Display of technology data
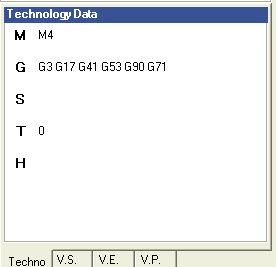
The currently active technology data such as G functions, zero shifts and rotation can be read via ADS.
Activation for reading the technology data
In order to read the above-mentioned parameters, activation via ADS is required first.
The function must be activated before the start of the NC program, or earlier. It remains active until either a TwinCAT restart is performed or the function is reset explicitly.
Function |
ADS-Write |
Port |
500 (dec) |
Index Group |
0x2000 + channel ID |
Index Offset |
0x0053 |
Data |
DWORD |
Reading the currently active zero shift
This command reads the active zero shift of the segment currently in block execution (SAF). If no zero shift is active (G53), the structure for the individual components contains a zero vector. These data can be used for switching the display between machine coordinates and programming coordinates, for example.
The data, which are read with the function block 'ItpReadZeroShift', for example, may differ from these values, since the interpreter data are read with the function block, which may already take into account new offsets.
Function |
ADS-Read | |
Port |
500 (dec) | |
Index Group |
0x2100 + channel ID | |
Index Offset |
0x0014 | |
Data |
{ |
|
UINT32 |
block counter | |
UINT32 |
dummy | |
LREAL[3] |
zero shift G54..G57 | |
LREAL[3] |
zero shift G58 | |
LREAL[3] |
zero shift G59 | |
} |
|
Reading the currently active rotation
This command reads the active rotation of the segment currently in block execution (SAF).
Function |
ADS-Read | |
Port |
500 (dec) | |
Index Group |
0x2100 + channel ID | |
Index Offset |
0x0015 | |
Data |
{ |
|
UINT32 |
block counter | |
UINT32 |
dummy | |
LREAL[3] |
rotation of X, Y & Z in degrees | |
} |
|
Reading the currently active G-Code
The G-Code is subdivided into groups. For example, the geometries types with modal effect (G01, G02...) and the plane selection (G17..G19) form separate groups. When the G-Code information is read, the enumerator for the groups is also read. These can then be displayed in an application-specific manner.
Since the read command comes with a parameter to be read, not all groups have to be read. The memory provided is always filled by group 1. If, for example, the transferred memory size is 3x8 bytes, the data for the block counter, group 1 and 2 are returned.
Function |
ADS-Read | |
Port |
500 (dec) | |
Index Group |
0x2100 + channel ID | |
Index Offset |
0x0013 | |
Data |
{ |
|
UINT32 |
block counter | |
UINT32 |
Group 1: ModalGeoTypes | |
UINT32 |
Group 2: BlockwiseGeoTypes | |
UINT32 |
Group 3: ModalPlaneSelection | |
UINT32 |
Group 4: ModalToolCompensation | |
UINT32 |
Group 5: ModalToolFeedDirection | |
UINT32 |
Group 6: ModalZeroShift | |
UINT32 |
Group 7: ModalAccurateStop | |
UINT32 |
Group 8: BlockwiseAccurateStop | |
UINT32 |
Group 9: ModalDesignationAbsInc | |
UINT32 |
Group 10: ModalDesignationInchMetric | |
UINT32 |
Group 11: ModalFeedRateInCurve | |
UINT32 |
Group 12: ModalCenterpointCorr | |
UINT32 |
Group 13: ModalCircleCpAbsInc | |
UINT32 |
Group 14: ModalCollisionDetection | |
UINT32 |
Group 15: ModalRotation | |
UINT32 |
Group 16: ModalCalcExRot | |
UINT32 |
Group 17: ModalDiam | |
UINT32 |
Group 18: ModalFeedrateIpol | |
UINT32 |
Group 19: ModalMirror | |
} |
|
#define GCodeOffset 0x1000
#define CommonIdentOffset 0x2000 // used for non-g-code commands, like rot, cfc...
Group 1: ModalGeoTypes
enum GCodeGroup_ModalGeoTypes
{
ModalGeoTypeUndefined = 0,
ModalGeoTypeG0 = 0 + GCodeOffset, // line - rapid traverse
ModalGeoTypeG01 = 1 + GCodeOffset, // straight line
ModalGeoTypeG02 = 2 + GCodeOffset, // circle clockwise
ModalGeoTypeG03 = 3 + GCodeOffset // circle anticlockwise
};
Group 2: BlockwiseGeoTypes
enum GCodeGroup_BlockwiseGeoTypes
{
BlockwiseGeoTypeNone = 0,
BlockwiseGeoTypeG04 = 4 + GCodeOffset, // dwell time
BlockwiseGeoTypeG74 = 74 + GCodeOffset, // homing
BlockwiseGeoTypeCip = 1 + CommonIdentOffset // circle parametrized with 3 points
};
Group 3: ModalPlaneSelection
enum GCodeGroup_ModalPlaneSelection
{
ModalPlaneSelectUndefined = 0,
ModalPlaneSelectG17 = 17 + GCodeOffset, // xy-plane
ModalPlaneSelectG18 = 18 + GCodeOffset, // zx-plane
ModalPlaneSelectG19 = 19 + GCodeOffset // yz-plane
};
Group 4: ModalToolCompensation
enum GCodeGroup_ModalToolCompensation
{
ModalToolCompUndefined = 0,
ModalToolCompG40 = 40 + GCodeOffset, // tool compensation off
ModalToolCompG41 = 41 + GCodeOffset, // tool compensation left
ModalToolCompG42 = 42 + GCodeOffset // tool compensation right
};
Group 5: ModalToolFeedDirection
enum GCodeGroup_ModalToolFeedDirection
{
ModalToolFeedDirUndefined = 0,
ModalToolFeedDirPos = 2 + CommonIdentOffset, // tool feed direction positive
ModalToolFeedDirNeg = 3 + CommonIdentOffset // tool feed direction negative
};
Group 6: ModalZeroShift
enum GCodeGroup_ModalZeroShift
{
ModalZeroShiftUndefined = 0,
ModalZeroShiftG53 = 53 + GCodeOffset, // zero shift off
ModalZeroShiftG54G58G59 = 54 + GCodeOffset, // zero shift G54 + G58+ G59
ModalZeroShiftG55G58G59 = 55 + GCodeOffset, // zero shift G55 + G58+ G59
ModalZeroShiftG56G58G59 = 56 + GCodeOffset, // zero shift G56 + G58+ G59
ModalZeroShiftG57G58G59 = 57 + GCodeOffset // zero shift G57 + G58+ G59
};
Group 7: ModalAccurateStop
enum GCodeGroup_ModalAccurateStop
{
ModalAccurateStopNone = 0,
ModalAccurateStopG60 = 60 + GCodeOffset // modal accurate stop
};
Group 8: BlockwiseAccurateStop
enum GCodeGroup_BlockwiseAccurateStop
{
BlockwiseAccurateStopNone = 0,
BlockwiseAccurateStopG09 = 9 + GCodeOffset, // common accurate stop
BlockwiseAccurateStopTpm = 4 + CommonIdentOffset // target position monitoring
};
Group 9: ModalDesignationAbsInc
enum GCodeGroup_ModalDesignationAbsInc
{
ModalDesignAbsIncUndefined = 0,
ModalDesignAbsIncG90 = 90 + GCodeOffset, // absolute designation
ModalDesignAbsIncG91 = 91 + GCodeOffset // incremental designation
};
Group 10: ModalDesignationInchMetric
enum
GCodeGroup_ModalDesignationInchMetric
{
ModalDesignInchMetricUndefined = 0,
ModalDesignInchMetricG70 = 70 + GCodeOffset, // designation inch
ModalDesignInchMetricG71 = 71 + GCodeOffset, // designation metric
ModalDesignInchMetricG700 = 700 + GCodeOffset, // designation inch & feedrate recalculated
ModalDesignInchMetricG710 = 710 + GCodeOffset // designation metric & feedrate recalculated
};
Group 11: ModalFeedRateInCurve
enum GCodeGroup_ModalFeedRateInCurve
{
ModalFeedRateInCurveUndefined = 0,
ModalFeedRateInCurveCfc = 5 + CommonIdentOffset, // constant feed contour
ModalFeedRateInCurveCfin = 6 + CommonIdentOffset, // constant feed inner contour
ModalFeedRateInCurveCftcp = 7 + CommonIdentOffset // constant feed tool center point
};
Group 12: ModalCenterpointCorr
enum GCodeGroup_ModalCenterpointCorr
{
ModalCenterpointCorrUndefined = 0,
ModalCenterpointCorrOn = 8 + CommonIdentOffset, // circle centerpoint correction on
ModalCenterpointCorrOff = 9 + CommonIdentOffset // circle centerpoint correction off
};
Group 13: ModalCircleCpAbsInc
enum GCodeGroup_ModalCircleCpAbsInc
{
ModalCircleCpUndefined = 0,
ModalCircleCpIncremental = 10 + CommonIdentOffset, // circle centerpoint incremental to start point
ModalCircleCpAbsolute = 11 + CommonIdentOffset // circle centerpoint absolute
};
Group 14: ModalCollisionDetection
enum GCodeGroup_ModalCollisionDetection
{
ModalCollisionDetectionUndefined = 0,
ModalCollisionDetectionOn = 12 + CommonIdentOffset, //collision detection on
ModalCollisionDetectionOff = 13 + CommonIdentOffset //collision detection off
};
Group 15: ModalRotation
enum GCodeGroup_ModalRotation
{
ModalRotationUndefined = 0,
ModalRotationOn = 14 + CommonIdentOffset, // rotation is turned on
ModalRotationOff = 15 + CommonIdentOffset // rotation is turned off
};
Group 16: ModalCalcExRot
enum GCodeGroup_ModalCalcExRot
{
ModalCalcExRotUndefined = 0,
ModalCalcExRotOn = 16 + CommonIdentOffset, // extended calculation for rotation turned on
ModalCalcExRotOff = 17 + CommonIdentOffset // extended calculation for rotation turned off
};
Group 17: ModalDiam
enum GCodeGroup_ModalDiam
{
ModalDiamUndefined = 0,
ModalDiamOn = 18 + CommonIdentOffset, // diameter programming on
ModalDiamOff = 19 + CommonIdentOffset // diameter programming off
};
Group 18: ModalFeedrateIpol
enum GCodeGroup_ModalFeedrateIpol
{
ModalFeedrateIpolUndefined = 0,
ModalFeedrateIpolConst = 20 + CommonIdentOffset, // federate interpolation constant (default)
ModalFeedrateIpolLinear = 21 + CommonIdentOffset // federate interpoaltion linear to remaining path
};
Group 19: ModalMirror
enum GCodeGroup_ModalMirror
{
// value - (32+CommonIdentOffset) shows the bitmask for mirrored axes
// that's why the sequence seems to be strange...
//
ModalMirrorUndefined = 0,
ModalMirrorOff = 32 + CommonIdentOffset,
ModalMirrorX = 33 + CommonIdentOffset,
ModalMirrorY = 34 + CommonIdentOffset,
ModalMirrorXY = 35 + CommonIdentOffset,
ModalMirrorZ = 36 + CommonIdentOffset,
ModalMirrorZX = 37 + CommonIdentOffset,
ModalMirrorYZ = 38 + CommonIdentOffset,
ModalMirrorXYZ = 39 + CommonIdentOffset
};