Help Functions
The following help functions are used as default behavior by certain controls or to ensure interoperability with PLC data types.
BoxColorConverter
The function BoxColorConverter
is listed in the category Vision
. It is used as the default value for the BoxColorConversion
attribute of the Color Control. It converts between the RGB and the color format specified by the Format
parameter.
Parameter | Data type | Description |
---|---|---|
|
| Is used internally in the HMI to receive asynchronous returns. Can be set to |
|
| The color value to be converted. |
|
| Direction of the conversion:
|
|
| Format for conversion:
|
|
| Scaling of the color values: |
As the function works asynchronously, it returns the converted color as a future value, i.e. as Promise<ColorValue | null>
. See ConvertColor
for handling Promise. Errors during conversion result in null
being returned.
ConvertColor
The function ConvertColor
is listed in the category Vision
. It converts a color array between the formats Gray, RGB, RGBa, HSV, HSV FULL, and HLS, HLS FULL, Lab, YUV and YCrCb.
Parameter | Data type | Description |
---|---|---|
|
| Is used internally in the HMI to receive asynchronous returns. Can be set to |
|
| The color value to be converted. |
|
| Format of the incoming color value:
|
|
| Format to which is converted:
|
|
| Specifies the number of decimal places to round to. |
|
| Scaling of the incoming color values:
|
|
| Scaling of the converted color values:
|
Errors during conversion result in zero being returned. As the function is asynchronous, it returns the converted color as a future value, i.e. as Promise<ColorValue | null>
.
For example, to convert the color red in RGB format to HLS format, the following function call can be used:
let rgb = [255, 0, 0];
let promise = TcHmi.Functions.Beckhoff.Vision.ConvertColor(null, rgb, "RGB", "HLS", "2 Decimals", "8-Bit (USINT)", "8-Bit (USINT)");
promise.then(hls => console.log(hls.toString()));
//console output
//0,127.5,255
//console output with DestPixelType "16-Bit (UINT)"
//0,32640,65280
In order to be able to process the future ColorValue
, this can be waited for with await
. Alternatively, as shown in this sample, the function then
can be used to pass an anonymous function, e.g. hls => console.log(hls.toString())
. This receives the ColorValue
after the conversion has been completed. The ColorValue
is then output as string
in the console.
PixelColorFormatter
The function PixelColorFormatter
is listed in the category Formatting
. It is the default value for the PixelColorFormat
property of the Image Watch Control. It converts the PixelColor
value into a string that can be displayed via HTML. Specifically, the color values of the individual channels are appended to each other and also highlighted in color for certain color formats:
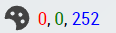
Parameter | Data type | Description |
---|---|---|
|
| Is used internally in the HMI to receive asynchronous returns. Can be set to |
|
| The color value to be converted. |
|
| Format for conversion:
|
|
| Specifies the number of decimal places to round to. With "Unrounded" there is no rounding. |
|
| Scaling of the color values:
|
The function returns a String
coded as HTML as Promise<string>
. See ConvertColor
for handling Promise.
ShapeValueFormatter
The function ShapeValueFormatter
is listed in the category Formatting
. It is the default value for the ShapeValueFormat
property of the Image Watch Control. It converts the ShapeValue
value into a string that can be displayed via HTML. For example, for a rectangle, the area is calculated in pixels and returned as a string:
Parameter | Data type | Description |
---|---|---|
|
| The shape data to be converted. |
| Type of the shape passed. |
The function returns an HTML coded String
.
ToRotatedRectangle
The ToRotatedRectangle
function is listed in the Data Conversion category. It converts a UprightRectangle
to a RotatedRectangle
with angle 0
.
Parameter | Data type | Description |
---|---|---|
| The rectangle to be converted. |
The function returns the converted rectangle as TcVnRotatedRectangle and can be applied as follows:
let upright = {
nWidth: 100,
nHeight: 60,
nX: 200,
nY: 100,
}
let rotated = TcHmi.Functions.Beckhoff.Vision.ToRotatedRectangle(upright);
console.log(rotated);
//console output
// {
// aCenter: [250, 130],
// fAngle: 0,
// stSize: { fWidth: 100, fHeight: 60 },
// }
ToUprightRectangle
The ToUprightRectangle
function is listed in the Data Conversion category. It converts a RotatedRectangle
to a UprightRectangle
, discarding the angle.
Parameter | Data type | Description |
---|---|---|
| The rectangle to be converted. |
The function returns the converted rectangle as TcVnRectangle and can be applied as follows:
let rotated = {
aCenter: [250, 130],
fAngle: 0,
stSize: { fWidth: 100, fHeight: 60 },
}
let upright = TcHmi.Functions.Beckhoff.Vision.ToUprightRectangle(rotated);
console.log(upright);
//console output
// {
// nWidth: 100,
// nHeight: 60,
// nX: 200,
// nY: 100,
// }