FB_ALY_TimeBasedTeachPath_1Ch
Time Based Teach Path 1Ch periodically writes the input data to a file according to the configured number of teach operations. This means that the values are not written sequentially for each period, but the values of a new period are compared with the existing values. The period can be defined by the input values Start Period and Stop Period (boolean signals are required). According to the teach mode, each value is overwritten or retained, so that the result is a taught input signal that can later be used as a reference signal for the Time Based Envelope 1Ch algorithm, for example.
Syntax
Definition:
FUNCTION_BLOCK FB_TimeBasedTeachPath_1Ch
VAR_OUTPUT
ipResultMessage: Tc3_EventLogger.I_TcMessage;
bError: BOOL;
bNewResult: BOOL;
bConfigured: BOOL;
bBusy: BOOL;
bTeaching: BOOL;
eState: E_TeachState;
nWrittenValues: ULINT;
nValuesInFile: ULINT;
nCurrentTeachCycles: ULINT;
END_VAR
Outputs
Name | Type | Description |
---|---|---|
ipResultMessage | Contains more detailed information on the current return value. This special interface pointer is internally secured so that it is always valid/assigned. | |
bError | BOOL | This output is |
bNewResult | BOOL | When a new result has been calculated, the output is |
bConfigured | BOOL | Displays |
bBusy | BOOL |
|
bTeaching | BOOL |
|
eState | Current state of the function block. See state diagram. | |
nWrittenValues | ULIINT | Total number of values written during the teach-in process. Not to be confused with the number of values in File, which are overwritten in each teach-in cycle. |
nValuesInFile | ULINT | Number of values currently being written to the file. |
nCurrentTeachCycles | ULINT | Number of teach-in cycles in the file. |
Methods
Name | Definition Location | Description |
---|---|---|
Call() | Local | Method calculates the outputs for a given configuration. |
Configure() | Local | General configuration of the algorithm with its parameterized conditions. |
GetBusyState() | Local | This method provide the busy state of the function block. |
Reset() | Local | Resets all internal states or the calculations made so far. |
SetChannelValue() | Local | Method to pass values to the algorithm. |
SwitchState_Idle() | Local | Method to set the function block into an idle state if the teach procedure is finished. |
SwitchState_Teach() | Local | Method to set the function block into the teach mode. |
UpdateState() | Local | Method to use for the transaction from one state to another state. |
State diagram
Due to the asynchronous file access in real time applications this function block needs a state machine to prepare and finish file access.
At startup the function block is in Idle state. To write the incoming data to a file it has to be switched to state Teach. Therefore the method SwitchState_Teach() has to be called once to set the function block to the PendingTeach state. After that the method UpdateState() has to be called until the function block is in state Teach. In this state one or multiple teach cycles can be proceeded. If the function block should not teach additional cycles it can be set to Idle state again. To initiate the state switch the method SwitchState_Idle() has to be called. After that the method UpdateState() has to be called until the function block is in state Idle.
State diagram for the teach procedure of the data:
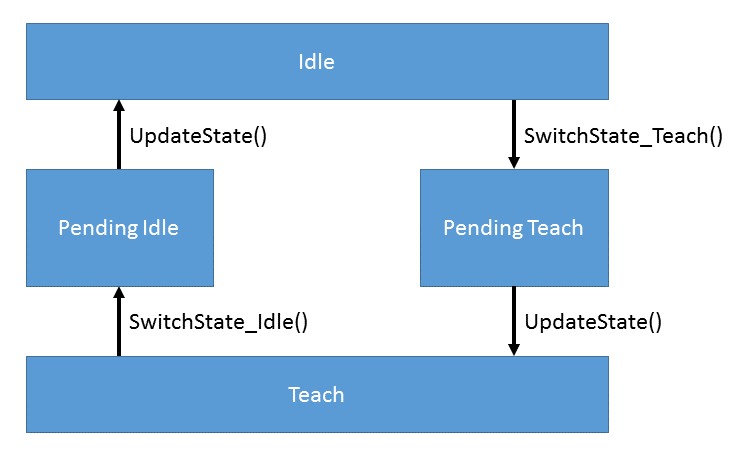
Sample
VAR
fbTimeBasedTeach : FB_ALY_TimeBasedTeachPath_1Ch;
eTeachMode : E_ALY_TeachMode := E_ALY_TeachMode.Mean;
nSegmentSize : UDINT := 200;
tTimeout : TIME := T#5S;
nSetNumTeaches : UDINT := 10;
bInvolveExistingFile : BOOL := TRUE;
sFilePath : STRING := 'C:\TwinCAT\3.1\Boot\TimeBasedTeach.tas';
bNegateStartPeriod : BOOL := FALSE;
bNegateStopPeriod : BOOL := FALSE;
bConfigure : BOOL := TRUE;
eState : E_ALY_TeachState := E_ALY_TeachState.Idle;
bTeach : BOOL;
fInput : LREAL;
bStartPeriod : BOOL;
bStopPeriod : BOOL;
END_VAR
// Configure algorithm
IF bConfigure THEN
bConfigure := FALSE;
fbTimeBasedTeach.Configure(eTeachMode, nSegmentSize, tTimeout, nSetNumTeaches, bInvolveExistingFile, sFilePath, bNegateStartPeriod, bNegateStopPeriod);
END_IF
// Call algorithm
eState := fbTimeBasedTeach.eState;
CASE eState OF
E_ALY_TeachState.Idle:
IF bTeach THEN
fbTimeBasedTeach.SwitchState_Teach();
fbTimeBasedTeach.UpdateState();
END_IF
E_ALY_TeachState.Teach:
fbTimeBasedTeach.SetChannelValue(fInput);
fbTimeBasedTeach.Call(bStartPeriod := bStartPeriod, bStopPeriod := bStopPeriod);
IF NOT bTeach THEN
fbTimeBasedTeach.SwitchState_Idle();
fbTimeBasedTeach.UpdateState();
END_IF
E_ALY_TeachState.Pending,
E_ALY_TeachState.PendingIdle,
E_ALY_TeachState.PendingTeach:
fbTimeBasedTeach.UpdateState();
eState := fbTimeBasedTeach.eState;
END_CASE
Requirements
Development environment | Target platform | Plc libraries to include |
---|---|---|
TwinCAT v3.1.4024.0 | PC or CX (x64, x86) | Tc3_Analytics |