KL6301 with CX5120
This example shows how to write a simple PLC program for EIB in TwinCAT and how to link it with the hardware. The task is to change the state of a switching output with a button.
Example: EIB_Sample_KL6301_CX5120.zip
![]() | The TwinCAT project is available for download as *.zip file. This must first be unpacked locally so that the archive (*.tnzip file) is available for import into the TwinCAT project. |
Hardware
Setting up the components
- 1x CX5120 Embedded PC
- 1x KL1104 four-channel digital input terminal (for the Set/Reset function)
- 1x KL6301 EIB terminal
- 1x KL9010 end terminal
Set up the hardware and the EIB components as described in the associated documentation.
This example assumes that a Set button was connected to the first KL1104 input and a Reset button to the second, and that the EIB group address of the switching output is known.
Software
Creation of the PLC program
Create a new "TwinCAT XAE Project" and create a "Standard PLC Project".
Add the Tc2_EIB library to the PLC project at "References".
Create a global variable list with the name GVL_EIB and create the following variables:
VAR_GLOBAL
bSet AT %I* : BOOL;
bReset AT %I* : BOOL
arrKL6301_IN AT %I* : ARRAY[1..24] OF BYTE;
arrKL6301_OUT AT %Q* : ARRAY[1..24] OF BYTE;
stDataRec : EIB_REC;
END_VAR
Name | Type | Description |
---|---|---|
bSet | BOOL | Input variable for the Set button. |
bReset | BOOL | Input variable for the Reset button. |
arrKL6301_IN | BYTE | Input variable for the EIB terminal. |
arrKL6301_OUT | BYTE | Output variable for the EIB terminal. |
stDataRec | Structure for the communication with EIB. |
All EIB function blocks must be called in the same task.
In the MAIN program (ST), call the function block KL6301 and create the following variables.
PROGRAM MAIN
VAR
fbKL6301 : KL6301;
iStep : INT;
END_VAR
In the program part, the KL6301 is initially assigned a physical address and the filter for receiving EIB telegrams is configured. For more information see KL6301.
CASE iStep OF
0: // Initialising of the physical EIB address; has to be unique in the network
fbKL6301.EIB_PHYS_ADDR.Area := 2; //EIB Address 2.3.4
fbKL6301.EIB_PHYS_ADDR.Line := 3;
fbKL6301.EIB_PHYS_ADDR.Device := 4;
// Configuration of filters for the group addresses
// Filter 1 1/1/0 LEN 63
fbKL6301.EIB_GROUP_FILTER[1].GROUP_ADDR.MAIN:=1;
fbKL6301.EIB_GROUP_FILTER[1].GROUP_ADDR.SUB_MAIN:=1;
fbKL6301.EIB_GROUP_FILTER[1].GROUP_ADDR.NUMBER:=0;
fbKL6301.EIB_GROUP_FILTER[1].GROUP_LEN:=63;
// Filter 2 1/2/0 LEN 63
fbKL6301.EIB_GROUP_FILTER[2].GROUP_ADDR.MAIN:=1;
fbKL6301.EIB_GROUP_FILTER[2].GROUP_ADDR.SUB_MAIN:=2;
fbKL6301.EIB_GROUP_FILTER[2].GROUP_ADDR.NUMBER:=0;
fbKL6301.EIB_GROUP_FILTER[2].GROUP_LEN:=63;
//Filter 3 1/4/0 LEN 63
fbKL6301.EIB_GROUP_FILTER[3].GROUP_ADDR.MAIN:=1;
fbKL6301.EIB_GROUP_FILTER[3].GROUP_ADDR.SUB_MAIN:=4;
fbKL6301.EIB_GROUP_FILTER[3].GROUP_ADDR.NUMBER:=0;
fbKL6301.EIB_GROUP_FILTER[3].GROUP_LEN:=63;
//Filter 4 3/1/0 LEN 63
fbKL6301.EIB_GROUP_FILTER[4].GROUP_ADDR.MAIN:=3;
fbKL6301.EIB_GROUP_FILTER[4].GROUP_ADDR.SUB_MAIN:=1;
fbKL6301.EIB_GROUP_FILTER[4].GROUP_ADDR.NUMBER:=0;
fbKL6301.EIB_GROUP_FILTER[4].GROUP_LEN:=63;
// bActivate: activates the configuration of the KL6301 and enables the further data exchange
fbKL6301.bActivate :=TRUE;
IF fbKL6301.bReady THEN
IF NOT fbKL6301.bError THEN
iStep:=1; // EIB functionblock is now ready to send and receive
ELSE
iStep:=100; // EIB ERROR
END_IF
END_IF
1: ; // EIB functionblock is now ready to send and receive
100: ; // optional error handling EIB ERROR
END_CASE
//Call communication function block
fbKL6301(
iMode:= 0, // 4 filter with 64 entries each
KL6301_IN:= GVL_EIB.arrKL6301_in,
KL6301_OUT:= GVL_EIB.arrKL6301_out,
str_Data_Rec => GVL_EIB.stDataRec);
//Call programs for reading and sending
EIB_Send_Prg();
EIB_Rec_Prg();
Create an EIB program called EIB_Send_Prg() (CFC), in which the function block EIB_BIT_SEND is called.
Link the local variable bData with the global variables bSet and bReset, then with the input bData of the send block. Link the global variable stDataRec with st_Rec.

Create an EIB program called EIB_Rec_Prg() (CFC), in which the function block EIB_BIT_REC is called. In the sample, the pressing of a button is read by the function block. Link the global variable stDataRec with st_Rec.

Go to the task configuration and give the task a lower cycle time of 5 ms, for example.
Further conditions can be found in the description of the function block KL6301.
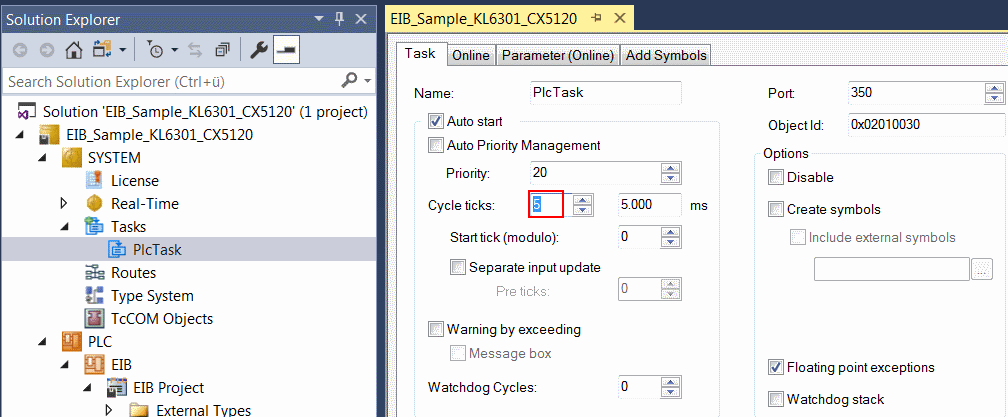
I/O configuration
Select the CX as target system and initiate a search for its hardware. In the project instance within the PLC section, you can see that the input and output variables are assigned to the task.
Now link the global variables with the inputs and outputs of the bus terminals.
The linking of the EIB variables is described in detail below.
Right-click the array arrKL6301_in and select "Change Link".
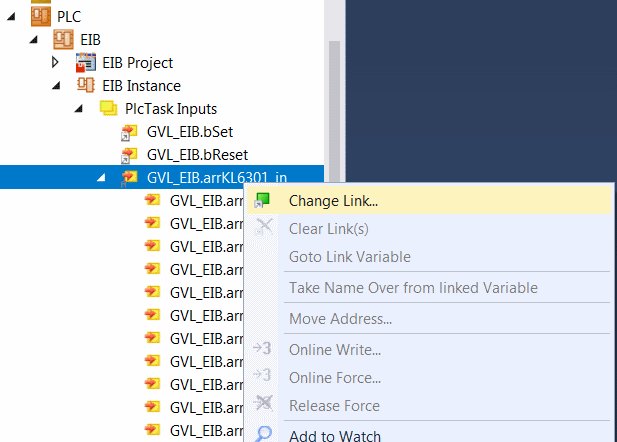
Under “I/O Configuration” select the terminal, select “All Types” and “Continuous”, then select “ParameterStatus”, “InputData1” to “InputData22” with the left mouse button and the >SHIFT< key. Then click "OK".
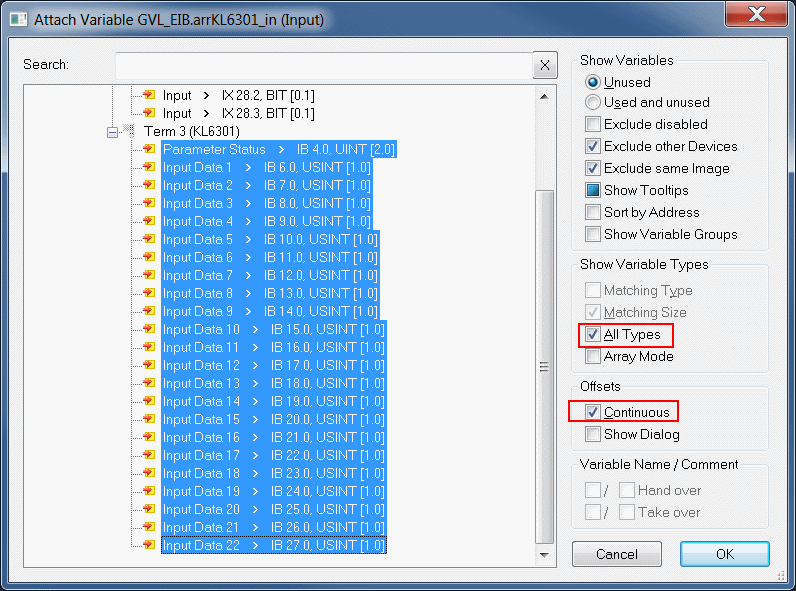
You can now check the link. To do this go onto the KL6301 and open it. All terminal data should now show a small arrow. If that is the case, then proceed in exactly the same way with the outputs.