Example program R/W CoE
Program description/ function:
After starting this example program, by setting TRUE of the variable startRead or startWrite, read or write access from/to the CoE directory of a particular EtherCAT participant can be made.
Notes:
- An EtherCAT slave with CoE directory on the EtherCAT Master is present; this example is initial configured for usage of a EL3751 terminal;
configuration e.g.: IPC/CX + (EK1100) + EL3751 + EL9011 - AmsNetId of the EtherCAT Master is known (it has to be inserted in the code before starting the program and can usually be found in the EtherCAT tab of the EtherCAT master)
- The address of the EtherCAT slave have to be adapted by variable declaration of userSlaveAddr as the case may be (usually the terminal, which CoE directory have to be accessed, e.g. 1002, 1007, etc.)
- Depending on which EtherCAT slave is used, the corresponding nIndex for the CoE object ID as well as the nSubIndex for the sub index of the CoE object must be entered at the places of the value transfer for reading and writing. The correct data length and data type may also need to be adjusted.
- This example program only makes accesses to a specific value identified by the (sub) index of a CoE object. If accesses are to be made to a complete object, the corresponding FB_EcCoeSdoReadEx and FB_EcCoeSdoWriteEx function blocks must be used. See additional documentation of library: Tc2_EtherCAT:
https://infosys.beckhoff.com/
TwinCAT 3 → TE1000 XAE → PLC → Libraries → TwinCAT 3 PLC Lib: Tc2_EtherCAT
→ CoE interface
This example writes into the CoE object 0x8000 with sub index 0x16 the value 22 and activates thereby filter 1 to „IIR Butterw. LP 5th Ord. 1000 Hz“, or reads out the internal temperature value of the EL3751 terminal from CoE object 0x9000, Sub‑Index 0x01. The program variable for writing has already been assigned the value in the variable declaration. For test of writing the CoE directory can be reviewed and the variable (int16Buffer) can be watched to check reading.
Download: Programmlink
Preparations for starting the sample programs (tnzip file / TwinCAT 3)
- Click on the download button to save the Zip archive locally on your hard disk, then unzip the *.tnzip archive file in a temporary folder.
- Select the .tnzip file (sample program).
- A further selection window opens. Select the destination directory for storing the project.
- For a description of the general PLC commissioning procedure and starting the program please refer to the terminal documentation or the EtherCAT system documentation.
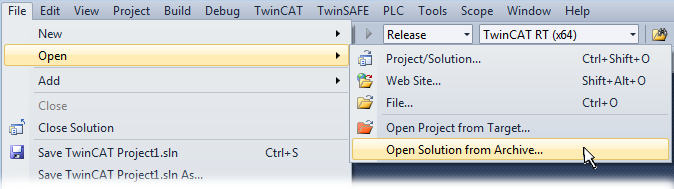
Declaration (ST)
PROGRAM MAIN
VAR
fb_coe_write : FB_EcCoESdoWrite; // Function Block for writing in CoE
fb_coe_read : FB_EcCoESdoRead; // Function Block for reading from CoE
userNetId : T_AmsNetId := 'a.b.c.d.4.1'; // Have to be entered
userSlaveAddr : UINT := 1002; // Have to be entered
startWrite : BOOL := FALSE; // Sign for start writing
startRead : BOOL := FALSE; // Sign for start reading
nState : BYTE := 0; // RW-status
// Example: Read EL3751 PAI Internal Data: Temperature Value
int16Buffer : INT; // Buffer for reading
// Example: Select EL3751 Filter1: 22 = IIR Butterw. LP 5th Ord. 1000 Hz:
uint16Buffer : UINT:=22; // Buffer for writing
bTxPDOState AT%I* : BOOL; // (PDO for synchronization)
END_VAR
Implementation (ST):
CASE nState OF
0:
IF startWrite THEN
// Prepare CoE-Access: Write value of CoE object/ sub index:
fb_coe_write(bExecute := FALSE);
nState := 1;// Next state for writing
startWrite := FALSE;
END_IF
IF startRead THEN
// Prepare CoE-Access: Read value of CoE object/ sub index:
fb_coe_read(bExecute := FALSE);
nState := 11;// Next state for reading
startRead := FALSE;
END_IF
// ============== COE WRITE ================
1:
// Write entry
fb_coe_write(
sNetId:= userNetId,
nSlaveAddr:= userSlaveAddr,
nSubIndex:= 16#16,
nIndex:= 16#8000,
pSrcBuf:= ADR(uint16Buffer),
cbBufLen:= 2,
bExecute:= TRUE,
tTimeout:= T#1S
);
nState := nState + 1; // Next state
2:
fb_coe_write(); // Execute CoE write until done
IF fb_coe_write.bError THEN
nState := 100; // Error case
ELSE
IF NOT fb_coe_write.bBusy THEN
nState := 0; // Done
END_IF
END_IF
// ============== COE READ =================
11:
// Read entry
fb_coe_read(
sNetId:= userNetId,
nSlaveAddr:= userSlaveAddr,
nSubIndex:= 1,
nIndex:= 16#9000,
pDstBuf:= ADR(int16Buffer),
cbBufLen:= 2,
bExecute:= TRUE,
tTimeout:= T#1S
);
nState := nState + 1; // Next state
12:
fb_coe_read(); // Execute CoE read until done
IF fb_coe_read.bError THEN
nState := 100; // Error case
ELSE
IF NOT fb_coe_read.bBusy THEN
nState := 0; // Done
END_IF
END_IF
100:
; // Error handling..
END_CASE
CoE_Access.zip