Reading and writing of DATE/TIME variables with Delphi
From TwinCAT.Ads.NET version >= 1.0.0.10
Task
A .Net application should read and write a date and a time.
Description
The PLC program contains variables of following type:
- MAIN.Time1 vom Typ TIME;
- MAIN.Date1 vom Typ DATE;
- MAIN.DateTime1 vom Typ DT (DATE_AND_TIME);
- MAIN.TimeOfDay1 vom Typ TOD (TIME_OF_DAY);
In the MainForm_Load event method a new instance of the class TcAdsClient is created. Then the method TcAdsClient.Connect of the TcAdsClient object is called to establish a connection to the port 801. Finally the method TcAdsClient.CreateVariableHandle is used to fetch the handle of the PLC variables . When the program finishes, the MainForm_FormClosing event is called and the Dispose method of the TcAdsClient object is called.
When the user clicks the "Read" button on the form, the variables MAIN.Time1, MAIN.Date1 and so on are read by means of the TcAdsClient.Read method. The class AdsBinaryReader, that derives from BinaryReader, is used to read the time and date from the PLC. The method AdsBinaryReader.ReadPlcTIME reads the time from the AdsStream and converts it to the .NET type: TimeSpan. The method AdsBinaryReader.ReadPlcDATE reads the date from the AdsStream and converts it to the .NET type: DateTime.
When the user clicks the "Write" button ont the form, the variables MAIN.Time1 and MAIN.Date1 are wirtten to the PLC. The class AdsBinaryWriter, that derives from BinaryWriter, is used to write the time and date to the PLC. The method AdsBinaryWriter.WritePlcType writes the .NET types TimeSpan and DateTime in the PLC format for time and date types to the AdsStream.
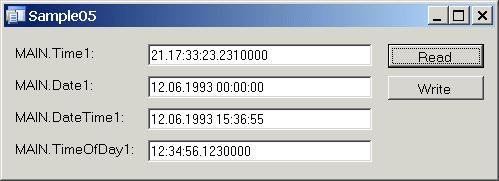
Delphi Prism (Embarcadero Prism XE2, Oxygene for .NET) program
namespace Sample05;
interface
uses
System.Drawing,
System.Collections,
System.Collections.Generic,
System.Windows.Forms,
System.ComponentModel, System.IO, TwinCAT.Ads;
type
/// <summary>
/// Summary description for MainForm.
/// </summary>
MainForm = partial class(System.Windows.Forms.Form)
private
adsClient : TcAdsClient;
varHandles : Array Of Integer;
method MainForm_Load(sender: System.Object; e: System.EventArgs);
method MainForm_FormClosing(sender: System.Object; e: System.Windows.Forms.FormClosingEventArgs);
method btnRead_Click(sender: System.Object; e: System.EventArgs);
method btnWrite_Click(sender: System.Object; e: System.EventArgs);
protected
method Dispose(disposing: Boolean); override;
public
// Time1 :TIME; Time duration, 4 byte unsigned integer, resolution in milliseconds
// Date1 :DATE; Date, 4 byte unsigned integer, resolution in seconds since 01.01.1970
// DateTime1 :DATE_AND_TIME; Date and time, 4 byte unsigned integer, resolution in seconds since 01.01.1970
// TimeOfDay1 :TIME_OF_DAY; Time of day, 4 byte unsigned integer, resolution in milliseconds
const MAX_ADSSTREAM_SIZE = 4;// SIZEOF(Time1) = SIZEOF( Date1) = SIZEOF(DateTime1) = SIZEOF(TimeOfDay1)
constructor;
end;
implementation
{$REGION Construction and Disposition}
constructor MainForm;
begin
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
end;
method MainForm.Dispose(disposing: Boolean);
begin
if disposing then begin
if assigned(components) then
components.Dispose();
//
// TODO: Add custom disposition code here
//
end;
inherited Dispose(disposing);
end;
{$ENDREGION}
method MainForm.MainForm_Load(sender: System.Object; e: System.EventArgs);
begin
try
// Set some initialization values
TextBox1.Text := new TimeSpan(0, 10, 10, 12, 345).ToString();
TextBox2.Text := new DateTime(2011, 9, 14).ToString();
TextBox3.Text := new DateTime(2011, 9, 14, 11, 6, 21).ToString();
TextBox4.Text := new TimeSpan(10, 11, 22).ToString();
// Creaste instance of class TcAdsClient
adsClient := new TcAdsClient();
// Create connectin with Port 801 on the local PC
adsClient.Connect(801);
varHandles := new Integer[4];
// Create PLC variable handles
varHandles[0] := adsClient.CreateVariableHandle('MAIN.Time1');
varHandles[1] := adsClient.CreateVariableHandle('MAIN.Date1');
varHandles[2] := adsClient.CreateVariableHandle('MAIN.DateTime1');
varHandles[3] := adsClient.CreateVariableHandle('MAIN.TimeOfDay1')
except
on err: Exception do
MessageBox.Show(err.Message, err.Source)
end;
end;
method MainForm.MainForm_FormClosing(sender: System.Object; e: System.Windows.Forms.FormClosingEventArgs);
begin
try
// Release PLC variable handles
for i:Integer := varHandles.GetLowerBound(0) to varHandles.GetUpperBound(0) do
adsClient.DeleteVariableHandle(varHandles[i]);
except
on err: Exception do
MessageBox.Show(err.Message, err.Source)
end;
// Close connection
adsClient.Dispose();
end;
method MainForm.btnRead_Click(sender: System.Object; e: System.EventArgs);
begin
try
// Create data stream instance
var dataStream := new AdsStream(MAX_ADSSTREAM_SIZE);
// Create AdsBinaryReader instance
var binRead := new AdsBinaryReader(dataStream);
// Read TIME value
dataStream.Position := 0;
adsClient.Read(varHandles[0], dataStream);
textBox1.Text := binRead.ReadPlcTIME().ToString();
// Read DATE value
dataStream.Position := 0;
adsClient.Read(varHandles[1], dataStream);
textBox2.Text := binRead.ReadPlcDATE().Date.ToString();
// Read DATE_AND_TIME value
dataStream.Position := 0;
adsClient.Read(varHandles[2], dataStream);
textBox3.Text := binRead.ReadPlcDATE().ToString();
// Read TIME_OF_DAY value
dataStream.Position := 0;
adsClient.Read(varHandles[3], dataStream);
textBox4.Text := binRead.ReadPlcTime().ToString()
except
on err: Exception do
MessageBox.Show(err.Message, err.Source)
end;
end;
method MainForm.btnWrite_Click(sender: System.Object; e: System.EventArgs);
begin
try
// Create data stream instance
var dataStream := new AdsStream(MAX_ADSSTREAM_SIZE);
// Create AdsBinaryWriter instance
var binWrite := new AdsBinaryWriter(dataStream);
// Write TIME value
dataStream.Position := 0;
binWrite.WritePlcType(TimeSpan.Parse(textBox1.Text));
adsClient.Write(varHandles[0], dataStream);
// Write DATE value
dataStream.Position := 0;
binWrite.WritePlcType(DateTime.Parse(textBox2.Text));
adsClient.Write(varHandles[1], dataStream);
// Write DATE_AND_TIME value
dataStream.Position := 0;
binWrite.WritePlcType(DateTime.Parse(textBox3.Text));
adsClient.Write(varHandles[2], dataStream);
// Write TIME_OF_DATE value
dataStream.Position := 0;
binWrite.WritePlcType(TimeSpan.Parse(textBox4.Text));
adsClient.Write(varHandles[3], dataStream)
except
on err: Exception do
MessageBox.Show(err.Message, err.Source)
end;
end;
end.
PLC program
PROGRAM MAIN
VAR
Time1 :TIME := T#521h33m23s231ms;
Date1 :DATE := D#1993-06-12;
DateTime1 :DATE_AND_TIME := DT#1993-06-12-15:36:55;
TimeOfDay1 :TIME_OF_DAY := TOD#12:34:56.123;
END_VAR
;
Download
Language / IDE | Unpack the sample program |
---|---|
Delphi Prism (Embarcadero Prism XE2, Oxygene for .NET) | |
Delphi for .NET (Borland Developer Studio 2006) |