ASP.NET 2.0 AJAX
Microsoft ASP.NET 2.0 AJAX Extensions is the new name for the ASP.NET technology code-named "Atlas".
System Requirements
- Supported Operating Systems: Windows Server 2003; Windows Vista; Windows XP
- Required Software:
- Microsoft .NET Framework Version 2.0
Link: http://msdn.microsoft.com/netframework/downloads/updates/default.aspx - Microsoft Internet Explorer 5.01 (or later)
- Visual Studio 2005
- Framework "ASP.NET 2.0 AJAX Extensions" (free)
Link : http://ajax.asp.net/ - Microsoft ASP.NET AJAX
Get Started with ASP.NET 2.0 AJAX in VS2005?
Step 1. Create a new project
Open Visual Studio 2005, choose under File , New the pption Website... Now you create a new website.
Choose under the "Templates" the "ASP.NET AJAX-Enabled Web Site" template.
Choose the source code language: Visual C#
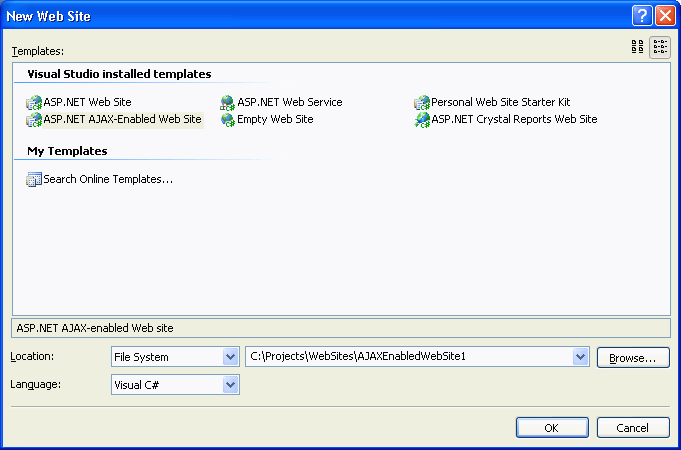
Step 2. Add the reference:
Add the reference of TwinCAT.Ads.dll to VS2005 -> Menu -> Website -> Add Reference....
The default installation path for .NET Framework 2.0 of TwinCAT.Ads.dll is C:\TwinCAT\ADS Api\.NET\v2.0.50727\TwinCAT.ADS.dll
Please work with the latest greatest Version.
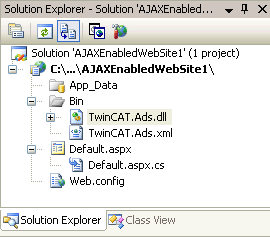
Step 3. Add AJAX component:
Add the Timer and the UpdatePanel of AJAX Extensions to the web file "Default.aspx" from Toolbox of Visual Studio 2005.
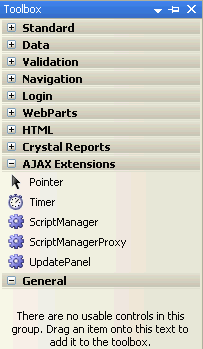
4. Edit properties:
Change Interval of the Timer1:
Interval = "3000"
Change the option UpdateMode of the UpdatePanel1:
UpdateMode = "Conditional"
5. Read PLC variables:
Use the TcAdsClient.Read() to read the variables from ADS device. Reads data synchronously from an ADS device and writes it to the given stream.
TcAdsClient.Read(int indexGroup, int indexOffset, AdsStream dataStream, int offset, int length);
Parameters:
- indexGroup: - Contains the index group number of the requested ADS service.
- indexOffset: - Contains the index offset number of the requested ADS service.
- dataStream: - Stream that receives the data.
- offset: - Offset of the data in dataStream.
- length: - Length of the data in dataStream.
Step 6. ASP.NET 2.0 AJAX Sample 01
Default.aspx
<%@ Page Language="C#"AutoEventWireup="true"CodeFile="Default.aspx.cs"Inherits="_Default"%>
<!DOCTYPE html PUBLIC"-//W3C//DTD XHTML 1.1//EN""http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ASP.NET 2.0 AJAX Sample 01</title>
</head>
<body>
<form id="form1"method="post"runat="server">
<div>
<asp:ScriptManager ID="ScriptManager1"runat="server" />
<asp:Timer ID="Timer1"runat="server"Interval="3000"OnTick="Timer1_Tick">
</asp:Timer>
<!-- ASP.NET AJAX UpdatePanel1 - Only the panel contet is updated. -->
<asp:UpdatePanel ID="UpdatePanel1"runat="server"UpdateMode="Conditional">
<ContentTemplate>
UpdatePanel content refreshed at <%=DateTime.Now.ToString() %>
<asp:Label ID="lblPlcVarBool01"runat="server"Text="PlcVarBool:"Style="z-index: 101; left: 10px; position: absolute; top: 50px"></asp:Label>
<asp:Label ID="lblPlcVarBool02"runat="server"Text="-"BackColor="Black"ForeColor="White"Style="z-index: 101; left: 100px; position: absolute; top: 50px"></asp:Label>
<asp:Label ID="lblPlcVarInt01"runat="server"Text="PlcVarInt:"Style="z-index: 101; left: 10px; position: absolute; top: 100px"></asp:Label>
<asp:Label ID="lblPlcVarInt02"runat="server"Text="-"BackColor="Black"ForeColor="White"Style="z-index: 101; left: 100px; position: absolute; top: 100px"></asp:Label>
<asp:Label ID="lblMessage01"runat="server"Text="Message:"Style="z-index: 101; left: 10px; position: absolute; top: 200px"></asp:Label>
<asp:Label ID="lblMessage02"runat="server"Text="-"Style="z-index: 101; left: 100px; position: absolute; top: 200px"></asp:Label>
<asp:Button ID="btnRefreshAll"runat="server"Text="Refresh All"Width="160px"OnClick="btnRefreshAll_Click"Style="z-index: 201; left: 207px; position: absolute; top: 250px"/>
<asp:Label ID="lblPlcVarString01"runat="server"Text="PlcVarString:"Style="z-index: 101; left: 10px; position: absolute; top: 150px"></asp:Label>
<asp:Label ID="lblPlcVarString02"runat="server"Text="-"BackColor="Black"ForeColor="White"Style="z-index: 101; left: 100px; position: absolute; top: 150px"></asp:Label>
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Timer1" />
</Triggers>
</asp:UpdatePanel>
</div>
</form>
</body>
</html>
Default.aspx.cs
![]() | Change the AMS NET ID and AdsServerPort settings You must change the AMS NET ID and AdsServerPort to your local project settings. |
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Drawing;
using TwinCAT.Ads;
using System.IO;
public partial class_Default : System.Web.UI.Page
{
// ADS Kommunication with ADS deviceTcAdsClient tcAds = newTcAdsClient();
// AMS NET ID <- Change this AMS NET ID for your TwinCAT Configurationstring strAmsNetId = "192.168.0.2.1.1";
// AMS Port (801, 811, 821, 831) <- Change this AMS Port for your TwinCAT Configurationint iAdsServerPort = 801;
protected void Page_Load(object sender, EventArgs e)
{
}
public void RemoteStatusRead()
{
try
{
tcAds.Connect(strAmsNetId, iAdsServerPort);
tcAds.Timeout = 200;
AdsStream dataStream = newAdsStream(171);
BinaryReader binRead = newBinaryReader(dataStream);
// read bool variable with IndexGroup and IndexOffset
tcAds.Read(16417, 0, dataStream, 0, 1);
// read int variable with IndexGroup and IndexOffset
tcAds.Read(16416, 1, dataStream, 1, 2);
// read string variable with IndexGroup and IndexOffset
tcAds.Read(16416, 5, dataStream, 3, 168);
bool bVarBool = false;
byte b = binRead.ReadByte();
if (b == 0) bVarBool = false;
else bVarBool = true;
if (bVarBool == true)
{
lblPlcVarBool02.Text = "TRUE";
lblPlcVarBool02.BackColor = Color.Blue;
lblPlcVarBool02.ForeColor = Color.White;
}
else
{
lblPlcVarBool02.Text = "FALSE";
lblPlcVarBool02.BackColor = Color.Black;
lblPlcVarBool02.ForeColor = Color.White;
}
int iData = 0;
iData = binRead.ReadByte();
if (iData != 0)
{
lblPlcVarInt02.Text = iData.ToString();
}
else
{
lblPlcVarInt02.Text = "-";
}
char [] strData = new char[160];
strData = binRead.ReadChars(160);
if (strData != null)
{
lblPlcVarString02.Text = "";
for (int i = 1; i < 160; i++)
{
lblPlcVarString02.Text += strData[i];
}
}
else
{
lblPlcVarString02.Text = "-";
}
lblMessage02.Text = "";
}
catch(Exception ex)
{
lblMessage02.Text = ex.Message;
}
}
protected void btnRefreshAll_Click(object sender, EventArgs e)
{
RemoteStatusRead();
// update content of the UpdatePanel1
UpdatePanel1.Update();
}
protected void Timer1_Tick(object sender, EventArgs e)
{
RemoteStatusRead();
// update content of the UpdatePanel1
UpdatePanel1.Update();
}
}
Step 7. PLC Sample
PROGRAM MAIN
VAR
PlcVarBool AT%MX0.0 : BOOL := TRUE;
PlcVarInt AT%MW1 : INT := 0;
PlcVarIntMax AT%MW3 : INT := 1000;
PlcVarString AT%MD5 : STRING(20);
TON1: TON;
bool1: BOOL;
time1: TIME;
END_VAR
PlcVarInt := PlcVarInt + 1;
IF PlcVarInt > PlcVarIntMax THEN
PlcVarInt := 0;
PlcVarBool := NOT PlcVarBool;
END_IF
TON1(IN:=TRUE , PT:=t#5m , Q=>bool1 , ET=>time1 );
IF bool1 THEN
TON1(IN:=FALSE , PT:= , Q=> , ET=> );
END_IF
PlcVarString := TIME_TO_STRING(time1);
Step 9. Configuration of the Microsoft Internet Information Services (IIS)
Open the IIS:
- Start -> Control Panel -> Administrative Tools -> Internet Information Services
- Add a new virtual Directory for a new website: IIS -> (local computer) -> Web Sites -> Default Web Sites -> New -> Virtual Directory...
Settings for IIS:
- Alias: AjaxSample01
- Directory: C:\Projects\WebSites\AJAXEnabledWebSite1
- Access Permisions: Choose "Read", "Run scripts" and "Execute"
- Important: Click to Node "AjaxSample01" and change the IIS Properties "ASP.NET" of the Web site to Version 2.0
Step 10. Demo of the AJAX Website Sample Microsoft IE
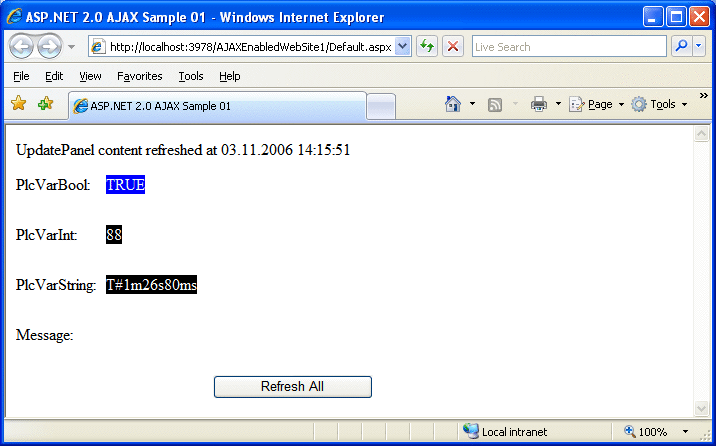
Browse to the website with the "Default.aspx" in IIS
or open the link http://localhost/AjaxSample01/Default.aspx with Internet Explorer
(p.E. http://192.168.0.2/AjaxSample01/Default.aspx or http://<ip-of-PC>/AjaxSample01/Default.aspx).
![]() | This example can be used only with access by a user with an instance. |