ModbusTCP Client
Via the ModbusTCP client (Modbus master) the BX9000 can establish a connection to a ModbusTCP server (Modbus slave). 3 function blocks are available. Fb_MBConnect for establishing a TCP/IP connection, FB_MBGenericReq for the sending and receiving of any Modbus functions, and FB_MBClose for closing the TCP/IP connection. Since FB_MBGenericReq is a generic function block, the corresponding Modbus functions have to be implemented. This enables sending of protocols that are not Modbus compliant. The maximum number of simultaneous client connections is 3. The option of closing and re-opening a TCP/IP connection means that the number of ModbusTCP servers that are accessible is almost unlimited.
FB_MBConnect
FB_MBConnect connects the BX9000 with another device via ModbusTCP. A connection is established with rising edge of bExecute. The IP address is set via sIPAddr. The TCP port number to be used is set via nTCPPort (usually set to 502 for ModbusTCP). bBusy is set as long the function block is active. If the ModbusTCP connection was initialized successfully bBusy is set to FALSE and the bError flag is FALSE. If the bError flag is TRUE be, the connection attempt has failed and the associated error code is stored in variable nErrId. nHandle must be linked to the function blocks FB_MBGernericReq and FB_MBClose.
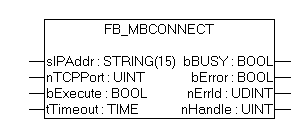
INPUT
VAR_INPUT
sIPAddr : STRING(15);
nTCPPort : UINT;
bExecute : BOOL;
tTimeout : TIME;
END_VAR
sIPAddr: IP address of the ModbusTCP-server (slave device) with which a Modbus connection is to be established.
nTCPPort: ModbusTCP port number, for ModbusTCP usually 502dec.
bExecute: A rising edge activates the function block.
tTimeout: Time after which the attempt is aborted.
OUTPUT
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
iErrId : WORD;
nHandle : UINT;
END_VAR
bBusy: This output remains TRUE until execution of the command is complete.
bError: This output is switched to TRUE as soon as an error occurs during the execution of a command. The command-specific error code is contained in iErrorId.
iErrorId: Contains the command-specific error code of the most recently executed command (see table).
nHandle: Handle that must be connected with function blocks FB_MBGernericReq and FB_MBClose.
FB_MBGENERICREQ
Function block FB_MBGENERICREQ enables sending and receiving of any ModbusTCP functions. With a rising edge of bExecute the function block becomes active, bBusy is set and the function block sends the data contained in pReqBuff (pointer to the data to be sent). The function block reads the length of the data to be sent from variable cbReqLen. The variable nHandle must be linked to the variable from the function block Fb_MBConnect nHandle. The response from the ModbusTCP server (slave) is stored in pResBuff (pointer to the receive data). The size of the variable should be adequate for receiving all data of a ModbusTCP telegram. If the ModbusClient does not receive a response within tTimeOut, bBusy is set to FALSE and bError is set. Variable nErrId contains the error code. cbResponse contains the number of received bytes. This number can be compared with the buffer size of cdResLen. If cbResponse is greater than dResLen data are lost and a larger buffer should be chosen.
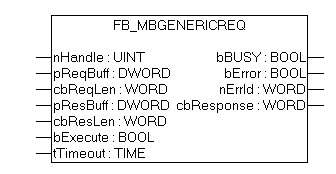
INPUT
VAR_INPUT
nHandle : UINT;
pRecBuff : DWORD;
cbReqLen : WORD;
pResBuff : DWORD;
cbResLen : WORD;
bExecute : BOOL;
tTimeout : TIME;
END_VAR
nHandle: Handle, which will be linked to the Fb_MBConnect function blocks.
pReqBuff: Pointer to the data to be sent.
cbReqLen: Length of the data to be sent.
pResBuff: Pointer to the data to be received. The size of the variable must be adequate.
cbResLen: Length of the data to be received. The length must be adequate.
bExecute: A rising edge activates the function block.
tTimeout: Time after which the attempt is aborted.
OUTPUT
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
iErrId : WORD;
cbResponse : WORD;
END_VAR
bBusy: This output remains TRUE until execution of the command is complete.
bError: This output is switched to TRUE as soon as an error occurs during the execution of a command. The command-specific error code is contained in iErrorId.
iErrorId: Contains the command-specific error code of the most recently executed command (see table).
cbResponse: Number of bytes that were received.
FB_MBCLOSE
Function block FB_MBCLOSE enables closing of a TCP/IP connection. The function block is activated with a rising edge of bExecute. The bit bBusy is set as long as the function block is busy. nHandle must be linked to the function block Fb_MBConnect nHandle. If an error occurs bError is set to TRUE. The error code can be found in nErrId. Once the function block has been called without error, Fb_MBConnect can be used to open a new socket.
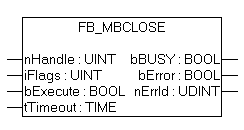
INPUT
VAR_INPUT
nHandle : UINT;
iFlags : UINT;
bExecute : BOOL;
tTimeout : TIME;
END_VAR
nHandle: Handle, which will be linked to the Fb_MBConnect function blocks.
iFlags: 0 - TCP/IP connection is closed even if the partner device cannot be reached, 1 - TCP/IP connection is terminated with "FIN"; for the function block to be able to close the TCP/IP connection without error it must exist and communication must be operational. It is recommend to use 0 because in this case the TCP/IP connection is always closed, irrespective of the state of the partner device.
bExecute: A rising edge activates the function block.
tTimeout: Time after which the attempt is aborted.
OUTPUT
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
iErrId : WORD;
END_VAR
bBusy: This output remains TRUE until execution of the command is complete.
bError: This output is switched to TRUE as soon as an error occurs during the execution of a command. The command-specific error code is contained in iErrorId.
iErrorId: Contains the command-specific error code of the most recently executed command (see table).
Example
For the example you need two BX9000 or a BC9x00 instead of a BX9000.
Download first BX9000 as ModbusTCP client (sample file)
Download second BX9000 as ModbusTCP server (or BC9x00) (sample file)
Development environment |
Target platform |
PLC libraries to be linked |
---|---|---|
TwinCAT v2.10.0 and above |
BX9000 (165) firmware version >=1.14 |
TcBaseBX9000.lbx |
Error Codes
Error code |
Description |
---|---|
0xFB00 |
No valid IP address |
0xFC00 |
Response length exceeds memory |
0xFD00 |
Request could not be sent |
0xFF00 |
Timeout during communication |
Modbus closed
Error code |
Description |
---|---|
0xFA00 |
Connection is already closed |
0xFF00 |
Timeout during closing of the connection. RST is used, but the remote device does not exist. |
Modbus client connect
Error code |
Description |
---|---|
0xFA00 |
Internal connection is refused |
0xFA01 |
Connection timeout event |
0xFA02 |
Connect was not accepted by remote |
0xFA03 |
Invalid IP address |
0xFA04 |
All Modbus connections occupied |
0xFA05 |
No further internal socket available |
0xFA06 |
Internal socket error |
0xFA07 |
Error during connect call |