SUPER
SUPER is a special variable used for object-oriented programming.
SUPER is the pointer of a function block to the basic function block instance from which the function block was created. The SUPER pointer thus also allows access to the implementation of the methods of the basic function block (basic class). A SUPER pointer is automatically available for each function block.
You can only use SUPER in method implementations and associated function block implementations.
Dereferencing the pointer: SUPER^
Using the SUPER pointer: Use the SUPER keyword to invoke the implementation of the base class or a method that is valid in the instance of the base class.
Examples:
ST:
SUPER^(); // Call of FB-body of base class
SUPER^.METH_DoIt(); // Call of method METH_DoIt that is implemented in base class
FBD/CFC/LD:
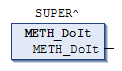
![]() | SUPER is not implemented for Instruction List (IL). |
Using the SUPER and THIS pointers:
Function block FB_Base:
FUNCTION_BLOCK FB_Base
VAR_OUTPUT
nCnt : INT;
END_VAR
Method FB_Base.METH_DoIt:
METHOD METH_DoIt : BOOL
nCnt := -1;
Method FB_Base.METH_DoAlso:
METHOD METH_DoAlso : BOOL
METH_DoAlso := TRUE;
Function block FB_1:
FUNCTION_BLOCK FB_1 EXTENDS FB_Base
VAR_OUTPUT
nBase: INT;
END_VAR
THIS^.METH_DoIt(); // Call of the methods of FB_1
THIS^.METH_DoAlso();
SUPER^.METH_DoIt(); // Call of the methods of FB_Base
SUPER^.METH_DoAlso();
nBase := SUPER^.nCnt;
Method FB_1.METH_DoIt:
METHOD METH_DoIt : BOOL
nCnt := 1111;
METH_DoIt := TRUE;
Method FB_1.METH_DoAlso:
METHOD METH_DoAlso : BOOL
nCnt := 123;
METH_DoAlso := FALSE;
Program MAIN:
PROGRAM MAIN
VAR
fbMyBase : FB_Base;
fbMyFB_1 : FB_1;
nTHIS : INT;
nBase : INT;
END_VAR
fbMyBase();
nBase := fbmyBase.nCnt;
fbMyFB_1();
nTHIS := fbMyFB_1.nCnt;
See also: