Operating cases
The methods FB_init, FB_reinit and FB_exit are called implicitly at different times. You can find information on these different operating cases in the following:
- Operating case "First download"
- Operating case "New download"
- Operating case "Online Change"
The call behavior of the methods differs depending on the operating case. Through the querying of the method parameters "bInCopyCode" and "bInitRetains" by FB_init and FB_exit you can distinguish between the operating cases within the methods. This enables you to adapt the implementation to the respective operating case.
Operating case | Operating case | Operating case |
---|---|---|
|
|
|
Method parameters:
| Method parameters:
| Method parameters:
|
Operating case "First download"
When downloading a PLC project to a controller that is in the delivery state, the memory locations of all variables must be set to the desired initial state through initialization. In the process, the data areas of function block instances will be filled with the desired values. Through the explicit implementation of FB_init for function blocks you can purposefully influence the initialization in this situation.
By evaluating the FB_init method parameters "bInitRetains" (TRUE) and "bInCopyCode" (FALSE) you can detect this operating case unambiguously. (See also operating case "Online Change")
![]() | The FB_init method cannot be compared with the construct of a constructor, as it is known from C#, C++ or Java, since a function block in the PLC does not require a constructor to initialize its declared variables. This takes place implicitly or explicitly in the declaration lines. The resulting consequences for function blocks that extend other function blocks are described in section Behavior with derived function blocks. |
Attribute 'call_after_init' as an alternative to FB_init
Following the call of FB_init and prior to the first cycle of the tasks of a PLC project, the external initial assignments are processed within the declaration of the function block instance.
fbTimer : TON := (PT := T#500MS);
Such assignments are only executed after FB_init has been called. If TON had an FB_init method, the assigned time value of T#500MS would not be known in this method.
To control the effects of these assignments, you can assign the attribute {attribute 'call_after_init'} to a method of a function block. You must insert the attribute both above the declaration part of the corresponding method and above the declaration part of the function block body.
The method is called after the processing of the external initial assignments and prior to the start of the tasks of a PLC project and can thus react appropriately to the user's specifications (with the external initialization).
Sample
{attribute 'call_after_init'}
FUNCTION_BLOCK FB_Sample
VAR_INPUT
nInput1 : INT;
nInput2 : INT := 100;
END_VAR
METHOD FB_init : BOOL
VAR_INPUT
bInitRetains : BOOL; // if TRUE, the retain variables are initialized (warm start / cold start)
bInCopyCode : BOOL; // if TRUE, the instance afterwards gets moved into the copy code (online change)
END_VAR
{attribute 'call_after_init'}
METHOD MyCallAfterInit
PROGRAM MAIN
VAR
fbSample : FB_Sample := (nInput2 := 700);
END_VAR
During the download, the following calls are made one after the other:
1. FB_init | Initialize instance |
1. implicit initialization code (implicit zero initialization and explicit internal value initialization of the variables) nInput1 := 0; 2. explicit initialization code (initialization code explicitly defined in FB_init) | |
fbSample.FB_init(bInitRetains := TRUE, bInCopyCode := FALSE); | |
By evaluating the FB_init method parameters, you can detect this operating case unambiguously. | |
2. explicit external variable initialization via instance declaration of the function block | Processing external initial assignments |
nInput2 := 700; | |
If the input variables of the function block have been assigned values, they will be copied. The original value is retained in the case of variables to which no value has been assigned from outside. | |
3. Method declared with attribute call_after_init | Alternative initialization |
fbSample.MyCallAfterInit(); | |
In order to unambiguously detect this operating case you can copy the value of bInCopyCode beforehand into an auxiliary variable in FB_init and evaluate this auxiliary variable in the 'call_after_init' method. |
Operating case "New download"
When downloading a PLC project again, a project already existing on the controller may be replaced. Therefore the memory space for the existing function blocks must first be released in a controlled manner. You can use the method FB_exit for this. If this method has been created it will be called before the old project is removed. Subsequently, the new project will be loaded to the controller and FB_init called. In FB_exit you can, for example, set external resources (with socket or file handles) to a defined state or release dynamically allocated memory (__NEW- or in this case __DELETE-Operator).
By evaluating the FB_exit method parameter "bInCopyCode" (FALSE), you can detect this operating case unambiguously.
Operating case "Online Change"
During an Online Change, you can influence the initialization of function block instances via the methods FB_exit, FB_init and FB_reinit. As part of the Online Change, the changes made to the PLC project during offline operation are tracked in the current control system. Therefore, the "old" instances of the function blocks are replaced by their "new" siblings as smoothly as possible.
If no changes were made in the declaration part of a function block before logging into the PLC project (i.e. changes were only made in the implementation), the data areas are not replaced. Only code blocks are replaced. The methods FB_exit, FB_init and FB_reinit are not called in this case.
![]() | If you have made changes to the declaration of a function block that will lead to the copy process described above, you receive a message about "possible unintentional effects" during an Online Change. The message box Details contain a list of all instances to be copied. |
In the code of the methods FB_init and FB_exit it is possible by evaluating the parameters "bInitRetains" (FALSE) and "bInCopyCode" (TRUE) to determine whether an Online Change is currently being executed that concerns the function block instances and is shifting them to a different memory location.
During the Online Change, the following calls take place one after the other:
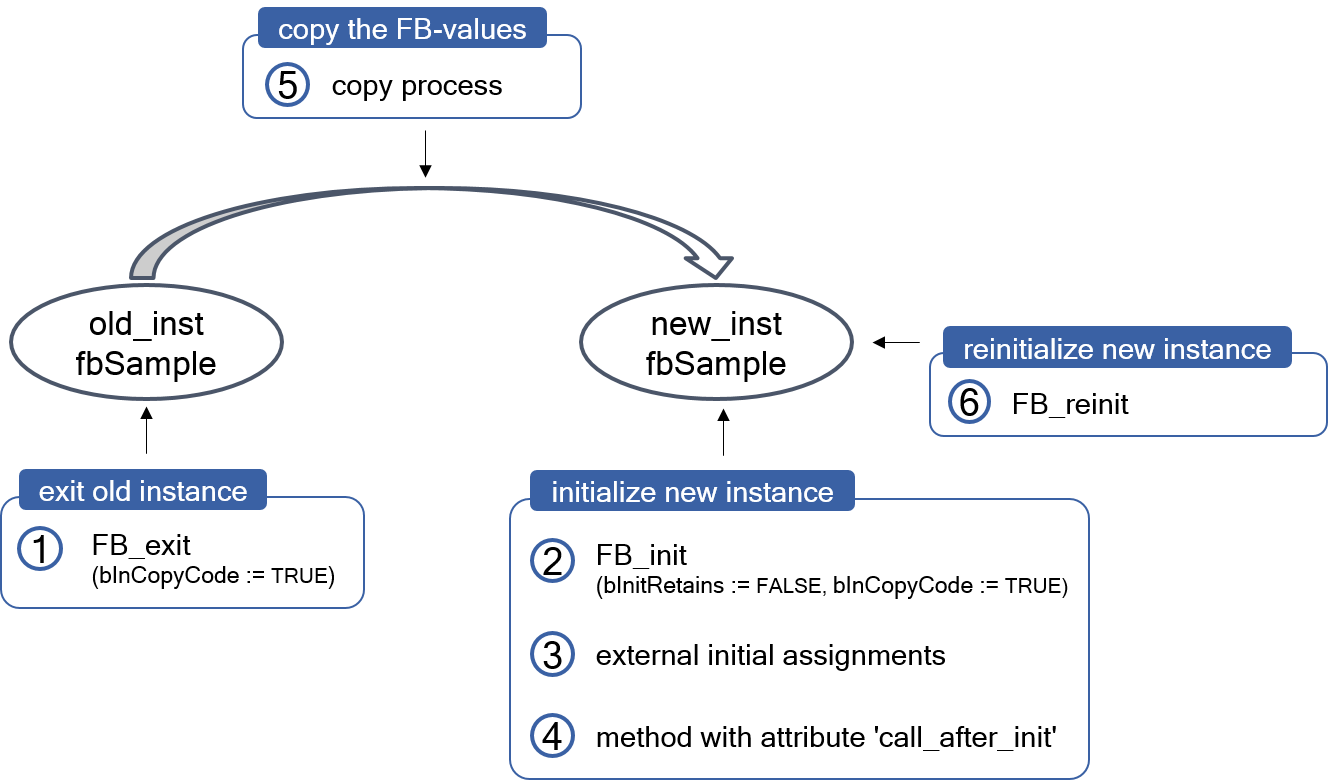
1. FB_exit | Exit old instance |
old_inst.FB_exit(bInCopyCode := TRUE); | |
By evaluating the FB_exit method parameter, you can detect this operating case unambiguously. | |
2. FB_init | Initialize new instance |
1. implicit initialization code (implicit zero initialization and explicit internal value initialization of the variables) 2. explicit initialization code (initialization code explicitly defined in FB_init) | |
new_inst.FB_init(bInitRetains := FALSE, bInCopyCode := TRUE); | |
By evaluating the FB_init method parameters, you can detect this operating case unambiguously. | |
3. explicit external variable initialization via instance declaration of the function block | Processing external initial assignments |
new_inst : <FB-Name> := (<Variable>:=<value>); | |
If the input variables of the function block have been assigned values, they will be copied. The original value is retained in the case of variables to which no value has been assigned from outside. | |
4. Method declared with attribute call_after_init | Alternative initialization |
new_inst.<Methodenname der gekennzeichneten Methode>(); | |
In order to unambiguously detect this operating case you can copy the value of bInCopyCode beforehand into an auxiliary variable in FB_init and evaluate this auxiliary variable in the 'call_after_init' method. | |
5. Copy process | Copy function block values (copy code) |
copy(&old_inst, &new_inst); | |
Existing values are retained. To this end, they are copied from the old instance to the new instance. | |
6. FB_reinit | Reinitialize new instance |
new_inst.FB_reinit(); | |
This method is called after the copy operation. It sets the variables of the instance to defined values. |
![]() | The attribute {attribute 'no_copy'} can be used to prevent a specific variable of the function block from being copied during the Online Change. In this case the variable always retains the initial value. |
See also: