Blob Detection with watchdog monitoring
This sample illustrates the following
- The function F_VN_DetectBlobs is used to find round structures in images.
- The execution time of the function is monitored via a watchdog.
Explanation
The F_VN_DetectBlobs function facilitates the search for similar structures in the image. It combines setting threshold values with searching and filtering for contours in a single call. In this sample, the function is parameterized to return all round structures in the example images.
Due to external influences, the actual image content can deviate greatly from the expected image content. With image processing algorithms whose execution time depends on the image content, this can lead to cycle time overruns and thus to undesired side effects. To prevent this there is a watchdog. The watchdog can abort functions marked with the sentence: "Can return partial results when canceled by Watchdog" in the API after a specified period of time. The results obtained up to this point can be used further.
F_VN_DetectBlobs is one the functions that can be monitored in this way. By changing the permissible execution time tStop
in the watchdog start function F_VN_StartRelWatchdog, a function termination followed by application of partial results can be observed and compared to full execution of F_VN_DetectBlobs. The watchdog can be stopped via F_VN_StopWatchdog. A positive return value in tRest
specifies the remaining time, while a negative return value in tRest
specifies the additional time required for termination.
Task configuration
Watchdog monitoring must be enabled in the executing PLC task. Otherwise the watchdog function calls in the PLC are ignored and the start watchdog function returns the return value 16#71A: "No Interface".
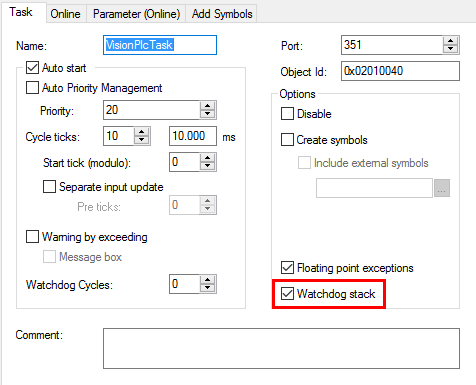
Variables
hr : HRESULT;
hrFunc : HRESULT;
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// Blob Detection
stBlobParams : TcVnParamsBlobDetection;
ipContourList : ITcVnContainer;
// Watchdog
hrWD : HRESULT;
tStop : DINT := 5000;
tRest : DINT;
nFraction : UDINT;
// Output
sText : STRING(255);
// Color
aColorGreen : TcVnVector4_LREAL := [0, 255, 0, 0];
// Image Infos
stPixelFormat : TcVnPixelFormat;
Code
// Set Parameters
// --------------------------------------------------------------
// - Set fMaxArea to 100000 detect all circles or set it to 10000
// to detect only the small circles
stBlobParams.bFilterByArea := TRUE;
stBlobParams.fMinArea := 100;
stBlobParams.fMaxArea := 100000;
stBlobParams.bFilterByCircularity := TRUE;
stBlobParams.fMinCircularity := 0.80;
stBlobParams.fMinThreshold := 70;
stBlobParams.fThresholdStep := 0;
// Execute DetectBlobs-Function monitored by Watchdog-Function
// --------------------------------------------------------------
// - Set the Watchdog stop time tStop from 5000us to 1000us to see
// a Watchdog interrupt and that the interim results can be used
hrWD := F_VN_StartRelWatchdog(tStop, S_OK);
hrFunc := F_VN_DetectBlobs(
ipSrcImage := ipImageIn,
ipBlobContours := ipContourList,
stParams := stBlobParams,
hrPrev := hr);
hrWD := F_VN_StopWatchdog(hrWD, tRest => tRest, nFractionProcessed => nFraction);
// Draw Result Image
// --------------------------------------------------------------
hr := F_VN_DrawContours(ipContourList, -1, ipImageRes, aColorGreen, 3, hr);
sText := CONCAT(CONCAT('Processed ', UDINT_TO_STRING(nFraction)), '%');
hr := F_VN_PutTextExp(sText, ipImageRes, 25, 50, TCVN_FT_HERSHEY_SIMPLEX, 1.3, aColorGreen, 2, TCVN_LT_8_CONNECTED, FALSE, hr);
sText := CONCAT(CONCAT('Time ', DINT_TO_STRING(tStop - tRest)), 'us');
hr := F_VN_PutTextExp(sText, ipImageRes, 25, 100, TCVN_FT_HERSHEY_SIMPLEX, 1.3, aColorGreen, 2, TCVN_LT_8_CONNECTED, FALSE,hr);
sText := CONCAT('Returncode ', DINT_TO_STRING(hrFunc));
hr := F_VN_PutTextExp(sText, ipImageRes, 25, 150, TCVN_FT_HERSHEY_SIMPLEX, 1.3, aColorGreen, 2, TCVN_LT_8_CONNECTED, FALSE,hr);
Results
The input image
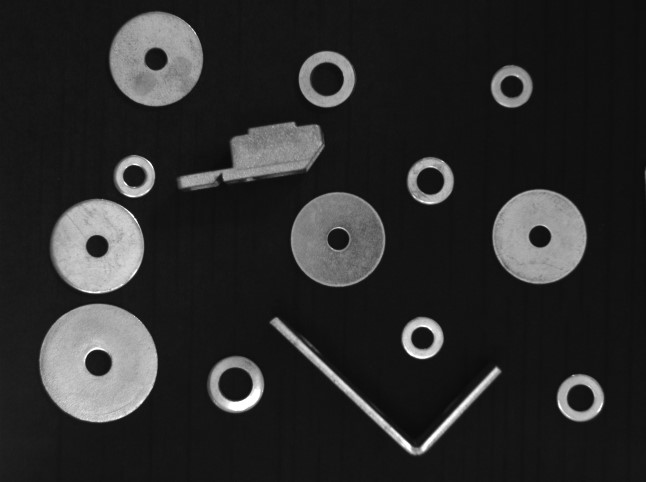
All circles were found in the image, with the parameterization of the sample code.
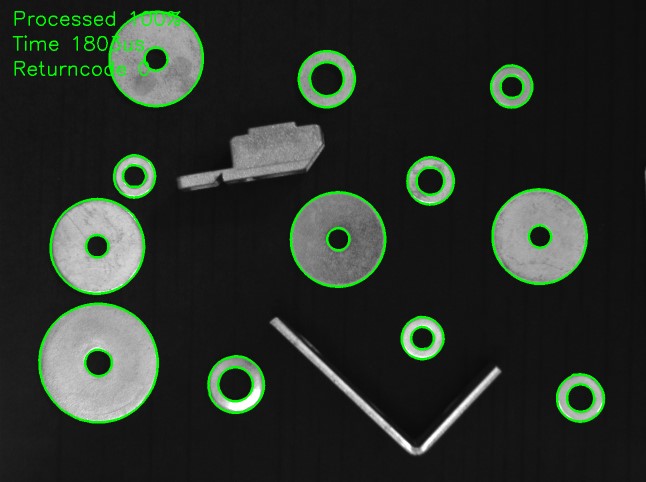
Some of the circles were found in the image, by triggering the watchdog after 1 ms. Since the abort process takes some time, the actual time required is greater than 1 ms.
hrWD := F_VN_StartRelWatchdog(tStop:=1000, WATCHDOG_ACCUMULATION_TYPE_MEAN, S_OK);
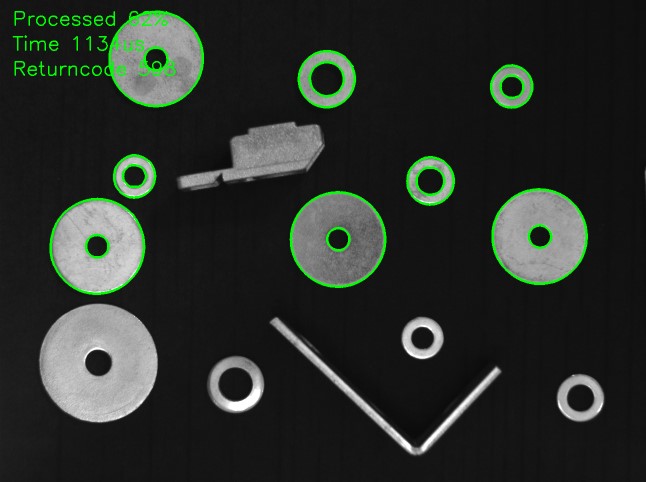
Only the small circles were found in the image by reducing the maximum enclosing area in pixels.
stBlobParams.fMinArea := 100;
stBlobParams.fMaxArea := 10000;
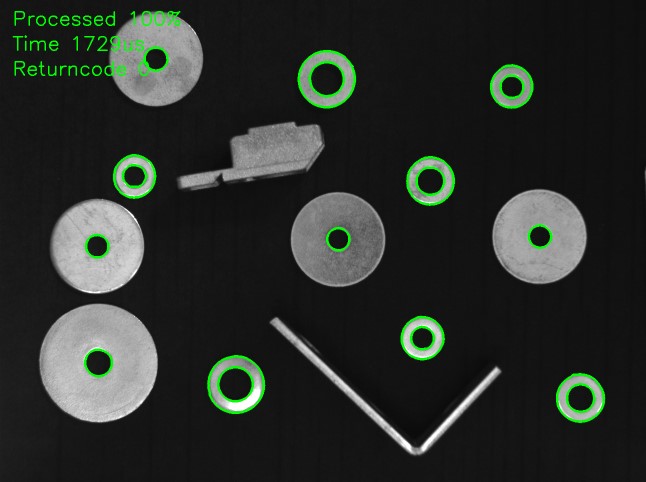