Function blocks
Basic structure of the function blocks
All function blocks in the TwinCAT Weighing PLC Library are based on the same basic structure. This simplifies the engineering process when changing from one Weighing type to another.
Syntax
FUNCTION_BLOCK FB_WG_<type>
VAR_INPUT
stConfig : ST_WG_<type>;
END_VAR
VAR_OUTPUT
bError : BOOL;
bConfigured : BOOL;
ipResultMessage : I_TCMessage;
END_VAR
Inputs
To configure the Weighing function block, a configuration structure of type ST_WG_<type> is transferred to the function blocks during instantiation. The configuration structure can be assigned in the declaration or via the method Configure()
at runtime.
See also: Data types > Configuration structures
Sample of configuration in the declaration:
(* define configure structure - exemplary for ComboFilter *)
stParams : ST_WG_ComboFilter := (
nOrder := nOrder,
fCutoff := fCutoff,
fSamplingRate := fSampleRate,
nSamplesToFilter := nSamplesToFilter);
(* create filter instance with configure structure *)
fbFilter : FB_WG_ComboFilter := (stConfig := stParams);
Outputs
All function blocks have an error flag bError
and a flag bConfigured
of type BOOL
as output parameters. These indicate whether an error has occurred and whether the corresponding function block instance has been successfully configured. The output ipResultMessage
of type I_TcMessage
provides various properties for explaining the cause of an event and methods for processing the message (event list).
See also: I_TcEventBase und I_TcMessage
Methods
All function blocks in the Tc3_Weighing library have three methods. They return a positive value if they were executed without errors.
Configure()
The method can be used at runtime to initially configure the instance of a Weighing function block (if not already done in the declaration) or to reconfigure it.
METHOD Configure : BOOL
VAR_INPUT
stConfig : ST_WG_<type>;
END_VAR
Call()
The method calculates a manipulated output signal from an input signal that is transferred in the form of a pointer.
METHOD Call : BOOL
VAR_INPUT
pIn : POINTER TO LREAL; (*address of input array*)
nSizeIn : UDINT; (*size of input array*)
pOut : POINTER TO LREAL; (*address of output array*)
nSizeOut : UDINT; (*size of output array*)
END_VAR
Reset()
The method resets the internal status of a Weighing function block. The influence of the past values on the current output value is eliminated.
METHOD Reset : BOOL
Properties
The Tc3_Weighing library references the TwinCAT 3 EventLogger and thus ensures that information (events) is provided via the standardized interface I_TcMessage.
Each function block has the properties eTraceLevel
of type TcEventSeverity
and eTraceLevelDefault
of type BOOL
.
The trace level determines the severity of an event (Verbose, Info, Warning, Error, Critical) and is set via the property eTraceLevel
.
(* Sample of setting fbFilter to trace level info *)
fbFilter.eTraceLevel := TcEventSeverity.Info;
The property eTraceLevelDefault
can be used to set the trace level back to the default value (TcEventSeverity.Warning
).
The property can be read and written, i.e. the property eTraceLevelDefault
can be used to query whether the default value is set.
The properties can also be set in Online View.
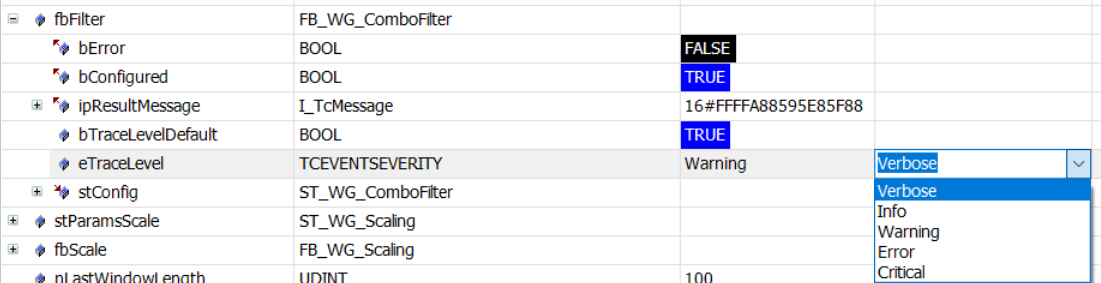
Dealing with oversampling
All function blocks are oversampling-capable, whereby different types of use are possible. The declaration of the Weighing function block instance fbFilter
is always the same here.
1-channel application with oversamples
The input and output arrays can be declared as one-dimensional variables.
VAR CONSTANT
cOversamples : UINT := 10;
END_VAR
VAR
aInput : ARRAY [1..cOversamples] OF LREAL;
aOutput : ARRAY [1..cOversamples] OF LREAL;
END_VAR
bSucceed := fbFilter.Call(ADR(aInput), SIZEOF(aInput), ADR(aOutput), SIZEOF(aOutput));
1-channel application without oversamples
If no oversampling is used, the input and output variables can also be declared as LREAL
.
VAR CONSTANT
cOversamples : UINT := 1;
END_VAR
VAR
fInput : LREAL;
fOutput : LREAL;
END_VAR
bSucceed := fbFilter.Call(ADR(fInput), SIZEOF(fInput), ADR(fOutput), SIZEOF(fOutput));