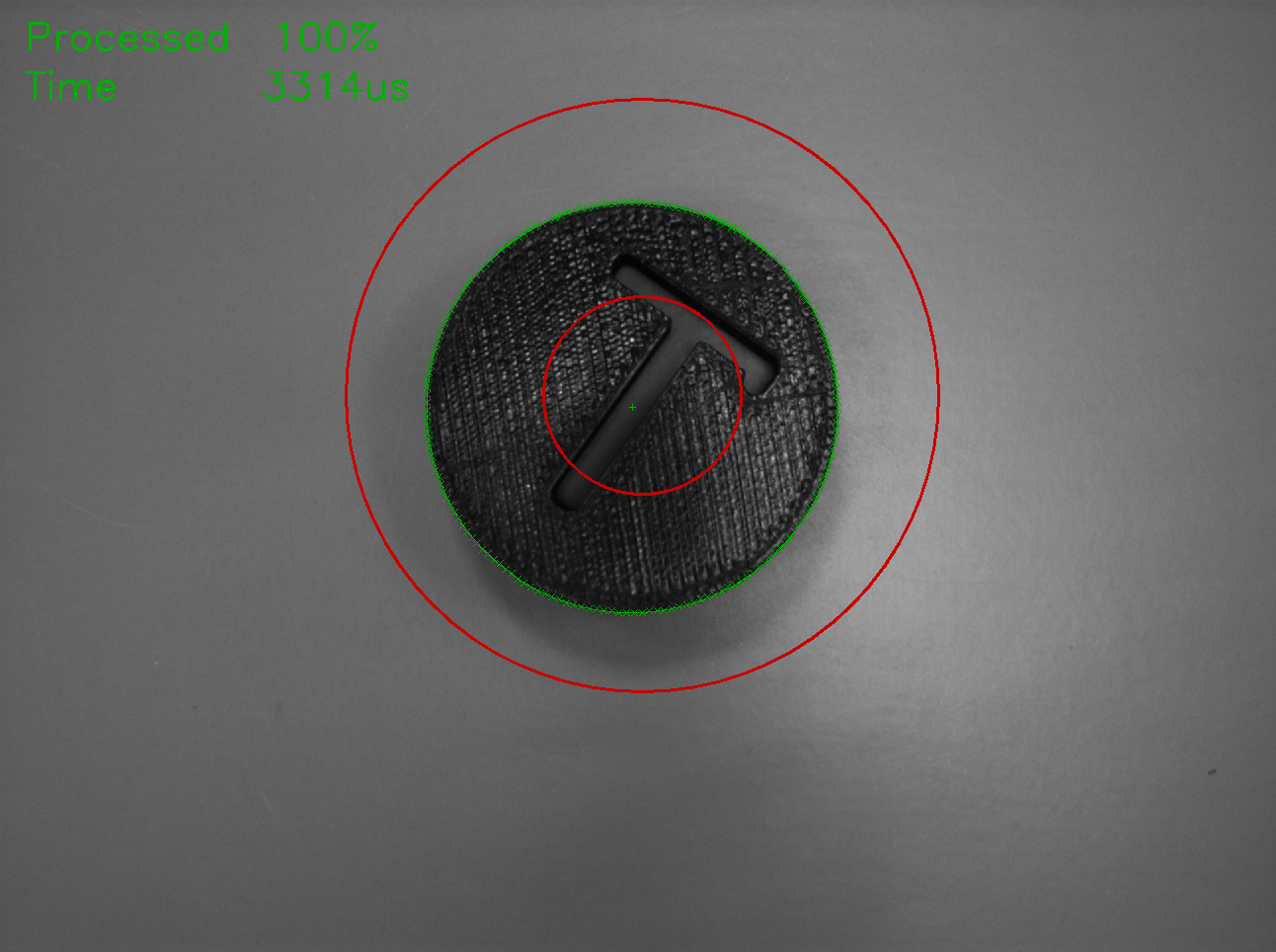
In practice the computing time varies somewhat, depending on the input image. In many cases it is therefore useful to limit the computing time by means of a watchdog. If, for example, the number of search lines is set to 120 and the watchdog timeout tStop
to 2000 (depending on the CPU used), the function is terminated at around 85% of the processing time, and the partial results available at this point are returned:
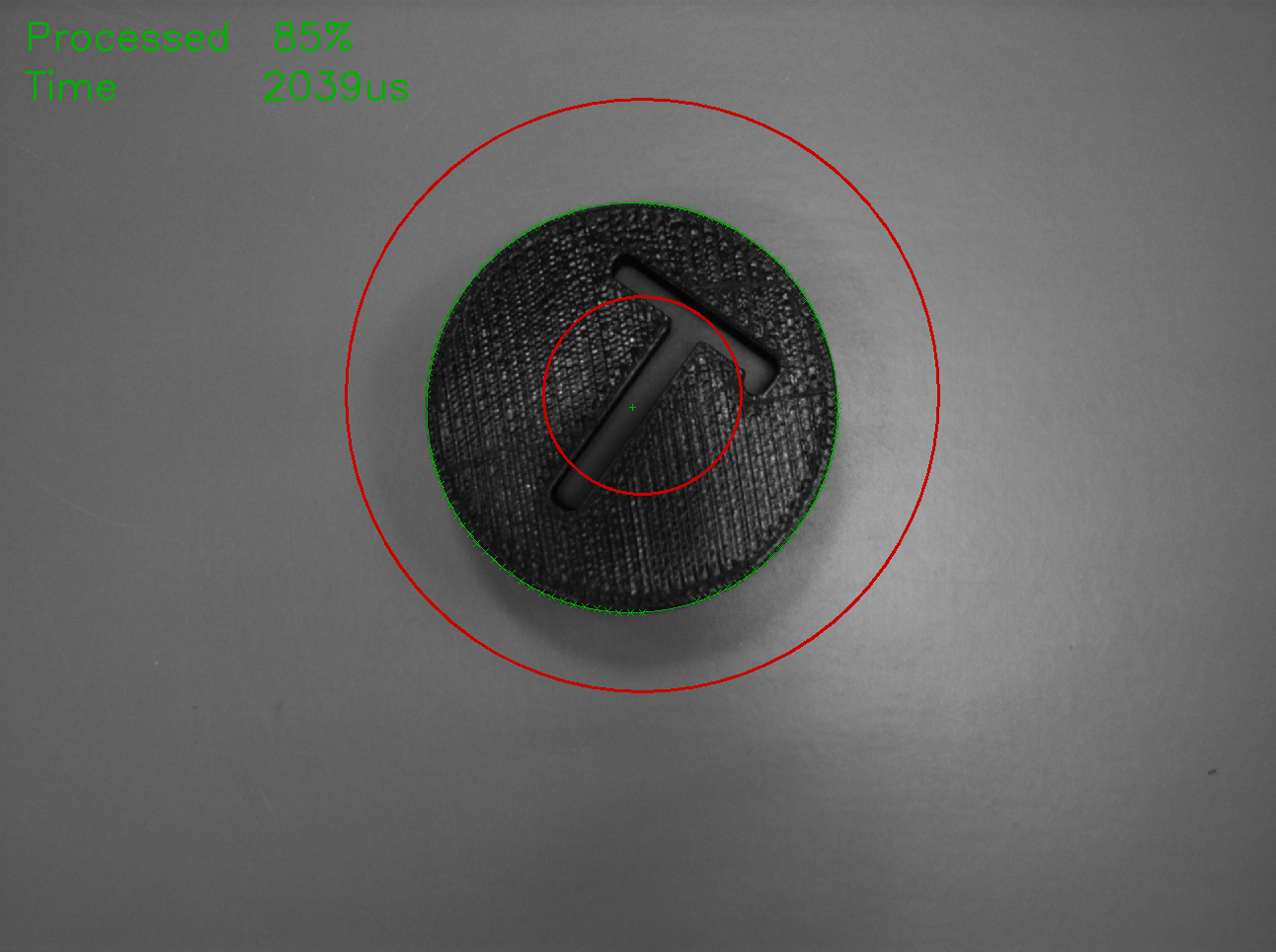
Since the algorithm can only be interrupted and the partial results returned at specific points, the function does not abort exactly after 2000 µs but takes a little longer, which must be taken into account when selecting the abort time. The maximum additional time required generally depends on the algorithm and the parameterization.
Although the results of some search lines are missing due to the termination (four relatively large gaps between the green x), the ellipse could still be correctly approximated, i.e. the partial results can be used as if the function had been processed 100%.
This is also possible to a limited extent if the position of an object is partly outside the defined search range, e.g. because a previous positioning step was incorrect. If, for example, fMinRadius
is set to 180, part of the outer contour of some sample images lies outside the specified range. Nevertheless, enough search points are available to approximate an ellipse. In practice, however, once should not rely on this and should set the search area large enough:
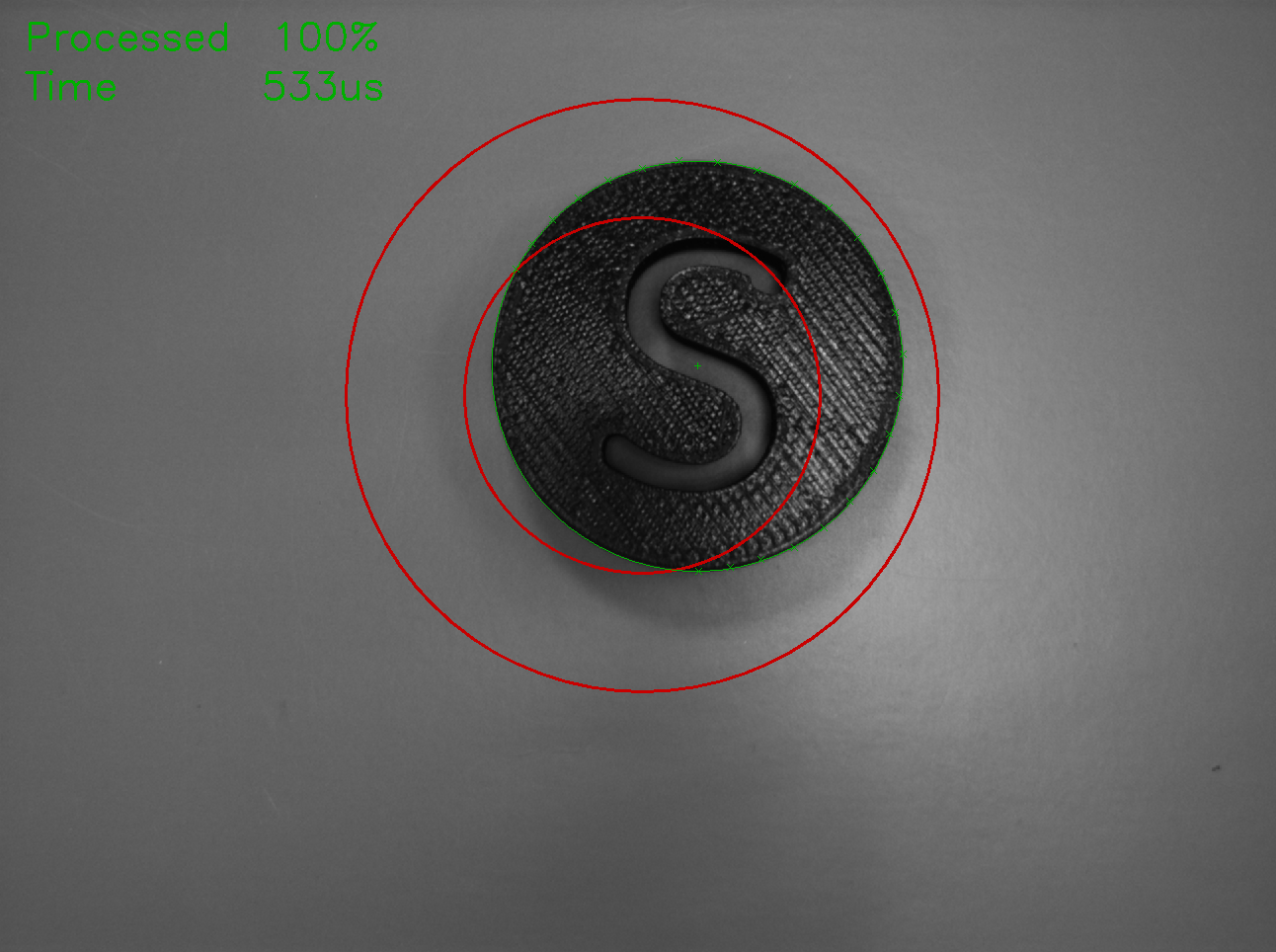