Event driven reading
Task
There are 7 global variables in the PLC. Each of these PLC variables is of a different data type. The values of the variables should be read in the most effective manner, and the value with its timestamp is to be displayed on a form in Visual Basic.
Description
In the form's load event, a connection to each of the PLC variables is created with the AdsReadVarConnectEx() method. The handle of this connection is stored in a global array.
The second parameter of the AdsReadVarConnectEx() method specifies the type of data exchange. ADSTRANS_SERVERONCHA has been selected here. This means that the value of the PLC variable is only transmitted if its value within the PLC has changed (see the ADSOCXTRANSMODE data type). The third parameter indicates that the PLC is to check whether the corresponding variable has changed every 100 ms.
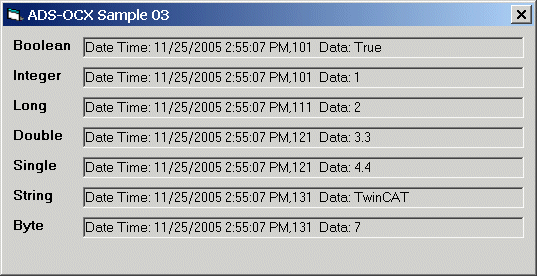
When the PLC variable changes, the AdsReadConnectUpdateEx() event is called. The timestamp, the handle, the value and a reference to the control in which the value is to be displayed are passed as parameters.
In the Unload event, the connections are released again with the method AdsDisconnectEx(). You should pay attention to this, because every connection made with AdsReadVarConnectEx() consumes resources.
Also, set the CycleTime to reasonable values, since too many read/write operations can load the system so much that the user interface slows down considerably.
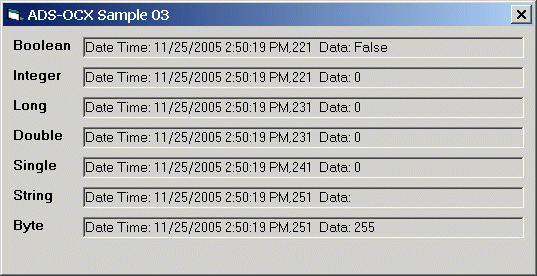
Visual Basic 6 program
Option Explicit
Dim hConnect(0 to 6) As Long
Private Sub Form_Load()
Dim nErr As Long
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarBoolean", ADSTRANS_SERVERONCHA, 100, hConnect(0), lblBoolean)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarBoolean: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarInteger", ADSTRANS_SERVERONCHA, 100, hConnect(1), lblInteger)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarInteger: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarLong", ADSTRANS_SERVERONCHA, 100, hConnect(2), lblLong)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarLong: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarDouble", ADSTRANS_SERVERONCHA, 100, hConnect(3), lblDouble)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarDouble: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarSingle", ADSTRANS_SERVERONCHA, 100, hConnect(4), lblSingle)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarSingle: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarString", ADSTRANS_SERVERONCHA, 100, hConnect(5), lblString)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarString: " & nErr)
nErr = AdsOcx1.AdsReadVarConnectEx(".PLCVarByte", ADSTRANS_SERVERONCHA, 100, hConnect(6), lblByte)
If (nErr > 0) Then Call MsgBox("Error AdsReadVarConnectEx -> .PLCVarByte: " & nErr)
End Sub
Private Sub AdsOcx1_AdsReadConnectUpdateEx(ByVal dateTime As Date,
ByVal nMs As Long,
ByVal hConnect As Long,
ByVal data As Variant,
Optional ByVal hUser As Variant)
hUser.Caption = ("Date Time: " & dateTime & "," & nMs & " Data: " & data)
End Sub
Private Sub Form_Unload(Cancel As Integer)
Dim nIndex As Long
For nIndex = 0 To 6
Call AdsOcx1.AdsDisconnectEx(hConnect(nIndex))
Next
End Sub
PLC program
VAR_GLOBAL
PLCVarBoolean : BOOL;
PLCVarInteger : INT;
PLCVarLong : DINT;
PLCVarDouble : LREAL;
PLCVarSingle : REAL;
PLCVarString : STRING(10);
PLCVarByte : BYTE;
END_VAR
PROGRAM MAIN
VAR
;
END_VAR
Language / IDE | Unpack sample program |
---|---|
Visual Basic 6 |