Accessing, creating and handling PLC projects
This chapter explains in-depth how to create, access and handle PLC projects. The following list shows all chapters in this article:
- General information about PLC projects
- Creating and handling PLC projects
- Opening existing PLC projects
- Nested projects and project instances
- Saving the PLC project as a library
- Handling online functionalities (Login, StartPlc, StopPlc)
- Setting Boot project options
- Saving project and/or solution as archive
- Calling CheckAllObjects()
General information about PLC projects
PLC projects are specified by their so-called project template. TwinCAT currently deploys two templates which are represented by a template file in the TwinCAT directory. The following table shows which PLC templates are available and the corresponding template file:
Template name |
Template file |
---|---|
Standard PLC template |
C:\TwinCAT\3.x\Components\Plc\PlcTemplate\Plc Templates\Standard PLC Template.plcproj |
Empty PLC template |
C:\TwinCAT\3.x\Components\Plc\PlcTemplate\Plc Templates\Empty PLC Template.plcproj |
Creating and handling PLC projects
To create a new PLC project via Automation Interface, you need to navigate to the PLC node and then execute the CreateChild() method with the corresponding template file as a parameter.
Code snippet (C#):
ITcSmTreeItem plc = systemManager.LookupTreeItem("TIPC");
ITcSmTreeItem newProject = plc.CreateChild("NameOfProject", 0, "", pathToTemplateFile);
Code snippet (Powershell):
$plc = $systemManager.LookupTreeItem("TIPC")
$newProject = $plc.CreateChild("NameOfProject", 0, "", pathToTemplateFile)
![]() | Please note When using standard PLC templates as provided by Beckhoff, please make sure to only use the template name instead of the full path, e.g. “Standard PLC Template”. |
All subsequent operations, like creating and handling POUs and filling them with code, are described in a separate article.
After the PLC project has been created, it can be further handled by casting it to the special interface ITcPlcIECProject, which provided more functionalities and access to the projects specific attributes:
Code snippet (C#):
ITcSmTreeItem plcProject = systemManager.LookupTreeItem("TIPC^NameOfProject^NameOfProject Project");
ITcPlcIECProject iecProject = (ITcPlcIECProject) plcProject;
Code snippet (Powershell):
$plcProject = $systemManager.LookupTreeItem("TIPC^NameOfProject^NameOfProject Project")
The object "iecProject" can now be used to access the methods of the ITcPlcIECProject interface, e.g. to save the PLC project as a PLC library.
Opening existing PLC projects
To open an existing PLC-Project via Automation Interface, you need to navigate to the PLC node and then execute the CreateChild() method with the path to the corresponding PLC project file file as a parameter.
You can use three different values as SubType:
- 0: Copy project to solution directory
- 1: Move project to solution directory
- 2: Use original project location (when used, please use
""
as project name parameter)
Basically, these values represent the functionalities (Yes, No, Cancel) from the following MessageBox in TwinCAT XAE:
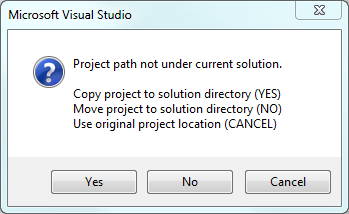
In place of the template file you need to use the path to the PLC project (to its plcproj file) that needs to be added. As an alternative, you can also use a PLC project archive (tpzip file).
Code snippet (C#):
ITcSmTreeItem plc = systemManager.LookupTreeItem("TIPC");
ITcSmTreeItem newProject = plc.CreateChild("NameOfProject", 1, "", pathToProjectOrTpzipFile);
Code snippet (Powershell):
$plc = $systemManager.LookupTreeItem("TIPC")
$newProject = $plc.CreateChild("NameOfProject", 1, "", pathToProjectOrTpzipFile)
TwinCAT PLC Projects consist of two different areas – the so-called Nested Project and the Project Instance. The Nested Project (tree item sub type 56) contains the source code of the PLC program whereas the Project Instance contains the declared input and output variables of the PLC program.
The following code snippet demonstrates a common way to generically access both tree items, if the full path name is not known.
Code Snippet (C#):
ITcSmTreeItem plc = sysManager.LookupTreeItem("TIPC");
foreach (ITcSmTreeItem plcProject in plc)
{ITcProjectRoot projectRoot = (ITcProjectRoot)plcProject;
ITcSmTreeItem nestedProject = projectRoot.NestedProject;
ITcSmTreeItem projectInstance = plcProject.get_Child(1);
}
Code snippet (Powershell):
$plc = $sysManager.LookupTreeItem("TIPC")
ForEach( $plcProject in $plc)
{
$nestedProject = $plcProject.NestedProject
$projectInstance = $plcProject.get_Child(1)
}
![]() | Please note A minimum of TwinCAT 3.1 Build 4018 is required to access the interface ITcProjectRoot. |
Saving the PLC project as a library
To save a PLC project as a PLC library, you need to make use of the ITcPlcIECProject::SaveAsLibrary() method.
Code snippet (C#):
iecProject.SaveAsLibrary(pathToLibraryFile, false);
Code snippet (Powershell):
$plcProject.SaveAsLibrary(pathToLibraryFile, $false)
The second parameter determines whether the library should be installed to the default repository after it has been saved as a file.
Handling online functionalities (Login, StartPlc, StopPlc, ResetCold, ResetOrigin)
Required version: TwinCAT 3.1 Build 4010 and above
The Automation Interface also provides you with PLC online features, for example to login to a PLC runtime and start/stop/reset the PLC program. These features can be accessed via the ITcSmTreeItem::ProduceXml() and ITcSmTreeItem::ConsumeXml() methods. These functions can be used on a ITcPlcIECProject node.
XML structure:
<TreeItem>
<IECProjectDef>
<OnlineSettings>
<Commands>
<LoginCmd>false</LoginCmd>
<LogoutCmd>false</LogoutCmd>
<StartCmd>false</StartCmd>
<StopCmd>false</StopCmd>
</Commands>
</OnlineSettings>
</IECProjectDef>
</TreeItem>
Code snippet (C#):
string xml = "<TreeItem><IECProjectDef><OnlineSettings><Commands><LoginCmd>true</LoginCmd></Commands></OnlineSettings></IECProjectDef></TreeItem>";
ITcSmTreeItem plcProject = systemManager.LookupTreeItem("TIPC^NameOfProject^NameOfProject Project");
plcProject.ConsumeXml(xml);
Code snippet (Powershell):
The following table describes every XML node in more detail:
XML |
Description |
---|---|
LoginCmd |
true = in SPS-Laufzeit einloggen |
LogoutCmd |
true = aus SPS-Laufzeit ausloggen |
StartCmd |
true = Starten des aktuell in die Laufzeit geladenen SPS-Programms |
StopCmd |
true = Stoppen des aktuell in die Laufzeit geladenen SPS-Programms |
Please note: In order to use commands like ResetOriginCmd, you must first execute a LoginCmd - similar to TwinCAT XAE.
Setting Boot project options
The following code snippet demonstrates how to use the ITcPlcProject interface to set Boot project options for a PLC project.
Code Snippet (C#):
ITcSmTreeItem plcProjectRoot = systemManager.LookupTreeItem(“TIPC^PlcGenerated”);
ITcPlcProject plcProjectRootIec = (ITcPlcProject) plcProjectRoot;
plcProjectRootIec.BootProjectAutostart = true;
plcProjectRootIec.GenerateBootProject(true);
Code snippet (Powershell):
$plcProject = $systemManager.LookupTreeItem(“TIPC^PlcGenerated“)
$plcProject.BootProjectAutostart = $true
$plcProject.GenerateBootProject($true)
Saving project and/or solution as archive
To save the whole TwinCAT solution in an ZIP compatible archive (*.tszip), the ITcSysManager9 interface may be used.
Code Snippet (C#):
ITcSysManager9 newSysMan = (ITcSysManager9)systemManager;
newSysMan.SaveAsArchive(@"C:\test.tszip");
Code snippet (Powershell):
$systemManager.SaveAsArchive("C:\test.tszip")
To reload a previously saved TSZIP file, the DTE method AddFromTemplate() may be used.
Code Snippet (C#):
dte.Solution.AddFromTemplate("C:\test.tszip",@"C:\tmp","CreatedFromTemplate");
Code snippet (Powershell):
$dte.Solution.AddFromTemplate("C:\test.tszip","C:\tmp","CreatedFromTemplate")
To save a specific PLC project in an ZIP compatible archive (*.tpzip), the method ITcSmTreeItem::ExportChild() may be used.
Code Snippet (C#):
ITcSmTreeItem plc= sysManager.LookupTreeItem("TIPC");
plc.ExportChild("PlcProject",@"C:\PlcTemplate.tpzip");
Code snippet (Powershell):
$plc = $systemManager.LookupTreeItem("TIPC")
$plc.ExportChild("PlcProject", "C:\PlcTemplate.tpzip")
To reload a previously saved TPZIP file, the ITcSmTreeItem::CreateChild() method may be used.
Code Snippet (C#):
plcConfig.CreateChild("PlcFromTemplate", 0, null, @"C:\PlcTemplate.tpzip");
Code snippet (Powershell):
$plc.CreateChild("plcFromTemplate", 0, $null, "C:\PlcTemplate.tpzip")
Calling CheckAllObjects()
To call the CheckAllObjects() method on the PLC Nested Project, you can use the corresponding method that is available in interface ITcPlcIECProject2.
Code snippet (C#):
ITcSmTreeItem plcProject = systemManager.LookupTreeItem("TIPC^NameOfProject^NameOfProject Project");
ITcPlcIECProject2 iecProject = (ITcPlcIECProject2) plcProject;
iecProject.CheckAllObjects();
Code snippet (Powershell):
$plcProject = $systemManager.LookupTreeItem("TIPC^NameOfProject^NameOfProject Project")
$plcProject.CheckAllObjects()