FB_FormatString2
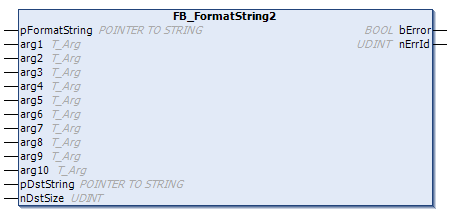
This function block can be used for converting up to 10 arguments (similar to fprintf) into a string and formatting them according to the format specification (e.g. '%+20.5f' or 'Measure X: %+.10d, Y: %+.10d'). The formatting takes place in the same PLC cycle. This means that the output string is available immediately after calling the function block.
As opposed to the function block FB_FormatString, the size of the format string and the output string is not limited to 255 characters. However, each argument is limited to a representation of 250 characters at the most. The function block returns an error if the number of characters is exceeded or if the output string is too small for the formatted character string.
Inputs
VAR_INPUT
pFormatString : POINTER TO STRING;
arg1 : T_Arg;
arg2 : T_Arg;
arg3 : T_Arg;
arg4 : T_Arg;
arg5 : T_Arg;
arg6 : T_Arg;
arg7 : T_Arg;
arg8 : T_Arg;
arg9 : T_Arg;
arg10 : T_Arg;
pDstString : POINTER TO STRING;
nDstSize : UDINT;
END_VAR
Name | Type | Description |
---|---|---|
pFormatString | STRING | Pointer to the format specification as a string. The address must be assigned each time the function block is called. The operator ADR() can be used for the assignment. |
arg1 to arg10 | Arguments to be formatted. The following helper functions can be used to convert PLC variables of different types into the required data type T_Arg: F_BYTE, F_WORD, F_DWORD, F_LWORD, F_SINT, F_INT, F_DINT, F_LINT, F_USINT, F_UINT, F_UDINT, F_ULINT, F_STRING, F_REAL, F_LREAL. | |
pDstString | STRING | Pointer to the resulting STRING variable. If successful, the formatted character string will be written here. The address must be assigned each time the function block is called. The operator ADR() can be used for the assignment. |
nDstSize | UDINT | Size of the resulting STRING variable in bytes. The operator SIZEOF() can be used for the assignment. |
Outputs
VAR_OUTPUT
bError : BOOL;
nErrId : UDINT;
END_VAR
Name | Type | Description |
---|---|---|
bError | BOOL | TRUE if an error occurred during the formatting. |
nErrId | UDINT | Returns the Format error code if the bError output is set. |
Example
PROGRAM MAIN
VAR
fbFormat : FB_FormatString2;
sFormat : STRING := 'Measure X: %+.10d, Y: %+.10d';
iY : DINT;
iX : DINT;
bError : BOOL;
nErrID : UDINT;
sOut : STRING(600);
END_VAR
iX := iX + 1;
iY := iY + 1;
fbFormat( pFormatString := ADR(sFormat), arg1 := F_DINT( iX ), arg2 := F_DINT( iY ), pDstString := ADR(sOut), nDstSize := SIZEOF(sOut), bError => bError, nErrID => nErrID );
Result:
sOut = 'Measure X: +0000000130, Y: +0000000130'
Requirements
Development environment | Target platform | PLC libraries to be integrated (category group) |
---|---|---|
TwinCAT v3.1.4022 | PC or CX (x86, x64, Arm®) | Tc2_Utilities (System) >=3.3.35.0 |