Reading and writing of string variables
Download
Language / IDE | Unpack the sample program |
---|---|
Visual C# | |
Visual Basic (for .NET Framework) | Sample04.exe |
Delphi Prism (Embarcadero Prism XE2, Oxygene for .NET) | |
Delphi for .NET (Borland Developer Studio 2006) |
Task
A .Net application should read a string from the PLC and write a string to the PLC.
Description
The PLC contains the string MAIN.text.
In the Form1_Load event method a new instance of the class TcAdsClient is created. Then the method TcAdsClient.Connect of the TcAdsClient object is called to establish a connection to the port 801. Finally the method TcAdsClient.CreateVariableHandle is used to fetch the handle of the PLC variable. When the program finishes, the Dispose method of the TcAdsClient object is called.
When the user clicks the "Read" button on the form, the string is read by means of the TcAdsClient.Read method and is displayed in the text box. When the user clicks the "Write" button on the form, the string is wirtten to PLC and is displayed in the text box.
TwinCAT.Ads.NET version < 1.0.0.10:
The length of the AdsStream must be equal to size of the string in the PLC plus 1 (inclusive the terminating zero character). The parameter System.Text.Encoding.ASCII has to be passed to constructor of the BinaryReader class. This is necessary for the BinaryReader to be able to convert the ANSI characters to the char type.
TwinCAT.Ads.NET version >= 1.0.0.10:
The classes AdsBinaryReader and AdsBinaryWriter can be used to read and write strings ( see commented section in sample program ). These classes derive from the BinaryReader/Writer classes. To read a string from the stream one must call the method AdsBinaryReader.ReadPlcString. To write a string to the stream one must call the method AdsBinaryWriter.WritePLCString. The length of the AdsStream must be equal to size of the string in the PLC (without terminating zero character). The length is passed to this method and have to be equal to the length of the string in the PLC (without the terminating zero character).
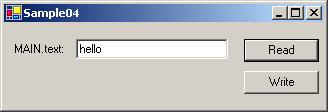
C# program
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using TwinCAT.Ads;
using System.IO;
namespace Sample04
{
public class Form1 : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Button btnRead;
private System.Windows.Forms.Button btnWrite;
private System.Windows.Forms.Label label1;
private TcAdsClient adsClient;
private int varHandle;
public Form1()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
...
}
private void InitializeComponent()
{
...
}
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
try
{
adsClient = new TcAdsClient();
// PLC1 Port: TwinCAT2=801, TwinCAT3=851
tcClient.Connect(801);
varHandle = adsClient.CreateVariableHandle("MAIN.text");
}
catch( Exception err)
{
MessageBox.Show(err.Message);
}
}
private void Form1_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
adsClient.Dispose();
}
private void btnRead_Click(object sender, System.EventArgs e)
{
try
{
//length of the stream = length of string in sps + 1
AdsStream adsStream = new AdsStream(31);
BinaryReader reader = new BinaryReader(adsStream,System.Text.Encoding.ASCII);
int length = adsClient.Read(varHandle, adsStream);
string text = new string(reader.ReadChars(length));
//necessary if you want to compare the string to other strings
//text = text.Substring(0,text.IndexOf('\0'));
textBox1.Text = text;
}
catch(Exception err)
{
MessageBox.Show(err.Message)
}
}
private void btnWrite_Click(object sender, System.EventArgs e)
{
try
{
//length of the stream = length of string + 1
AdsStream adsStream = new AdsStream(textBox1.Text.Length+1);
BinaryWriter writer = new BinaryWriter(adsStream, System.Text.Encoding.ASCII);
writer.Write(textBox1.Text.ToCharArray());
//add terminating zero
writer.Write('\0');
adsClient.Write(varHandle,adsStream);
}
catch(Exception err)
{
MessageBox.Show(err.Message);
}
}
/*From version 1.0.0.10 and higher the classes AdsBinaryReader and AdsBinaryWriter
can be used to read and write strings
private void btnRead_Click(object sender, System.EventArgs e)
{
try
{
AdsStream adsStream = new AdsStream(30);
AdsBinaryReader reader = new AdsBinaryReader(adsStream);
adsClient.Read(varHandle, adsStream);
textBox1.Text = reader.ReadPlcString(30);
}
catch(Exception err)
{
MessageBox.Show(err.Message);
}
}
private void btnWrite_Click(object sender, System.EventArgs e)
{
try
{
AdsStream adsStream = new AdsStream(30);
AdsBinaryWriter writer = new AdsBinaryWriter(adsStream);
writer.WritePlcString(textBox1.Text, 30);
adsClient.Write(varHandle, adsStream);
}
catch(Exception err)
{
MessageBox.Show(err.Message);
}
}
*/
}
}
PLC program
PROGRAM MAIN
VAR
text : STRING[30] := 'hello';
END_VAR