Reading and writing of string variables with Visual Basic
Task
A .Net application should read a string from the PLC and write a string to the PLC.
Description
The PLC contains the string: MAIN.text.
In the MainForm_Load event method a new instance of the class TcAdsClient is created. Then the method TcAdsClient.Connect of the TcAdsClient object is called to establish a connection to the port 801. Finally the method TcAdsClient.CreateVariableHandle is used to fetch the handle of the PLC variable . When the program finishes, the Dispose method of the TcAdsClient object is called. When the user clicks the "Read" button on the form, the string is read by means of the TcAdsClient.Read method and is displayed in the text box. When the user clicks the "Write" button ont the form, the string is wirtten to PLC and is displayed in the text box.
TwinCAT.Ads.NET version < 1.0.0.10:
The length of the AdsStream must be equal to size of the string in the PLC plus 1 (inclusive the terminating zero character). The parameter System.Text.Encoding.ASCII has to be passed to constructor of the BinaryReader class. This is necessary for the BinaryReader to be able to convert the ANSI characters to the char type.
TwinCAT.Ads.NET version >= 1.0.0.10:
The classes AdsBinaryReader and AdsBinaryWriter can be used to read and write strings ( see commented section in sample program ). These classes derive from the BinaryReader/Writer classes. To read a string from the stream one has to call the method AdsBinaryReader.ReadPlcString. To write a string to the stream one has to call the method AdsBinaryWriter.WritePLCString. The length of the AdsStream must be equal to size of the string in the PLC (without terminating zero character). The length is passed to these method and have to be equal to the length of the string in the PLC (without the terminating zero character).
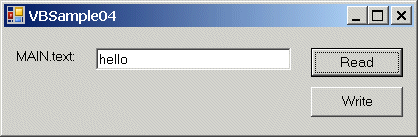
Visual Basic (for .NET framework) program
Imports TwinCAT.Ads
Imports System.IO
Public Class Form1
Inherits System.Windows.Forms.Form
Private adsClient As TcAdsClient
Private varHandle As Integer
#Region " Windows Form Designer generated code "
...
#End Region
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Try
adsClient = New TcAdsClient
adsClient.Connect(801)
varHandle = adsClient.CreateVariableHandle("MAIN.text")
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
Private Sub Form1_Closing(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles MyBase.Closing
adsClient.Dispose()
End Sub
Private Sub btnRead_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRead.Click
Dim length As Integer
Dim text As String
Dim dataStream As AdsStream
Dim reader As BinaryReader
Try
'length of the stream = length of string in sps + 1
dataStream = New AdsStream(31)
reader = New BinaryReader(dataStream, System.Text.Encoding.ASCII)
length = adsClient.Read(varHandle, dataStream)
text = New String(reader.ReadChars(length))
'necessary if you want to compare the string to other strings
'text = text.Substring(0, text.IndexOf(Chr(0)))
textBox1.Text = text
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
Private Sub btnWrite_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnWrite.Click
Dim dataStream As AdsStream
Dim writer As BinaryWriter
Dim c As Char
Try
'length of the stream = length of string + 1
dataStream = New AdsStream(textBox1.Text.Length + 1)
writer = New BinaryWriter(dataStream, System.Text.Encoding.ASCII)
writer.Write(textBox1.Text.ToCharArray())
'add terminating zero
writer.Write(Chr(0))
adsClient.Write(varHandle, dataStream)
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
End Class
PLC program
PROGRAM MAIN
VAR
text : STRING[30] := 'hello';
END_VAR
;
Requirements
Language / IDE | Unpack the sample program |
---|---|
Visual Basic (for .NET framework) | Sample04.exe |