FB_FileRingBuffer
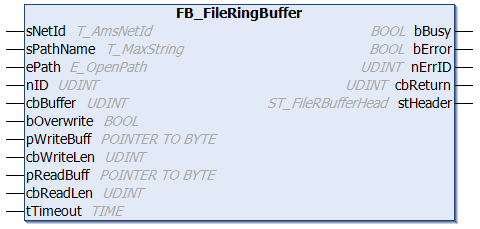
The function block FB_FileRingBuffer allows data sets of varying lengths to be written into a ring buffer file, or for data sets that have previously been written there to be removed from the ring buffer file. The written data sets are read out according to the FIFO principle in the same order in which they were previously written to the ring buffer file. This means that the oldest entries are the first ones that are read. Opening, closing, writing and reading the data sets is controlled by action calls. The function block features the following tasks:
- A_Open (Opens an existing ring buffer file for appending or generating new data sets. No error is returned if the file is already open. )
- A_Close (Closes an open ring buffer file. No error is returned if the file is already closed. )
- A_Create (Opens a new ring buffer file. If the file already exists, it is overwritten. No error is returned if the file is already open)
- A_AddTail (Writes a new data set into the ring buffer file. )
- A_GetHead (Reads the oldest data set from the ring buffer file, but does not remove it – the file pointer is not moved to the next data set. )
- A_RemoveHead (Reads and removes the oldest data set from the ring buffer file – the file pointer is moved on to the next data set.)
- A_Reset (Deletes all the data sets from an open ring buffer file. Only the file pointer and the number of data sets are reset; the existing physical file size is not changed, although the oldest data sets will be overwritten by new ones.
When a ring buffer file that already exists is opened, the file header is read first. In a ring buffer file that has previously been closed without error, bit 0 in the header status (Header.status.Bit 0) must be zero. If not, it is assumed that the file was not properly closed beforehand, and the corrupt file is replaced by a new, empty file, while Header.status.Bit 1 is set to 1 (file corrupted). When the file is closed, Header.status.Bit 0 is set to 0, and the complete file header is updated in the file.
Inputs
VAR_INPUT
sNetId : T_AmsNetId := '';
sPathName : T_MaxString := 'c:\Temp\data.dat';
ePath : E_OpenPath := PATH_GENERIC;
nID : UDINT := 0;
cbBuffer : UDINT := 16#100000;
bOverwrite : BOOL := FALSE;
pWriteBuff : POINTER TO BYTE;
cbWriteLen : UDINT;
pReadBuff : POINTER TO BYTE;
cbReadLen : UDINT;
tTimeout : TIME := DEFAULT_ADS_TIMEOUT;
END_VAR
Name | Type | Description |
---|---|---|
sNetID | T_AmsNetID | A string with the network address of the TwinCAT computer on which a directory search is to be executed can be specified here. For the local computer an empty string may be specified. |
sPathName | T_MaxString | Contains the path and file name for the buffer file to be opened (type: T_MaxString). Note: the path can only point to the local computer’s file system! This means that network paths cannot be used here! |
ePath | E_OpenPath | This input can be used to select a TwinCAT system path on the target device for opening the file. |
nID | UDINT | User-defined 32-bit value. When a new file is opened, this value is saved in the file and can be used, for instance, for checking the version of the buffer file. |
cbBuffer | UDINT | The maximum size, in bytes, of the buffer file that is to be open. This parameter is saved in the file header when the file is created, and is checked when the same file is opened again. You can only reopen files that have been created using the same maximum buffer size. You cannot, in other words, create a file with a smaller buffer size, fill it with data sets, and then open it again with a larger buffer size. If the check of the maximum buffer size fails, a new file with the new buffer size is automatically created and opened. Bit 1 (file corrupted) is also set in the file header status. |
bOverwrite | BOOL | Write behavior when the maximum file buffer size is reached. If this input is TRUE, the oldest entries are overwritten if the max. file buffer size has already been reached (entries are deleted until the free buffer size is sufficient to store the new entry). If this input is FALSE, a buffer overflow when the maximum file buffer size is reached is reported as an error. |
pWriteBuff | BYTE | The address of the PLC variable or of a buffer variable that contains the value data that is to be written. The address can be determined with the ADR operator. The programmer is himself responsible for dimensioning the buffer variable in such a way that cbWriteLen data bytes can be taken from it. |
cbWriteLen | UDINT | The number of value data bytes that are to be written (In the case of string variables this includes the final null). |
pReadBuff | BYTE | The address of the PLC variable or of a buffer variable into which the value data that has been read is to be copied. The address can be determined with the ADR operator. The programmer is himself responsible for dimensioning the buffer variable in such a way that it can accept cbReadLen data bytes. The size of the buffer variables in bytes must be greater than or equal to the size of the data set that is to be read. |
cbReadLen | UDINT | The number of value data bytes to be read. If the buffer size is too small, no data is copied, the function block reports a buffer underflow error and the required buffer size for the next data set to be read is returned at the cbReturn output. |
tTimeout | TIME | States the length of the timeout that may not be exceeded by execution of the ADS command. |
Outputs
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
nErrId : UDINT;
cbReturn : UDINT;
stHeader : ST_FileRBufferHead;
END_VAR
Name | Type | Description |
---|---|---|
bBusy | BOOL | When the function block is enabled, this output is set and remains set until a feedback is received. |
bError | BOOL | If an error occurs during the transmission of the command, this output is set after the bBusy output is reset. |
nErrId | UDINT | Returns the ADS error number when the bError output is set. |
cbReturn | UDINT | The number of value data bytes successfully read. If a read buffer underflow error has occurred, this output supplies the necessary read buffer size in bytes. |
stHeader | Ring buffer file header/status. |
Requirements
Development environment | Target platform | PLC libraries to be integrated (category group) |
---|---|---|
TwinCAT v3.1.0 | PC or CX (x86, x64, Arm®) | Tc2_Utilities (System) |