Creating and handling Profibus devices
This article explains how to create and handle Profibus Master and Slave devices via TwinCAT Automation Interface. It consists of following main points:
- Creating and adding a Profibus Master
- Searching appropriate device (EL6731, FC310x, etc.) and configuring
- Creating and adding a Profibus Slave
- Searching appropriate Slave device (EL6731-0010, etc.) and configuring
- Changing fieldbus address
Creating and adding a Profibus Master
- 1. To create a Profibus Master device, open a new or an existing TwinCAT configuration
- 2. Scan all the devices. (These actions can also be performed via Automation Interface.)
- 3. Create a system manager object and navigate to devices
Code Snippet (C#):
project = solution.Projects.Item(1);
sysman = (ItcSysManager)project.Object;
ITcSmTreeItem io = (ITcSmTreeItem)sysman.LookupTreeItem("TIID");
Code Snippet (Powershell):
$project = $sln.Projects.Items(1)
$sysman = $project.Object
$io = $sysman.LookupTreeItem("TIID")
To add a Profibus master, use ITcSmTreeItem:CreateChild method.
Code Snippet (C#):
ITcSmTreeItem5 profi_master = (ITcSmTreeItem5)io.CreateChild("Device 2 (EL6731)", 86, "", null);
Code Snippet (Powershell):
$profi_master = $io.CreateChild("Device 2 (EL6731) ", "86", "", $null)
For other Profibus Masters, enter the correct ItemSubtype listed here. This will add the new device as shown in the screenshot:
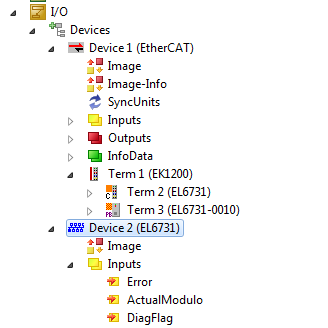
Searching and claiming a Profibus Master device from the list:
It is necessary to configure the newly added Profibus master, which is usually done in TwinCAT by pressing the search button and selecting the correct device from the list:
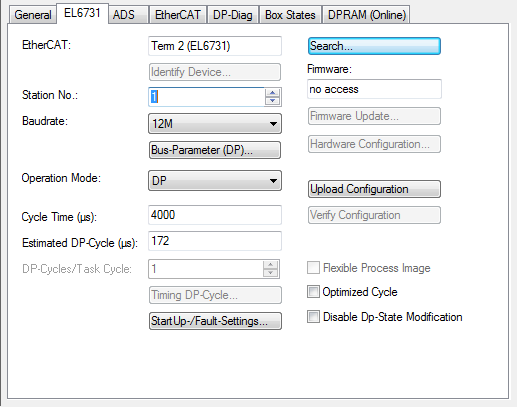
This can be done via Automation Interface:
Code Snippet (C#):
string availableMaster = profi_master.ResourcesCount;
profi_master.ClaimResources(1);
Code Snippet (Powershell):
$availableMaster = $profi_master.ResourcesCount
$profi_master.ClaimResources(1)
The ITcSmTreeItem5:ResourcesCount gives out the number of compatible Profibus master devices, and the ITcSmTreeItem5:ClaimResources takes the index of the CANOpen device to be configured as the master.
Creating and adding a Profibus slave
A Profibus slave can be added to the current configuration as shown below:
Code Snippet (C#):
ITcSmTreeItem5 profi_slave = (ITcSmTreeItem5)io.CreateChild("Device 3 (EL6731-0010)", 97, null);
Code Snippet (Powershell):
$profi_slave = $io.CreateChild("Device 3 (EL6731-0010)", "97", "", $null)
Searching and claiming a Profibus slave
Similar to Profibus master, number of Profibus slaves can be published by following code:
Code Snippet (C#):
string availableSlaves = profi_slave.ResourcesCount;
profi_slave.ClaimResource(1);
Code Snippet (Powershell):
$availableSlaves = $profi_slave.ResourcesCount
$profi_slave.ClaimResources(1)
The last line in code claims the EL6731-0010 as the Profibus slave, similar to the dialog box that appears in TwinCAT user interface.
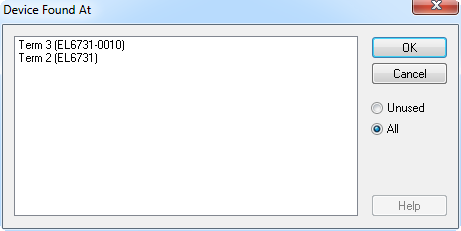
Changing fieldbus address
To change the the fieldbus address (station no.) of a profibus box in a configuration, an instance of the TwinCAT System Manager has to be created and the configuration has to be opened. The LookupTreeItem method of the ITcSysManager interface returns a ITcSmTreeItem interface pointer implemented by the tree item referenced by its pathname. This interface contains a ConsumeXml method of the tree item.
Procedure
The Procedure to create the ITcSysManager interface (the 'sysMan' instance here) is described in the chapter Accessing TwinCAT Configurations. This interface has a LookupTreeItem method that returns a ITcSmTreeItem pointer to a specific tree item given by its pathname. To change the the fieldbus address (station no.) of a profibus box "TIID^Device 1 (FC310x)^Box 1 (BK3100)" to 44 the following code can be used:
Code Snippet (C#):
ItcSmTreeItem item = sysMan.LookupTreeItem("TIID^Device 1 (FC310x)^Box 1 (BK3100)");
item.ConsumeXml("44");
Code Snippet (PowerShell):
$item = $sysMan.LookupTreeItem("TIID^Device 1 (FC310x)^Box 1 (BK3100)")
$item.ConsumeXml("44")