FB_ALY_LinearRegressionFitting
The Linear Regression Fitting function block approximates one variable (the Dependent input) by linear combination of several other variables (Input 01 ... Input 0n). This is done by the incremental stochastic gradient method. At the end of the analysis, the calculated coefficients are written to a file.
The linear combination is given by the following equation:
In each cycle, the values for to
are recalculated using the following rule:
This corresponds to the minimization of the squared deviation of the calculated values y (output by the function block as result) from the corresponding input value Dependent. The parameter corresponds to the step size and specifies how strongly the parameters are adjusted. The larger the value, the faster the coefficients approach a local optimum. However, if the value is too large, the algorithm may not converge.
Typically, the Linear Regression Fitting function block is first used to fit the weights for the regression of a target variable. Then, using the Linear Regression Inference function block and the fitted weights, the target variable can be predicted based on the input variables.
Syntax
Definition:
FUNCTION_BLOCK FB_LinearRegressionFitting
VAR_OUTPUT
ipResultMessage: Tc3_EventLogger.I_TcMessage;
bError: BOOL;
bNewResult: BOOL;
bConfigured: BOOL;
fResult: LREAL;
fMSE: LREAL;
bBusy: BOOL;
eState: E_TeachState;
END_VAR
Outputs
Name | Type | Description |
---|---|---|
ipResultMessage | Contains more detailed information on the current return value. This special interface pointer is internally secured so that it is always valid/assigned. | |
bError | BOOL | This output is |
bNewResult | BOOL | When a new result has been calculated, the output is |
bConfigured | BOOL | Displays |
fResult | LREAL | Outputs the approximated value for the inputs of the current cycle with the coefficients updated from them. |
fMSE | LREAL | Specifies the MSE (mean squared error) between the calculated Result value and the Dependent input value. |
bBusy | BOOL |
|
eState | Current state of the function block. See state diagram. |
Methods
Name | Definition location | Description |
---|---|---|
Call() | Local | Method for calculating the outputs for a specific configuration. |
Configure() | Local | General configuration of the algorithm with its parameterized conditions. |
FB_init() | Local | Initializes the number of input channels. |
GetBusyState() | Local | This method returns the Busy state of the function block. |
GetChannelOutputValue() | Local | Method for receiving values from different output channels. |
Reset() | Local | Resets all internal states or the calculations performed so far. |
SetChannelValue() | Local | Method for passing values to the algorithm. |
SwitchState_Idle() | Local | Method for setting the function block to Idle state when the teach-in process is complete. |
SwitchState_Teach() | Local | Method for putting the function block into teach-in mode. |
UpdateState() | Local | Method to be used for the transition from one state to another state. |
State diagram
Due to the asynchronous file access in real time applications this function block needs a state machine to prepare and finish file access.
At startup the function block is in Idle state. To write the incoming data to a file it has to be switched to state Teach. Therefore the method SwitchState_Teach() has to be called once to set the function block to the PendingTeach state. After that the method UpdateState() has to be called until the function block is in state Teach. In this state one or multiple teach cycles can be proceeded. If the function block should not teach additional cycles it can be set to Idle state again. To initiate the state switch the method SwitchState_Idle() has to be called. After that the method UpdateState() has to be called until the function block is in state Idle.
State diagram for the teach procedure of the data:
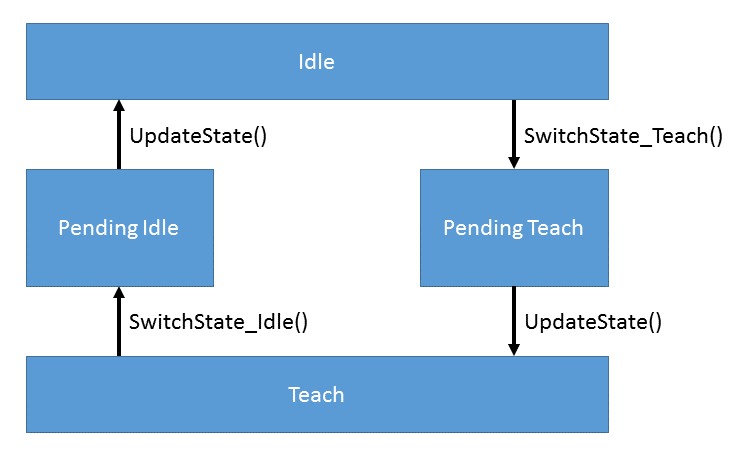
Sample
VAR
fbLinearRegressionFitting : FB_ALY_LinearRegressionFitting(nNumChannels := 1);
bBias : BOOL := TRUE;
fStepSize : LREAL := 0.01;
nMiniBatchSize : UDINT := 1;
tTimeout : TIME := T#5S;
bInvolveExistingFile : BOOL := TRUE;
sFilePath : STRING := 'C:\TwinCAT\3.1\Boot\LinearRegressionFitting.tas';
bConfigure : BOOL := TRUE;
eState : E_ALY_TeachState := E_ALY_TeachState.Idle;
bTeach : BOOL;
bFit : BOOL := TRUE;
fInputX : LREAL;
fInputY : LREAL;
fOut0 : LREAL;
fOut1 : LREAL;
END_VAR
// Configure algorithm
IF bConfigure THEN
bConfigure := FALSE;
fbLinearRegressionFitting.Configure(bBias, fStepSize, nMiniBatchSize, tTimeout, bInvolveExistingFile, sFilePath);
END_IF
// Call algorithm
eState := fbLinearRegressionFitting.eState;
CASE eState OF
E_ALY_TeachState.Idle:
IF bTeach THEN
fbLinearRegressionFitting.SwitchState_Teach();
fbLinearRegressionFitting.UpdateState();
END_IF
E_ALY_TeachState.Teach:
fbLinearRegressionFitting.SetChannelValue(1, fInputX);
fbLinearRegressionFitting.Call(bFit := bFit, dependent := fInputY);
fbLinearRegressionFitting.GetChannelOutputValue(0, fOut0);
fbLinearRegressionFitting.GetChannelOutputValue(1, fOut1);
IF NOT bTeach THEN
fbLinearRegressionFitting.SwitchState_Idle();
fbLinearRegressionFitting.UpdateState();
END_IF
E_ALY_TeachState.Pending,
E_ALY_TeachState.PendingIdle,
E_ALY_TeachState.PendingTeach:
fbLinearRegressionFitting.UpdateState();
eState := fbLinearRegressionFitting.eState;
END_CASE
Requirements
Development environment | Target platform | Plc libraries to include |
---|---|---|
TwinCAT v3.1.4024.0 | PC or CX (x64, x86) | Tc3_Analytics |