Find contour instead of Blob Detection
This sample shows an alternative solution to the Blob Detection sample using F_VN_FindContoursExp.
Explanation
The function F_VN_DetectBlobs consolidates many individual image processing steps in one function call. However, the internal processing steps can also be carried out by individual function calls. This can make it easier to set parameters because one can see the intermediate results. Therefore, in this sample the same images are used as in the Blob Detection sample, and the same round structures are searched for.
Variables
hr : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageWork : ITcVnImage;
ipImageThresholdDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// Sample Specific Variables
ipContourList : ITcVnContainer;
ipContourResultList : ITcVnContainer;
ipContour : ITcVnContainer;
ipIterator : ITcVnForwardIterator;
aOffset : TcVnPoint;
fThreshold : LREAL := 70;
fArea : LREAL;
fAreaMin : LREAL := 100;
fAreaMax : LREAL := 100000;
fCircularity : LREAL;
fCircularityMin : LREAL := 0.8;
// Image Infos
stPixelFormat : TcVnPixelFormat;
// COLORS
aColorGreen : TcVnVector4_LREAL := [0, 255, 0];
Code
Preprocessing for image segmentation
// Image Segementation
hr := F_VN_Threshold(ipImageIn, ipImageWork, fThreshold, 255, TCVN_TT_Binary, hr);
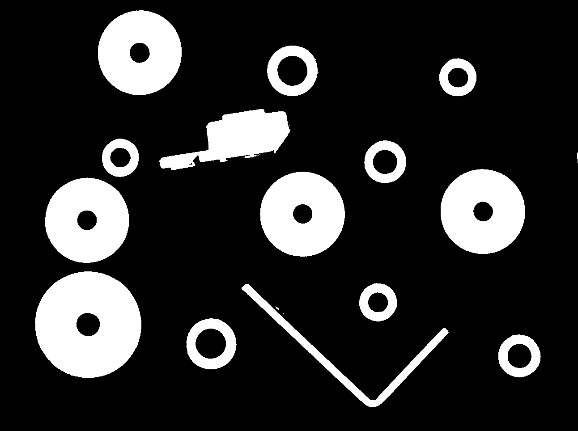
Contour search
// Find Contours
hr := F_VN_FindContoursExp(
ipSrcImage := ipImageWork,
ipContours := ipContourList,
eRetrievalMode := ETcVnContourRetrievalmode.TCVN_CRM_LIST,
eApproximationMethod := ETcVnContourApproximationMethod.TCVN_CAM_SIMPLE,
aOffset := aOffset,
hrPrev := hr);
Filtering of contours according to the surrounding area in pixels and circularity
// Filter Contours
hr := F_VN_GetForwardIterator(ipContourList, ipIterator, hr);
hr := F_VN_CreateContainer(ipContourResultList, ContainerType_Vector_Vector_TcVnPoint2_DINT, 0, hr);
WHILE SUCCEEDED(hr) AND_THEN ipIterator.CheckIfEnd() <> S_OK DO
hr := F_VN_GetContainer(ipIterator, ipContour, hr);
hr := F_VN_IncrementIterator(ipIterator, hr);
// Filter by Area
hr := F_VN_ContourArea(ipContour, fArea, hr);
IF fArea > fAreaMin AND fArea < fAreaMax THEN
// Filter by Circularity
hr := F_VN_ContourCircularity(ipContour, fCircularity, hr);
IF fCircularity > fCircularityMin THEN
// Add contour to the result contour container
hr := F_VN_AppendToContainer_ITcVnContainer(ipContour, ipContourResultList, hr);
END_IF
END_IF
END_WHILE
Result output
// Draw contours into the result image and display it
hr := F_VN_DrawContours(ipContourResultList, -1, ipImageRes, aColorGreen, 3, hr);
hr := F_VN_TransformIntoDisplayableImage(ipImageRes, ipImageResDisp, hr);
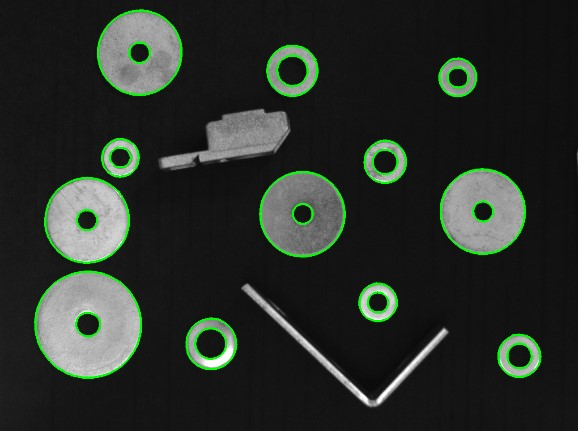