Find Contour - Hierarchy & Retrieval Mode
This sample shows the retrieval mode (ETcVnContourRetrievalMode) for the contour search (F_VN_FindContoursExp and F_VN_FindContourHierarchyExp) and how it affects the contour result and the returned hierarchy.
Explanation
The contour retrieval mode (eRetrievalMode of type ETcVnContourRetrievalMode) can be used to specify how the contour hierarchy is taken into account during the contour search. This sample visualizes the results according to the selected mode.
Detailed explanations of the hierarchy structure can be found in F_VN_FindContourHierarchyExp.
Variables
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
ipImageHierarchy : ITcVnImage;
ipImageHierarchyDisp : ITcVnDisplayableImage;
// Sample Specific Variables
ipContourList : ITcVnContainer;
ipContour : ITcVnContainer;
ipHierarchyList : ITcVnContainer;
aHierarchy : TcVnVector4_DINT;
aHierarchyTree : TcVnVector4_DINT;
eRetrievalMode : ETcVnContourRetrievalMode := TCVN_CRM_LIST;
eApproximationMethod : ETcVnContourApproximationMethod := TCVN_CAM_SIMPLE;
aOffset : TcVnPoint;
nNumOfElem : ULINT;
nParents : UINT;
i : ULINT;
j : ULINT;
stRect : TcVnRectangle_UDINT;
sText : STRING(80);
// Colors
aColorWhite : TcVnVector4_LREAL := [255, 255, 255];
aColorBlack : TcVnVector4_LREAL := [0, 0, 0];
aColorList : ARRAY[0..4] OF TcVnVector4_LREAL := [ [255,0,0], [0,255,0], [0,0,255], [200,200,0], [200,0,200]];
Code
// Create Result Image
hr := F_VN_ConvertColorSpace(ipImageIn, ipImageRes, TCVN_CST_Gray_TO_RGB, hr);
hr := F_VN_CreateImage(ipImageHierarchy, 130, 200, EtcVnElementType.TCVN_ET_USINT, 3, hr);
hr := F_VN_SetPixels(ipImageHierarchy, aColorWhite, hr);
// Find Contours and their Hierarchy
// -----------------------------------
hr := F_VN_FindContourHierarchyExp(
ipSrcImage := ipImageIn,
ipContours := ipContourList,
ipHierarchy := ipHierarchyList,
eRetrievalMode := eRetrievalMode,
eApproximationMethod := eApproximationMethod,
aOffset := aOffset,
hrPrev := hr);
hr := F_VN_GetNumberOfElements(ipContourList, nNumOfElem, hr);
IF nNumOfElem > 0 THEN
FOR i:= 0 TO (nNumOfElem-1) BY 1 DO
hr := F_VN_GetAt_ITcVnContainer(ipContourList, ipContour, i, hr);
hr := F_VN_GetAt_TcVnVector4_DINT(ipHierarchyList, aHierarchy, i, hr);
// Count Parents and set aColor depending on parent number
nParents := 0;
aHierarchyTree := aHierarchy;
WHILE SUCCEEDED(hr) AND_THEN aHierarchyTree[3] >= 0 DO
nParents := nParents + 1;
hr := F_VN_GetAt_TcVnVector4_DINT(ipHierarchyList, aHierarchyTree, aHierarchyTree[3], hr);
END_WHILE
// Draw contour and number into the result image
sText := TO_STRING(i);
hr := F_VN_UprightBoundingRectangle(ipContour, stRect, hr);
hr := F_VN_PutText(sText, ipImageRes, stRect.nX +10, stRect.nY +15, TCVN_FT_HERSHEY_PLAIN, 1, aColorList[(nParents) MOD 4], hr);
hr := F_VN_DrawContours(ipContour, -1, ipImageRes, aColorList[(nParents) MOD 4], 2, hr);
// Write Hierarchy
hr := F_VN_PutText(sText, ipImageHierarchy, 10, TO_UDINT(10 + i*10), TCVN_FT_HERSHEY_PLAIN, 0.5, aColorList[(nParents) MOD 4], hr);
FOR j := 0 TO 3 BY 1 DO
sText := TO_STRING(aHierarchy[j]);
hr := F_VN_PutText(sText, ipImageHierarchy, TO_UDINT(10 + (j+1)*22), TO_UDINT(10 + i*10), TCVN_FT_HERSHEY_PLAIN, 0.5, aColorBlack, hr);
END_FOR
END_FOR
END_IF
Results
The input image
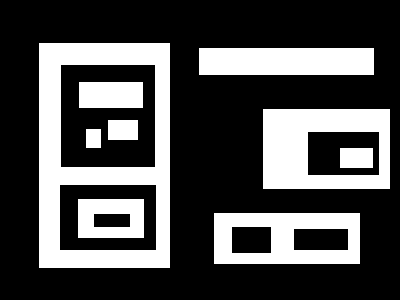
The contours found with TCVN_CRM_TREE. The colors render contours at the same level
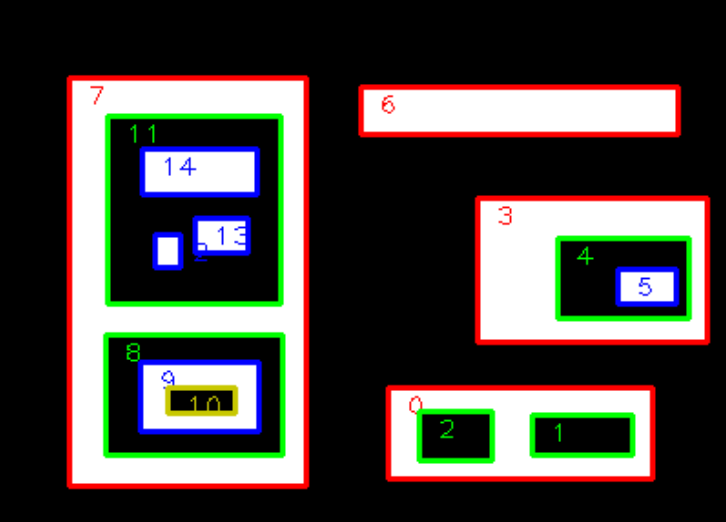
The hierarchy for the above result image. Each row refers to a contour. The columns have the following meaning:
- Contour index ("colored column“)
- Index of the next contour on the same hierarchical level
- Index of the previous contour on the same hierarchical level
- Index of the first child contour
- Index of parent contour
The number -1 indicates that no corresponding contour exists.
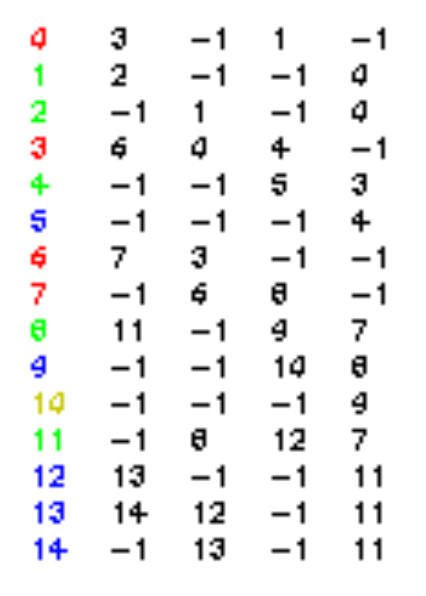