Example
This example offers an introduction to the handling of the function blocks that are available with the TcPlcMDP library.
This example is dedicated to the goal of determining the state of the Compact Flash card in the Embedded PC.
This can be found out via a parameter in the MDP Model. The querying of other parameters takes place analogously to this example.
Hence, this example can also be a guide to querying any MDP parameter from an MDP module.
If you wish to go through the example on a PC that does not use a silicon Compact Flash card as memory, you can query the CPU utilization instead, for example. To do this, execute this example in the same way, adapting just a few points accordingly. Necessary values for this can be found in the general module description of the MDP CPU module.
Overview
The following steps are now performed:
1. Installation of the PLC library
2. Program structure
3. Determination of the dynamic Module ID
4. Querying of the MDP parameter
5. Test
1. Installation of the PLC library
Start TwinCAT PLC Control.
Create a new PLC project with 'File > New'.
Select your target platform PC and CX (x86) or CX (ARM).
Your first POU is a program called MAIN and in the programming language ST (Structured Text).
Open the Resources tab and the library manager.
Insert the library TcMDP.lib as shown in the picture below via 'Insert > Further library'.
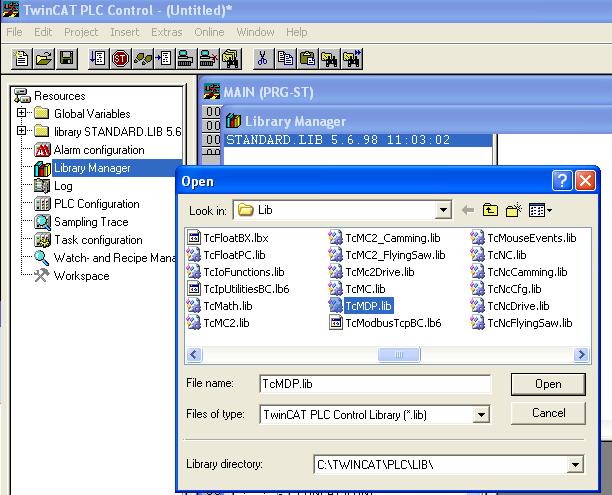
All PLC blocks of the TwinCAT PLC MDP library are now available to you. All further implicitly required libraries have been automatically integrated with the TcMDP.lib.
2. Program structure
The state of the Compact Flash card is represented by a parameter in the MDP. To query this individual parameter, the dynamic Module ID of the module in which the parameter is located must be known.
This dynamic Module ID must be determined using the function block FB_MDP_ScanModules.
The parameters can then be queried with the function block FB_MDP_Read.
Generate a state machine in the MAIN program for this sequence.
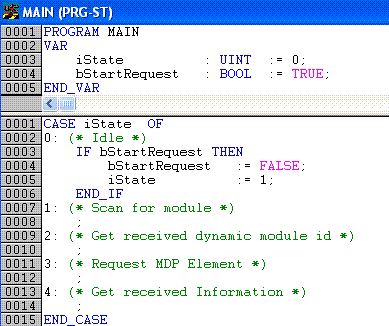
3. Determination of the dynamic Module ID
Insert the MDP function block FB_MDP_ScanModules (press F2).
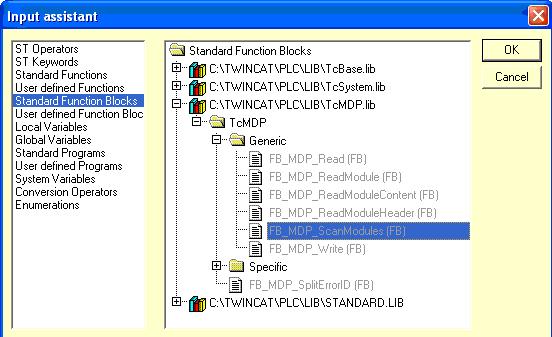
In state 1, start the function block by setting the input bExecute to TRUE.
The enumeration value eMDP_ModT_SiliconDrive, which allows accesses to information from Compact Flash cards, is specified at nModuleType. Further information on this module: General information on the MDP SiliconDrive moduleThere does no need to be any input on iModIdx. This way you can call the first found module of the selected module type automatically (default: iModIdx := 0).
Likewise, there does no need to be any input on tTimeout; instead, you can work with the default (DEFAULT_ADS_TIMEOUT).
1: (* Scan for module *)
FB_MDP_ScanModules(
bExecute:= TRUE,
nModuleType:= eMDP_ModT_SiliconDrive,
iModIdx:= ,
tTimeout:= ,
bBusy=> ,
bError=> ,
nErrID=> ,
nDynModuleId=> ,
iModuleTypeCount=> ,
iModuleCount=>
);
In state 2, call this function block cyclically by setting the input bExecute to FALSE. In this state, the function block is called if it is busy with the processing of the query.
The transition to the next state can be accomplished as soon as the output bBusy goes FALSE.
Your program should now look as follows:
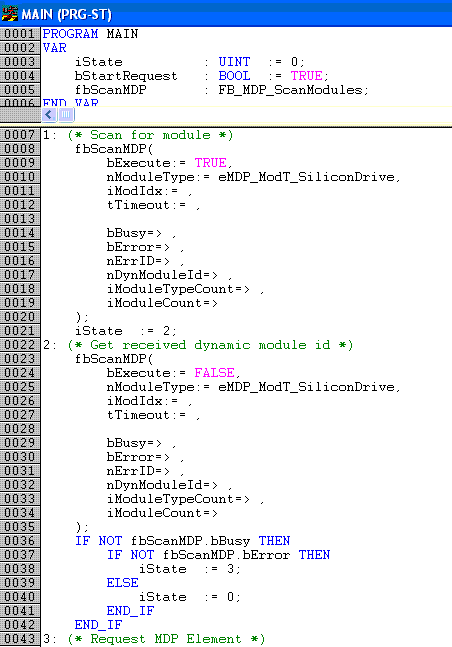
4. Querying of the MDP parameter
The MDP parameter that you would like to query is in a certain table.
This is in turn located in a certain module, which belongs to an area.
Take these values from the MDP description:
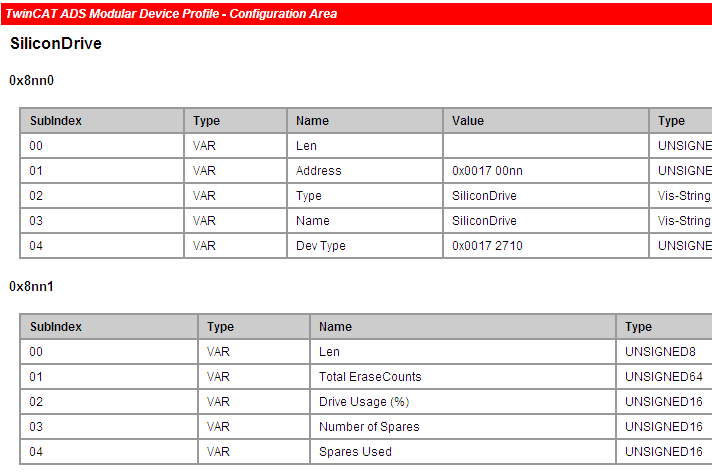
Excerpt from the general information on the MDP SiliconDrive module.
In order to query an MDP element, declare an instance of the function block FB_MDP_Read.
Likewise define a variable iDriveUsage, whereby this is an Unsigned16 variable.
fbReadMDP : FB_MDP_Read;
iDriveUsage : UINT;
Transfer the determined values for the sought MDP parameter to the function block. To do this, select the input variable stMDP_DynAddr of the type ST_MDP_Addr of the function block and assign the values.
3: (* Request MDP Element *)
fbReadMDP.stMDP_DynAddr.nArea := eMDP_Area_ConfigArea;
fbReadMDP.stMDP_DynAddr.nModuleId := fbScanMDP.nDynModuleId;
fbReadMDP.stMDP_DynAddr.nTableId := 1;
fbReadMDP.stMDP_DynAddr.nSubIdx := 2;
Further, in state 3, call the function block and start it by setting the input bExecute to TRUE.
You have already explicitly assigned the input stMDP_DynAddr.
As a data buffer, enter the address and length of your variable iDriveUsage for pDstBuf and cbDstBufLen.
As with the above function block, there does not need to be any input on tTimeout; instead, you can work with the default (DEFAULT_ADS_TIMEOUT).
The program section should now look as follows:
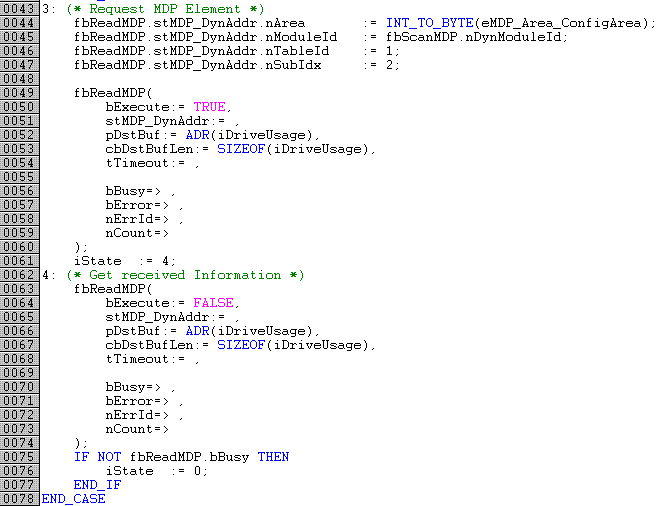
5. Test
Compile the created PLC program. Make sure that TwinCAT is in the Run mode on the desired system.
Login to the desired run-time system from TwinCAT PLC Control. Start the PLC program.
Upon the initialisation of bStartRequest with TRUE (see 2. Program structure) all conditions of the state machine are implemented once immediately at the program start.
If executed without error, the queried value is now stored in your variable iDriveUsage. In this example, this value indicates the percentage of the statistically possible number of writing cycles already performed by the Compact Flash card and thus provides useful information on the service life of your CF card.
If you have performed this example with the goal of querying the CPU utilization, then the utilization of the CPU in % will now be in your variable.
To start the complete query again, set your variable bStartRequest to TRUE again (for example by Online Write).
This example can also serve as a general guide. Each MDP parameter can be queried from an MDP module in the same way.
Click here to save this example program:
TcPlcMDP_Sample.zip.
Requirements
Development environment | Target platform | PLC libraries to be linked |
---|---|---|
TwinCAT v2.11.0 Build >= 1541 | PC or CX (x86, ARM) | TcMDP.Lib |