FB_MaximumDemandController
Function block for peak load optimization, which ensures compliance with the set power limit by means of switching on or off up to eight consumers. The consumers can be switched off according to their rated power and priority in such a manner that the production sequence is not disturbed.
To distinguish the individual measurement cycles, a synchronization pulse is supplied by the electricity supply company (ESC). This indicates the start of a new measurement cycle and must be connected to the input bPeriodPuls. The actual power is recorded via the counter terminal KL1501.
The block works with a fixed measurement period of 15 minutes. If the synchronization pulse exceeds the 16-minute limit, the output bEmergencySignal is set.
All consumers are switched on at the start of each measurement period. If the power limit (fAgreedPower) threatens to be exceeded within the measurement period, the consumers are switched off one after the other. If the danger of an excess load no longer exists, the consumers are switched on again.
Special items, such as minimum power-on time, minimum power-off time or maximum power-off time can be specified via an input variable. The priority of the individual consumers can similarly be determined. Consumers with a low priority will be switched off before consumers with a high priority.
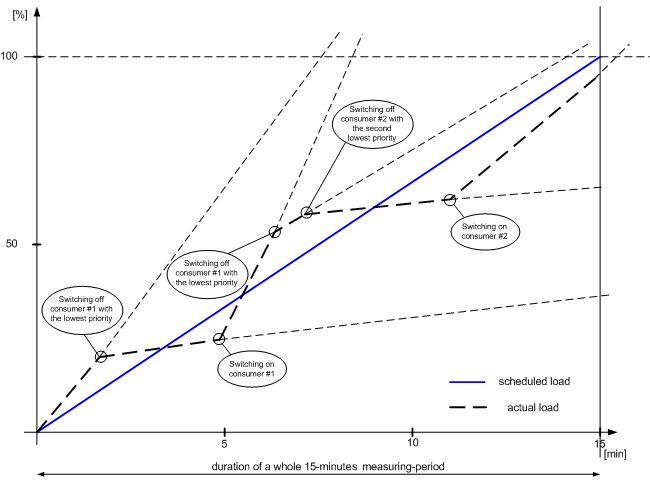
VAR_INPUT
bStart : BOOL;
fMeterConstant : LREAL;
fAgreedPower : LREAL;
bPeriodPulse : BOOL;
arrLoadParameter : ARRAY[1..8] OF ST_MDCLoadParameters;
bStart: The block is activated by a rising edge at this input.
fMeterConstant: Meter constant [pulses / kWh].
fAgreedPower: This is the agreed power limit which, as far as possible, should not be exceeded in the operational case [kW].
bPeriodPulse: Synchronisation pulse sent by the electricity supply company (ESC). This pulse starts the measurement interval.
arrLoadParameter: Parameter structure of the respective consumer. This consists of the following elements:
TYPE ST_MDCLoadParameters: STRUCT bConnected : BOOL; nDegreeOfPriority : INT; tMINPowerOnTime : TIME; tMINPowerOffTime : TIME; tMAXPowerOffTime : TIME; END_STRUCT END_TYPE |
bConnected: TRUE = consumer connected; FALSE = consumer not connected.
nDegreeOfPriority: Indicates the switch-off priority; consumers with a low priority will be switched off first. ( 1 => lox; ... 8 => high priority)
tMINPowerOnTime: The minimum power-on time (minimum ramp-up time) during which the consumer may not be switched off.
tMINPowerOffTime: The minimum power-off time (recovery time) during which the consumer may not be switched on again.
tMAXPowerOffTime: The maximum power-off time after which the consumer must be switched on again.
VAR_OUTPUT
arrLoad : ARRAY[1..8] OF BOOL;
fAgreedEnergy : LREAL;
fInstantaneousEnergy : LREAL;
fActualEnergy : LREAL;
tRemainingTime : TIME;
fLastPeriodEnergy : LREAL;
bEmergencySignal : BOOL;
bError : BOOL;
nErrorId : UDINT;
arrLoad: This is an array of data type BOOL; consumers that are switched on are TRUE.
fAgreedEnergy: Agreed energy consumption [kWh].
fInstantaneousEnergy: Momentary energy consumption [kWh] in relation of the integration period with 15s (internal measurement interval).
fActualEnergy: Energy consumed at the "presently" observed point in time of the measurement period.
tRemainingTime: Time remaining until the next measurement interval.
fLastPeriodEnergy: Rated power from the preceding measurement period [kWh].
bEmergencySignal: This output is set as soon as the specified energy is exceeded.
bError: This output is switched to TRUE if an error occurs during the execution of a command.
nErrorId: Contains the Errorcode.
VAR_IN_OUT
stInDataKL1501 : ST_MDCInDataKL1501;
stOutDataKL1501 : ST_MDCOutDataKL1501;
stInDataKL1501: Linked to the KL1501.
TYPE ST_MDCInDataKL1501 :
STRUCT
nStatus : USINT;
nDummy1 : USINT;
nDummy2 : USINT;
nDummy3 : USINT;
nData : DWORD;
END_STRUCT
END_TYPE
stOutDataKL1501: Linked to the KL1501.
TYPE ST_MDCOutDataKL1501 :
STRUCT
nCtrl : USINT;
nDummy1 : USINT;
nDummy2 : USINT;
nDummy3 : USINT;
nData : DWORD;
END_STRUCT
END_TYPE
Sample
VAR_GLOBAL
arrLoadParameters AT %MB100 : ARRAY [1..8] OF ST_MDCLoadParameters;
(* KL1002 *)
bPeriodPulse AT %IX6.0 : BOOL;
(* KL1501*)
stInDataKL1501 AT %IB0 : ST_MDCInDataKL1501;
stOutDataKL1501 AT %QB0 : ST_MDCOutDataKL1501;
(* KL2404 *)
bLoadOut1 AT %QX6.0 : BOOL;
bLoadOut2 AT %QX6.1 : BOOL;
bLoadOut3 AT %QX6.2 : BOOL;
bLoadOut4 AT %QX6.3 : BOOL;
(* KL2404 *)
bLoadOut5 AT %QX6.4 : BOOL;
bLoadOut6 AT %QX6.5 : BOOL;
bLoadOut7 AT %QX6.6 : BOOL;
bEmergencySignal AT %QX6.7 : BOOL;
END_VAR
PROGRAM MAIN
VAR
fbMaximumDemandController : FB_MaximumDemandController;
END_VAR
arrLoadParameters[1].bConnected := TRUE;
arrLoadParameters[1].nDegreeOfPriority := 1;
arrLoadParameters[1].tMINPowerOnTime := t#60s;
arrLoadParameters[1].tMINPowerOffTime := t#120s;
arrLoadParameters[1].tMAXPowerOffTime := t#600s;
arrLoadParameters[2].bConnected := TRUE;
arrLoadParameters[2].nDegreeOfPriority := 2;
arrLoadParameters[2].tMINPowerOnTime := t#60s;
arrLoadParameters[2].tMINPowerOffTime := t#120s;
arrLoadParameters[2].tMAXPowerOffTime := t#600s;
arrLoadParameters[3].bConnected := TRUE;
arrLoadParameters[3].nDegreeOfPriority := 3;
arrLoadParameters[3].tMINPowerOnTime := t#60s;
arrLoadParameters[3].tMINPowerOffTime := t#120s;
arrLoadParameters[3].tMAXPowerOffTime := t#300s;
arrLoadParameters[4].bConnected := TRUE;
arrLoadParameters[4].nDegreeOfPriority := 4;
arrLoadParameters[4].tMINPowerOnTime := t#20s;
arrLoadParameters[4].tMINPowerOffTime := t#30s;
arrLoadParameters[4].tMAXPowerOffTime := t#8m;
arrLoadParameters[5].bConnected := TRUE;
arrLoadParameters[5].nDegreeOfPriority := 5;
arrLoadParameters[5].tMINPowerOnTime := t#20s;
arrLoadParameters[5].tMINPowerOffTime := t#50s;
arrLoadParameters[5].tMAXPowerOffTime := t#20m;
arrLoadParameters[6].bConnected := TRUE;
arrLoadParameters[6].nDegreeOfPriority := 6;
arrLoadParameters[6].tMINPowerOnTime := t#30s;
arrLoadParameters[6].tMINPowerOffTime := t#1m;
arrLoadParameters[6].tMAXPowerOffTime := t#1m;
arrLoadParameters[7].bConnected := TRUE;
arrLoadParameters[7].nDegreeOfPriority := 7;
arrLoadParameters[7].tMINPowerOnTime := t#0s;
arrLoadParameters[7].tMINPowerOffTime := t#0s;
arrLoadParameters[7].tMAXPowerOffTime := t#1m;
arrLoadParameters[8].bConnected := FALSE;
fbMaximumDemandController(bStart := TRUE,
fMeterConstant := 20000,
fAgreedPower := 600,
bPeriodPulse := bPeriodPulse,
arrLoadParameters := arrLoadParameters,
stInDataKL1501 := stInDataKL1501,
stOutDataKL1501 := stOutDataKL1501);
bLoadOut1 := fbMaximumDemandController.arrLoad[1];
bLoadOut2 := fbMaximumDemandController.arrLoad[2];
bLoadOut3 := fbMaximumDemandController.arrLoad[3];
bLoadOut4 := fbMaximumDemandController.arrLoad[4];
bLoadOut5 := fbMaximumDemandController.arrLoad[5];
bLoadOut6 := fbMaximumDemandController.arrLoad[6];
bLoadOut7 := fbMaximumDemandController.arrLoad[7];
bEmergencySignal := fbMaximumDemandController.bEmergencySignal;