Creating custom tools
The TwinCAT Building Automation Manager offers the possibility to create custom tools (add-ins). Add-ins have the possibility to access the internal data management of the TwinCAT Building Automation Manager via an object model. This may be a read or a write access. Hence, add-ins can be created with which documentation on the system can be automatically generated. It is also possible to create add-ins for reading files (e.g. Excel files) from which the project file is generated. Of particular importance is the possibility to generate entire operator interfaces.
The following executions show all steps necessary to create a basis for the actual add-in development. The complete Microsoft Visual Studio 2010 project is available in the download area at the foot of the page.
Requirements
- Microsoft Visual Studio 2010 (or higher)
- Microsoft .NET Framework 4.0 (or higher)
- Programming skills in C#
Creating a project
Start Microsoft Visual Studio 2010 and create a new project. The project must be of the type Class Library.
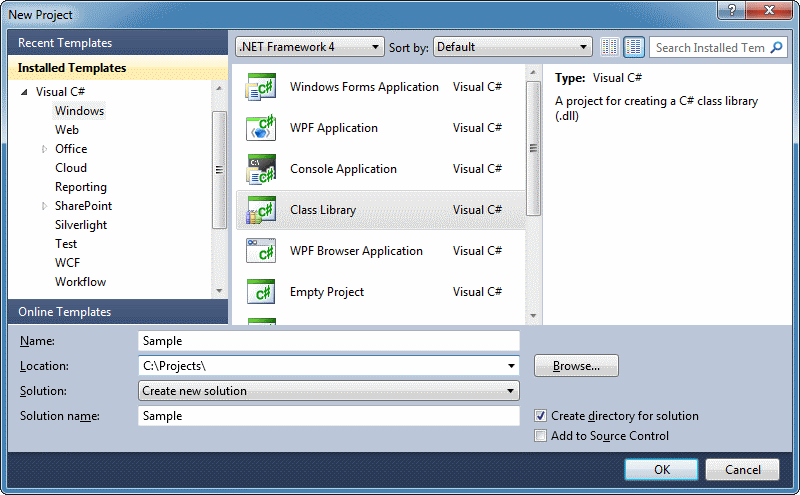
Remove the Class1.cs file created as standard in the Solution Explorer and add a new Windows form to the project. Mark the newly added Form1.cs file and open the code view. The source text which appears should now look as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Sample
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
}
}
Adding references
The next step covers the adding of three references.
- System.ComponentModel.Composition: provides the necessary attributes ExportMetadata and Export.
- TcBAManager.AddIn: offers access to classes that are necessary for working with the TwinCAT Building Automation Manager.
- TcBAManager.AddIn.Contract: interfaces and enumeration types which the add-in makes available to the TwinCAT Building Automation Manager.
Right-click in the Solution Explorer on References and select Add Reference…. In the window which then opens, select Assemblies on the left-hand side and then look for the reference System.ComponentModel.Composition. A double-click adds it to the project.
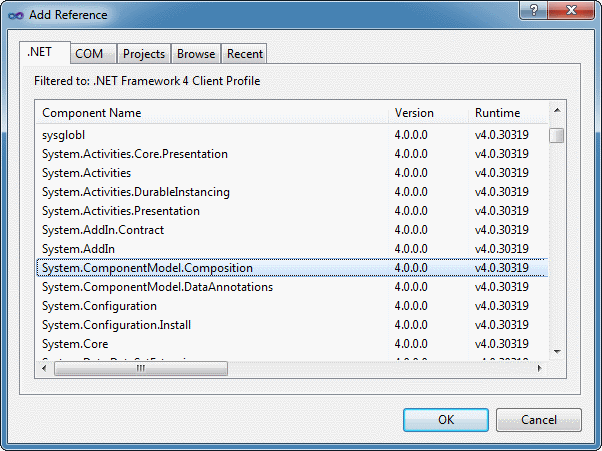
The references from the TwinCAT Building Automation Manager follow next. To this end press the Browse button and navigate to the TwinCAT Building Automation Manager folder (standard is C:\TwinCAT\BAFrameworkV2.20\BAManager). TcBAManager.AddIn.dll and TcBAManager.AddIn.Contract.dll are located there. Add both to the project. The window can now be closed.
Placing at disposal
So that the add-in is available in the TwinCAT Building Automation Manager and can be correctly displayed, a couple of extensions are necessary in the source text of the Form1.cs file.
Begin with the extension of the namespaces. System.ComponentModel.Composition, TwinCAT.BAManager.AddIns.Model and TwinCAT.BAManager.AddIns.Contract are added.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.ComponentModel.Composition;
using TwinCAT.BAManager.AddIns.Model;
using TwinCAT.BAManager.AddIns.Contract;
namespace Sample
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
}
}
Now extend the constructor by the interfaces IAddInContractV1 and IDisposable. The attributes via which the additional information within the TwinCAT Building Automation Manager is made available follow.
These include the display name and the description in the respective language, the version number and the type of add-in. This specifies the place of display of the add-in in the menu item Tools within the TwinCAT Building Automation Manager.
The specification next the Export attribute enables the TwinCAT Building Automation Manager to identify valid add-ins.
Now the interface IAddInContractV1 needs to be implemented and the global variable of the class Project created and initialised.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.ComponentModel.Composition;
using TwinCAT.BAManager.AddIns.Model;
using TwinCAT.BAManager.AddIns.Contract;
namespace Sample
{
[ExportMetadata("Name1031", "Beispiel")]
[ExportMetadata("Name1033", "Sample")]
[ExportMetadata("Description1031", "Ein Beispielprogramm.")]
[ExportMetadata("Description1033", "A sample program.")]
[ExportMetadata("Version", "V1.0.0")]
[ExportMetadata("Target", AddInTarget.Extension)]
[Export(typeof(IAddInContractV1))]
public partial class Form1 : Form, IAddInContractV1, IDisposable
{
private Project project;
public Form1()
{
InitializeComponent();
project = Project.Instance;
}
public Form AddInUserControl
{
get
{
return this;
}
}
}
}
The instance of the Project class now offers access to all functions and information provided by the TwinCAT Building Automation Manager.
Since this project concerns a class library, you must first define an external program in the project properties with which the add-in is to be started in order to start and debug. To do this, open the Solution Explorer and call the Properties by right-clicking on the project. In the Debug menu item, enter the path to the TwinCAT Building Automation Manager next to Start external program.
Select the Build menu item and, in the Output area, enter the path to the AddIn folder of the TwinCAT Building Automation Manager (standard is C:\TwinCAT\BAFrameworkV2.20\BAManager\AddIns). The properties can now be closed.
Finally, steps must be taken to prevent the entire TwinCAT Building Automation Manager from being copied to the AddIn folder when compiling. To do this, open the Solution Explorer and expand the References folder. Right-click to open the properties of the TcBAManager.AddIn reference and set Copy Local to false. Repeat this procedure for the TcBAManager.AddIn.Contract reference.
Information and functions of the interface
The properties are used for querying or setting simple object information, such as name or comment. More complex information, such as links or occupied terminal channels, is available as an XML document and can be queried with the GetParameters() method or set with SetParameters(). The structure for the setting of parameters thereby corresponds precisely to the delivered structure of GetParameters().
Furthermore, messages can be deposited in the message window of the TwinCAT Building Automation Manager.
The attached project, AddIns.Test, in the download area provides a detailed insight into the functions and structure.
Application snippets
The project from the previous steps serves as the basis for the following snippets.
Snippet 1 - Reading a scene
Using the TwinCAT Building Automation Manager, create a new project and add to it any controller with at least four scenes and lamps. The first scene receives a command that switches on the first lamp. Repeat this procedure for the other scenes.
foreach (Controller controller in project.Controllers)
{
var scenes = from scene in controller.Scenes
where (scene.Id % 2) == 0
select scene;
Debug.WriteLine("Controller {0}: {1} ", controller.Id, controller.Name);
foreach (Scene scene in scenes)
{
XmlDocument doc = scene.GetParameters();
Debug.WriteLine(" Scene {0}: {1}", scene.Id, scene.Name);
}
}
After calling the add-in, all scenes of the controller whose ID is divisible by two are transferred to a new list and afterwards output in the debug console.
The GetParameters( ) method delivers the scene contents in the following XML structure:
‹?xml version="1.0" encoding="utf-16" ?›
‹Scene Id="2"›
‹ExternalImplementation›false‹/ExternalImplementation›
‹SumUpScenes›false‹/SumUpScenes›
‹SceneItem›
‹EntityControllerId›1‹/EntityControllerId›
‹EntityType›ActuatorLampBase‹/EntityType›
‹EntityId›2‹/EntityId›
‹EntityCommandId›3‹/EntityCommandId›
‹/SceneItem›
‹/Scene›
Snippet 2 - Creating a project
project.CreateNew("Sample");
Controller controllerCX1010 = project.Controllers.Add(5);
controllerCX1010.Name = "My CX1010";
FieldBusDevice fieldBusDeviceCX1010 = controllerCX1010.FieldBusDevices.Add(1);
FieldBusCoupler fieldBusCouplerCX1010 = fieldBusDeviceCX1010.FieldBusCouplers.Add(4);
Terminal terminalKL2012 = fieldBusCouplerCX1010.Terminals.Add(project.TerminalDescriptions["2012_0"]);
terminalKL2012.Name = "My KL2012";
Lamp lampType1 = controllerCX1010.Lamps.Add(1);
lampType1.Name = "My Lamp";
XmlDocument doc = new XmlDocument();
doc.LoadXml(@"
‹Lamp›
‹Link›
‹LinkChannelId›0‹/LinkChannelId›
‹BusDeviceId›1‹/BusDeviceId›
‹BusCouplerId›1‹/BusCouplerId›
‹TerminalId›1‹/TerminalId›
‹ChannelId›1‹/ChannelId›
‹ControllerId›1‹/ControllerId›
‹/Link›
‹/Lamp›
");
lampType1.SetParameters(doc);
Here a new project is created with controller, fieldbus device, fieldbus coupler, terminal and lamp. After creation of the parameters for linking the lamp to the first terminal channel, the XML structure is transferred to the lamp with the SetParameters( ) method.
The AddIns.Test project in the download area provides a detailed insight into the XML structure of the respective elements.
Close the add-in and the add-in selection dialogue. The newly-created project now appears in the navigation tree.
Download area
Download area
Project template for the development of add-ins.
Project that demonstrates the functions and structure of the interface.