FB_GetDPRAMInfo
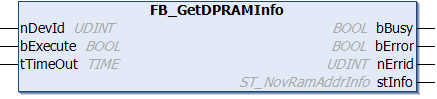
The FB_GetDPRAMInfo function block determines the address pointer and the configured size of the NOV/DP-RAM of a fieldbus card. The address pointer can be used, for example, for direct write or read access of the NOV-RAM of the FCxxx-0002 (Beckhoff) cards or the DPRAM of cards that are not supported by TwinCAT (third-party manufacturers). First, the card has to be configured as general NOV/DP-RAM within the TwinCAT system. The MEMCPY, MEMSET or MEMCMP functions of the PLC program can then be used for write/read access to any memory offset.
This function block returns a Service Not Supported error (16#701) if the NOV/DP-RAM requires a special access method (e.g. BYTE, aligned WORD). In this case, the FB_NovRamReadWriteEx function block can be used to read data from the NOV/DP-RAM or to write data to the NOV/DP-RAM. For example, the NOV-RAM of the CX9000 only allows BYTE accesses, so that the FB_NovRamReadWriteEx function block should be used in this case.
Inputs
VAR_INPUT
nDevId : UDINT;
bExecute : BOOL;
tTimeOut : TIME := DEFAULT_ADS_TIMEOUT;
END_VAR
Name | Type | Description |
---|---|---|
nDevId | UDINT | The device ID of a NOV/DPRAM card. The ID is used to specify the card from which information is to be read. The device IDs are specified by the TwinCAT system during the hardware configuration. |
bExecute | BOOL | The function block is activated on a positive edge at this input. |
tTimeOut | TIME | Specifies the time-out period that must not be exceeded when executing the command/function. |
Outputs
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
nErrId : UDINT;
stInfo : ST_NovRamAddrInfo;
END_VAR
Name | Type | Description |
---|---|---|
bBusy | BOOL | This output is set when the function block is activated, and remains set until execution has been completed. |
bError | BOOL | This output is set if an error occurs during execution. |
nErrId | UDINT | Returns the ADS error number when the bError output is set. |
stInfo | A structure with the address and size of the NOV/DP-RAM. |
Sample:
A pointer to an instance of the ST_NOVRAM structure variable is declared in MAIN. This pointer is initialized with the address of the NOV/DP-RAM at the start of the program. By referencing the pointer, the individual structure elements (and the NOV/DP-RAM) can be accessed for writing or reading.
TYPE ST_NOVRAM :
STRUCT
dwParam_0 : DWORD;
dwParam_1 : DWORD;
dwParam_2 : DWORD;
dwParam_3 : DWORD;
cbSize : DWORD;
wCounter : WORD;
func : BYTE;
sMsg : STRING(20);
(* ...other NOV-/DP-RAM variables *)
END_STRUCT
END_TYPE
PROGRAM MAIN
VAR
pNOVRAM : POINTER TO ST_NOVRAM;
cbNOVRAM : DWORD;
fbGetInfo : FB_GetDPRAMInfo;
state : INT := 0;
bInit : BOOL := FALSE;
timer : TON;
bReset : BOOL;
END_VAR
CASE state OF
0:
IF NOT bInit THEN
state := 1;
END_IF
1:
fbGetInfo( bExecute := FALSE ); (* reset fb *)
fbGetInfo( nDevId:= 3,
bExecute:= TRUE, (* start fb execution *)
tTimeOut:= T#10s );
state := 2;
2:
fbGetInfo( bExecute := FALSE );
IF NOT fbGetInfo.bBusy THEN
IF NOT fbGetInfo.bError THEN
IF fbGetInfo.stInfo.pCardAddress <> 0 THEN
pNOVRAM := fbGetInfo.stInfo.pCardAddress;
cbNOVRAM := fbGetInfo.stInfo.iCardMemSize;
bInit := TRUE;
state := 0; (* ready, go to the idle step *)
ELSE
state := 100; (* error *)
END_IF
ELSE
state := 100; (* error *)
END_IF
END_IF
100:
; (* error, stay here *)
END_CASE
IF bInit THEN
(* read from or write to NOV-/DP-RAM*)
IF bReset THEN (* reset all bytes to 0 *)
bReset := FALSE;
MEMSET( pNOVRAM, 0, cbNOVRAM );
END_IF
timer( IN := TRUE, PT := T#1s );
IF timer.Q THEN
timer( IN := FALSE );
pNOVRAM^.dwParam_0 := pNOVRAM^.dwParam_0 + 1;
pNOVRAM^.dwParam_1 := pNOVRAM^.dwParam_1 - 1;
END_IF
END_IF
Requirements
Development environment |
Target platform |
IO Hardware |
PLC libraries to be integrated (category group) |
---|---|---|---|
TwinCAT v3.1.0 |
PC or CX (x86) |
FCxxxx cards with NOVRAM (FCxxxx-0002) |
Tc2_IoFunctions (IO) |