FB_JsonDomParser
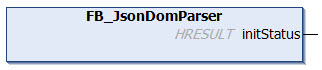
This function block is derived from the same internal function block as FB_JsonDynDomParser and thus offers the same interface.
The two derived function blocks differ only in their internal memory management. FB_JsonDomParser is optimized for the fast and efficient parsing and creation of JSON documents that are only changed a little. The function block FB_JsonDynDomParser is recommended for JSON documents to which many changes are made (e.g. the cyclic changing of a specific value in the JSON document).
| |
Use of router memory The function block occupies new memory with each change, e.g. with the methods SetObject() or SetJson(). As a result, the amount of router memory used can grow enormously after repeated actions. This allocated memory is only released again by calling the NewDocument() or ParseDocument() methods. |
![]() | Strings in UTF-8 format The variables of type STRING used here are based on the UTF-8 format. This STRING formatting is common for MQTT communication as well as for JSON documents. In order to be able to receive special characters and texts from a wide range of languages, the character set in the Tc3_IotBase and Tc3_JsonXml libraries is not limited to the typical character set of the data type STRING. Instead, the Unicode character set in UTF-8 format is used in conjunction with the data type STRING. If the ASCII character set is used, there is no difference between the typical formatting of a STRING and the UTF-8 formatting of a STRING. Further information on the UTF-8 STRING format and available display and conversion options can be found in the documentation for the Tc2_Utilities PLC library. |
Syntax
FUNCTION_BLOCK FB_JsonDomParser
VAR_OUTPUT
initStatus : HRESULT;
END_VAR
Outputs
Name | Type |
---|---|
initStatus | HRESULT |
Methods
Name | Description |
---|---|
Adds an Array member to a JSON object. | |
Adds a Base64 member to a JSON object. | |
Adds a Bool member to a JSON object. | |
Adds a DateTime member to a JSON object. | |
Adds a DcTime member to a JSON object. | |
Adds a Double member to a JSON object. | |
Adds a FileTime member to a JSON object. | |
Adds a HexBinary member to a JSON object. | |
Adds an Int64 member to a JSON object. | |
Adds an Int member to a JSON object. | |
Adds a JSON member to a JSON object. | |
Adds a NULL member to a JSON object. | |
Adds an Object member to a JSON object. | |
Adds a String member to a JSON object. | |
Adds an UInt64 member to a JSON object. | |
Adds an UInt member to a JSON object. | |
Returns the first element of an array. | |
Returns the last element of an array. | |
Deletes the contents of an array. | |
Copies the contents of the DOM memory into a variable of data type STRING. | |
Extracts a JSON object from a key and stores it in a variable of data type STRING. | |
Copies the value of a key into a variable of data type STRING. | |
Searches for a specific property in a JSON document. | |
Searches for a specific property in a JSON document (path-specific). | |
Returns the number of elements in a JSON array. | |
Returns the value at the current iterator position of an array. | |
Returns the value of an array at a specified index. | |
Decodes a Base64 value from a JSON property. | |
Returns the value of a property of the data type BOOL. | |
Returns the value of a property of the data type DATE_AND_TIME. | |
Returns the value of a property of the data type DCTIME. | |
Returns the content of the DOM memory as the data type STRING(255). | |
Returns the length of a JSON document in the DOM memory. | |
Returns the root node of a JSON document in the DOM memory. | |
Returns the value of a property of the data type LREAL. | |
Returns the value of a property of the data type DCTIME. | |
Decodes the HexBinary content of a property and writes it to a certain memory address, | |
Returns the value of a property of the data type DINT. | |
Returns the value of a property of the data type LINT. | |
Returns the value of a property of the data type STRING(255). | |
Returns the length of a property if this is a JSON document. | |
Returns the current setting for MaxDecimalPlaces. | |
Returns the name of a JSON property member at the position of the current iterator, | |
Returns the value of a JSON property member at the position of the current iterator, | |
Returns the value of a property of the data type STRING(255). | |
Returns the length of a property if its value is a string. | |
Returns the type of a property value. | |
Returns the value of a property of the data type UDINT. | |
Returns the value of a property of the data type ULINT. | |
Checks whether a certain property is present in the DOM memory. | |
Checks whether a given property is an array. | |
Checks whether the value of a given property is of the data type Base64. | |
Checks whether the value of a given property is of the data type BOOL. | |
Checks whether the value of a given property is of the data type Double (PLC: LREAL). | |
Checks whether the value of a given property is FALSE. | |
Checks whether the value of a property is in the HexBinary format. | |
Checks whether the value of a given property is of the data type Integer (PLC: DINT) . | |
Checks whether the value of a given property is of the data type LINT. | |
Checks whether the value of a given property has a time format according to ISO8601. | |
Checks whether the value of a given property is NULL. | |
Checks whether the value of a given property is a numerical value. | |
Checks whether the given property is a further JSON object. | |
Checks whether the value of a given property is of the data type STRING. | |
Checks whether the value of a given property is TRUE. | |
Checks whether the value of a given property is of the data type UDINT. | |
Checks whether the value of a given property is of the data type ULINT. | |
Loads a JSON document from a file. | |
Returns the first child element below a JSON property. | |
Returns the last child element below a JSON property. | |
Generates a new empty JSON document in the DOM memory. | |
Returns the next element in an array. | |
Returns the next property in a JSON document. | |
Loads a JSON object into the DOM memory for further processing. | |
PopbackValue | Deletes the element at the end of an array. |
Appends a Base64 value to the end of an array. | |
Appends a Base64 value to the end of an array. | |
Appends a value of the data type DATE_AND_TIME to the end of an array. | |
Appends a value of the data type DCTIME to the end of an array. | |
Appends a value of the data type Double to the end of an array. | |
Appends a value of the data type FILETIME to the end of an array. | |
This method appends a HexBinary-coded value to the end of an array. | |
Appends a value of the data type Int64 to the end of an array. | |
Appends a value of the data type INT to the end of an array. | |
Appends a JSON document to the end of an array. | |
Appends a NULL value to the end of an array. | |
Appends a value of the data type String to the end of an array. | |
Appends a value of the data type UInt64 to the end of an array. | |
Appends a value of the data type UInt to the end of an array. | |
Removes all child elements from a given property. | |
Deletes the value of the current array iterator. | |
Deletes the property at the current iterator. | |
Removes a child element from a given property. | |
Saves a JSON document in a file. | |
Sets the value of a property to the type "Array". | |
Sets the value of a property to a Base64-coded value. | |
Sets the value of a property to a value of the data type BOOL. | |
Sets the value of a property to a value of the data type DATE_AND_TIME. | |
Sets the value of a property to a value of the data type DCTIME. | |
Sets the value of a property to a value of the data type Double. | |
Sets the value of a property to a value of the data type FILETIME. | |
Sets the value of a property to a HexBinary-coded value. | |
Sets the value of a property to a value of the data type INT | |
Sets the value of a property to a value of the data type Int64. | |
Inserts a further JSON document into the value of a property. | |
Sets the current setting for MaxDecimalPlaces. | |
Sets the value of a property to the value NULL. | |
Sets the value of a property to the type "Object". | |
Sets the value of a property to a value of the data type STRING. | |
Sets the value of a property to a value of the data type UInt. | |
Sets the value of a property to a value of the data type UInt64. |
Requirements
TwinCAT version | Hardware | Libraries to be integrated |
---|---|---|
TwinCAT 3.1, Build 4022 | x86, x64, ARM | Tc3_JsonXml |
- LoadDocumentFromFile
- AddArrayMember
- AddBase64Member
- AddBoolMember
- AddDateTimeMember
- AddDcTimeMember
- AddDoubleMember
- AddFileTimeMember
- AddHexBinaryMember
- AddInt64Member
- AddIntMember
- AddJsonMember
- AddNullMember
- AddObjectMember
- AddStringMember
- AddUint64Member
- AddUintMember
- ArrayBegin
- ArrayEnd
- ClearArray
- CopyDocument
- CopyFrom
- CopyJson
- CopyString
- ExceptionRaised
- FindMember
- FindMemberPath
- GetArraySize
- GetArrayValue
- GetArrayValueByIdx
- GetBase64
- GetBool
- GetDateTime
- GetDcTime
- GetDocument
- GetDocumentLength
- GetDocumentRoot
- GetDouble
- GetFileTime
- GetHexBinary
- GetInt
- GetInt64
- GetJson
- GetJsonLength
- GetMaxDecimalPlaces
- GetMemberName
- GetMemberValue
- GetString
- GetStringLength
- GetType
- GetUint
- GetUint64
- HasMember
- IsArray
- IsBase64
- IsBool
- IsDouble
- IsFalse
- IsHexBinary
- IsInt
- IsInt64
- IsISO8601TimeFormat
- IsNull
- IsNumber
- IsObject
- IsString
- IsTrue
- IsUint
- IsUint64
- MemberBegin
- MemberEnd
- NewDocument
- NextArray
- NextMember
- ParseDocument
- PushbackBase64Value
- PushbackBoolValue
- PushbackCopyValue
- PushbackDateTimeValue
- PushbackDcTimeValue
- PushbackDoubleValue
- PushbackFileTimeValue
- PushbackHexBinaryValue
- PushbackInt64Value
- PushbackIntValue
- PushbackJsonValue
- PushbackNullValue
- PushbackStringValue
- PushbackUint64Value
- PushbackUintValue
- RemoveAllMembers
- RemoveArray
- RemoveMember
- RemoveMemberByName
- SaveDocumentToFile
- SetAdsProvider
- SetArray
- SetBase64
- SetBool
- SetDateTime
- SetDcTime
- SetDouble
- SetFileTime
- SetHexBinary
- SetInt
- SetInt64
- SetJson
- SetMaxDecimalPlaces
- SetNull
- SetObject
- SetString
- SetUint
- SetUint64
- Swap