Synchronisierung von Nachrichtenoperationen
TC3 IoT Functions umfasst Funktionen zur Synchronisierung mehrerer Nachrichtenoperationen. Dies kann in Szenarien, in denen Daten aus verschiedenen Datenquellen/Kanälen eingehen, sehr nützlich sein. Der Funktionsbaustein FB_IotFunctions_Request bietet verschiedene Mechanismen zur Synchronisierung von Nachrichtenoperationen.
Beispiel-Szenario
Ein Cocktailmixer stellt verschiedene Zutaten zur Verfügung, und jeder Cocktail besteht aus fünf Zutaten. Durch Drücken einer Taste wird dem Rezept eine Zutat hinzugefügt. Der Cocktailmixer beginnt mit dem Mixen des Cocktails, nachdem die fünfte Zutat ausgewählt wurde. Wenn eine Zutat ausgewählt wird, wird eine MQTT-Nachricht an ein (anderes) Topic veröffentlicht. Diese Nachrichten sollten von TC3 IoT Functions empfangen werden.
Grundlegendes Setup
TC3 IoT Data Agent ist mit einem MQTT-Gate und fünf verschiedenen Kanälen für TC3 IoT Functions konfiguriert. Jeder Kanal ist für ein "Zutaten-Topic" konfiguriert.
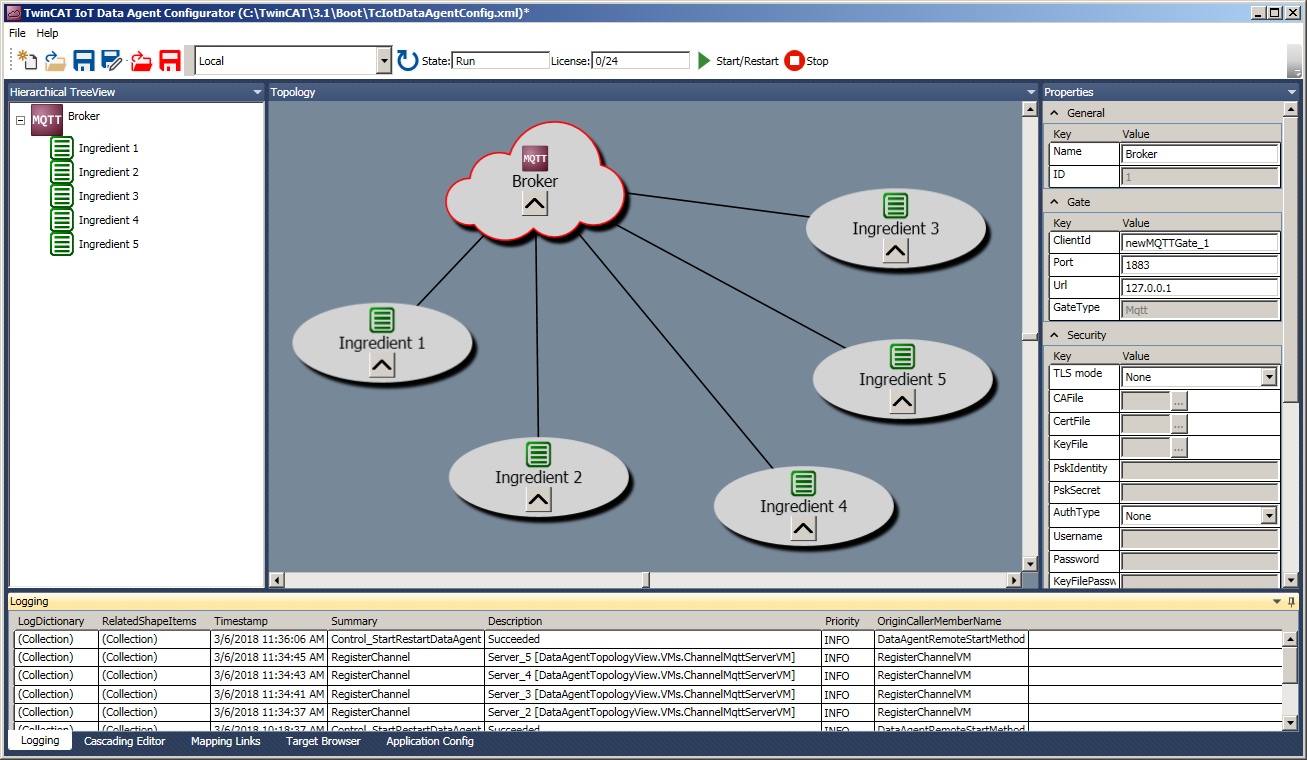
Um den Lesevorgang für fünf verschiedene Zutaten zu synchronisieren, wird eine Instanz von FB_IotFunctions_Request erstellt. Dann wird für jeden der fünf Kanäle eine Instanz von FB_IotFunctions_Message erstellt.
fbRequestIngredients : FB_IotFunctions_Request;
fbReadIngredient : ARRAY[0..4] OF FB_IotFunctions_Message := [(nChannelId := 1),(nChannelId := 2),(nChannelId := 3),(nChannelId := 4),(nChannelId := 5)];
nIn : ARRAY[0..4] OF STRING;
Die synchronisierte Anfrage kann dann wie folgt erstellt werden:
IF NOT fbRequestIngredients.bBusy THEN
IF fbRequestIngredients.bError THEN
...
ELSE
IF fbRequestIngredients.bTimeoutOccurred THEN
...
ELSE
// request was successful
...
END_IF
END_IF
// Prepare next read operation for ingredients
fbRequestIngredients.Create();
fbRequestIngredients.EnqueueRead(ADR(fbReadIngredient [0]),ADR(nIn[0]),SIZEOF(nIn[0]));
fbRequestIngredients.EnqueueRead(ADR(fbReadIngredient [1]),ADR(nIn[1]),SIZEOF(nIn[1]));
fbRequestIngredients.EnqueueRead(ADR(fbReadIngredient [2]),ADR(nIn[2]),SIZEOF(nIn[2]));
fbRequestIngredients.EnqueueRead(ADR(fbReadIngredient [3]),ADR(nIn[3]),SIZEOF(nIn[3]));
fbRequestIngredients.EnqueueRead(ADR(fbReadIngredient [4]),ADR(nIn[4]),SIZEOF(nIn[4]));
fbRequestIngredients.Execute();
END_IF
Sample04 zeigt den vollständigen Beispielcode (siehe Beispiele).
Synchronisationsbedingungen
Die Funktionsbausteininstanz fbRequest enthält verschiedene Synchronisationsbedingungen. Diese können verwendet werden, um festzustellen, ob die Lesevorgänge innerhalb der Anfrage erfolgreich waren, einen Fehler verursachten oder zu einem Timeout führten. Beachten Sie, wie die verschiedenen Timeout- und Retry-Einstellungen zur Unterstützung dieses Anwendungsfalls beitragen können (siehe Timeout Einstellungen).
Bedingung | Beschreibung |
---|---|
bBusy | TRUE: Die Anfrage ist noch in Bearbeitung und nicht alle Vorgänge wurden erfolgreich abgeschlossen FALSE: Die Anfrage wurde beendet. Um herauszufinden, ob ein Fehler oder eine Zeitüberschreitung aufgetreten ist, müssen weitere Flags ausgewertet werden. |
bTimeoutOccurred | TRUE: RequestTimeout wurde für mindestens einen Vorgang ausgelöst. FALSE: Es ist kein Timeout aufgetreten. |
bError | TRUE: Bei mindestens einem Vorgang ist ein Fehler aufgetreten. FALSE: Es ist kein Fehler aufgetreten. |
Flags der einzelnen Nachrichtenoperationen | Darüber hinaus können die Flags (error, success, bDataAvailable, bDataChanged) jeder Nachrichtenoperation analysiert werden, um herauszufinden, ob eine Anfrageoperation erfolgreich war oder nicht. |