Reading and writing of TIME/DATE variables
Download
Language / IDE | Unpack the example program |
---|---|
Visual C# | |
Visual Basic (for .NET Framework) | |
Delphi Prism (Embarcadero Prism XE2, Oxygene for .NET) | |
Delphi for .NET (Borland Developer Studio 2006) |
Task
A .Net application should read and write a date and a time.
Description
The PLC contains the TIME variable MAIN.Time1 and the DT variable MAIN.Date1.
In the Form1_Load event method a new instance of the class TcAdsClient is created. Then the method TcAdsClient.Connect of the TcAdsClient object is called to establish a connection to the port 801. Finally the method TcAdsClient.CreateVariableHandle is used to fetch the handle of the PLC variables. When the program finishes, the Dispose method of the TcAdsClient object is called.
When the user clicks the "Read" button on the form, the variables MAIN.Time1 and MAIN.Date1 are read by means of the TcAdsClient.Read method. The class AdsBinaryReader, that derives from BinaryReader, is used to read the time and date from the PLC. The method AdsBinaryReader.ReadPlcTIME reads the time from the AdsStream and converts it to the .NET type TimeSpan. The method AdsBinaryReader.ReadPlcDATE reads the date from the AdsStream and converts it to the .NET type DateTime.
When the user clicks the "Write" button ont the form, the variables MAIN.Time1 and MAIN.Date1 are wirtten to the PLC. The class AdsBinaryWriter, that derives from BinaryWriter, is used to write the time and date to the PLC. The method AdsBinaryWriter.WritePlcType writes the .NET types TimeSpan and DateTime in the PLC format for time and date types to the AdsStream.
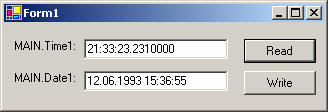
C# program
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using TwinCAT.Ads;
using System.IO;
namespace Sample04
{
public class Form1 : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Button btnRead;
private System.Windows.Forms.Button btnWrite;
private System.Windows.Forms.Label label1;
private TcAdsClient adsClient;
private int[] varHandles;
public Form1()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
...
}
private void InitializeComponent()
{
...
}
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
try
{
adsClient = new TcAdsClient();
// PLC1 Port: TwinCAT2=801, TwinCAT3=851
adsClient.Connect(801);
varHandles = new int[2];
varHandles[0] = adsClient.CreateVariableHandle("MAIN.Time1");
varHandles[1] = adsClient.CreateVariableHandle("MAIN.Date1");
}
catch( Exception err)
{
MessageBox.Show(err.Message);
}
}
private void Form1_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
adsClient.Dispose();
}
private void btnRead_Click(object sender, System.EventArgs e)
{
try
{
AdsStream adsStream = new AdsStream(4);
AdsBinaryReader reader = new AdsBinaryReader(adsStream);
adsClient.Read(varHandles[0], adsStream);
textBox1.Text = reader.ReadPlcTIME().ToString();
adsStream.Position = 0;
adsClient.Read(varHandles[1], adsStream);
textBox2.Text = reader.ReadPlcDATE().ToString();
}
catch(Exception err)
{
MessageBox.Show(err.Message)
}
}
private void btnWrite_Click(object sender, System.EventArgs e)
{
try
{
AdsStream adsStream = new AdsStream(4);
AdsBinaryWriter writer = new AdsBinaryWriter(adsStream);
writer.WritePlcType(TimeSpan.Parse(textBox1.Text));
adsClient.Write(varHandles[0], adsStream);
adsStream.Position = 0;
writer.WritePlcType(DateTime.Parse(textBox2.Text));
adsClient.Write(varHandles[1], adsStream)
}
catch(Exception err)
{
MessageBox.Show(err.Message);
}
}
}
}
PLC program
PROGRAM MAIN
VAR
Time1:TIME := T#21h33m23s231ms;
Date1:DT:=DT#1993-06-12-15:36:55.40;
END_VAR