Job methods
The concept of Job methods has a fundamental difference compared to regular method calls: the OPC UA methods are no longer mapped 1:1 to a PLC method, but instead to a function block with a specific signature. This also allows method calls to be realized that take longer than one cycle from the perspective of a PLC application.
![]() | Requirements This functionality is only available for Data Access devices based on TwinCAT 3 and the import of TMC symbol files, as well as the online symbolism. |
The PLC-side structure of such a Job method is defined as follows: there is a function block which is defined as a Job method via a PLC attribute. The function block then contains various PLC methods that are accessed by the TwinCAT OPC UA Server in the form of a handshake mechanism in order to be able to provide them as OPC UA methods.
Method | Description |
---|---|
Start | Is called by the server as soon as an OPC UA client calls the OPC UA method. Contains the input parameters of the OPC UA method call as VAR_INPUT. The HRESULT return value of the method can be used to directly return an OPC UA Status Code in its decimal representation, e.g. “0” for the Status Code “Good”. The numerical value of a Status Code defined in the OPC UA specification is used as the value. A definition of all available Status Codes can be viewed here: http://www.opcfoundation.org/UA/schemas/StatusCode.csv Typically, this method returns the value “0” (Good). However, the PLC developer can also decide to validate the input parameters, for example. In the event of an error, the OPC UA method call could then fail, e.g. with the return value “2158690304” (BadInvalidArguments). |
CheckState | Is called cyclically by the server to check whether the job is still being processed or not. As long as the job is still being processed, this method returns the value “Busy”, otherwise “Done”. Output parameters for the OPC UA method are declared here as VAR_OUTPUT. The HRESULT return value of the method can be used to directly return an OPC UA Status Code in its decimal representation, e.g. “0” for the Status Code “Good”. The numerical value of a Status Code defined in the OPC UA specification is used as the value. A definition of all available Status Codes can be viewed here: http://www.opcfoundation.org/UA/schemas/StatusCode.csv If the job is processed successfully, this method typically returns the value “0” (Status Code “Good”). However, the PLC developer can also decide that the OPC UA method call should fail in the event of an error, e.g. with the return value “2151415808” (BadOutOfRange) or the more general “2147483648” (Bad). |
Abort | This method is called by the server if a job has to be aborted, for example if the server is shut down or restarted. The PLC developer then has the opportunity to clean up his PLC code accordingly. |
Workflow
The handshake mechanism between server and PLC can be represented in simplified form as follows:
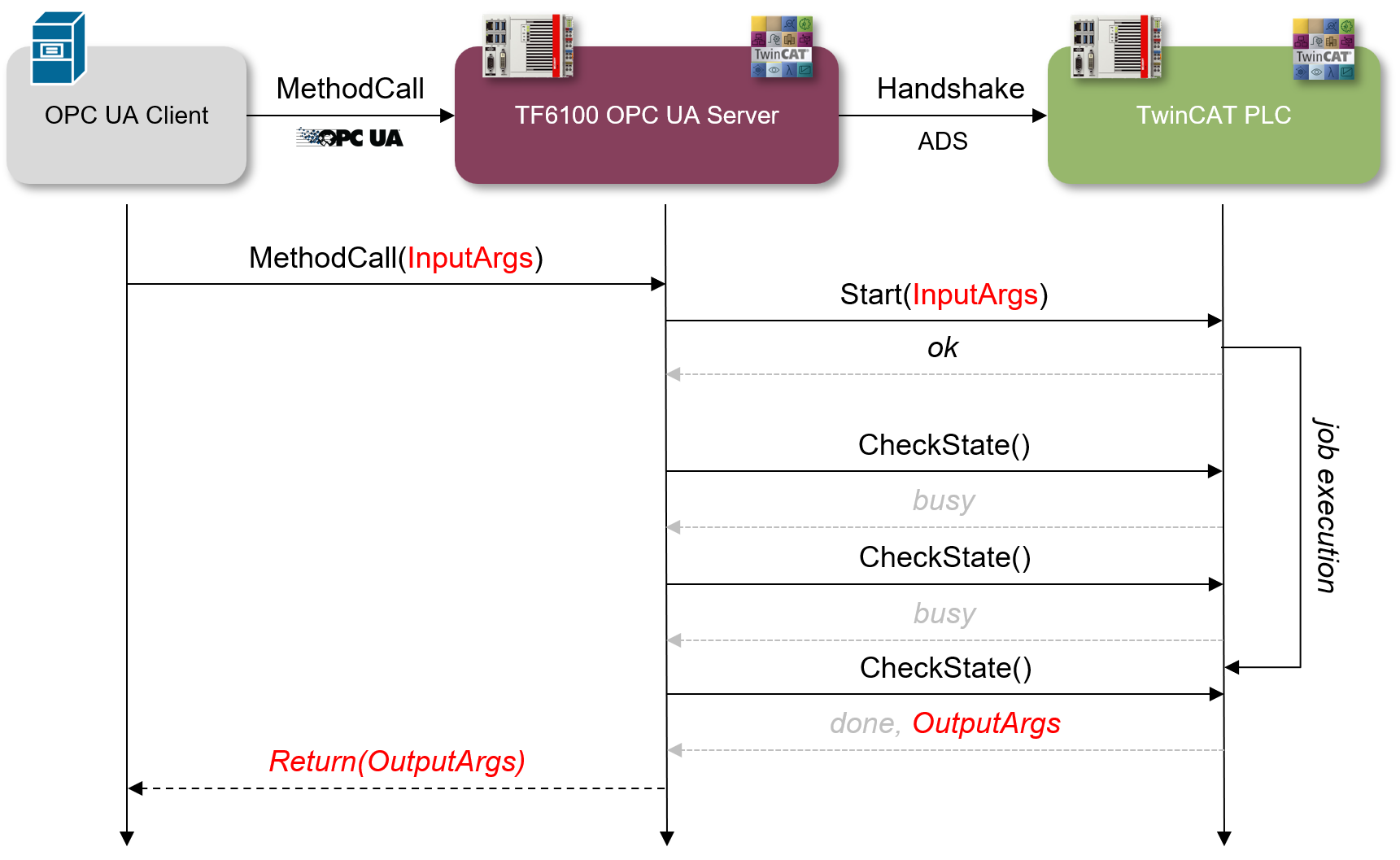
Example
The following example is also available in executable form at Examples. This part of the documentation is intended to explain the basic concepts of how the system works. The example “simulates” a Job method call that takes about four seconds to be processed in the PLC. This was realized with the help of a timer, which was declared and used in the function block part of the job. The State Machine of the function block delays the completion of the job (the CheckState handshake method returns “busy”) and the job is not completed until the timer has expired (the CheckState handshake method returns “done”).
In this example, a function block called FB_Job was created for the OPC UA method with the name “MyJob”, which has the required signature mentioned above.
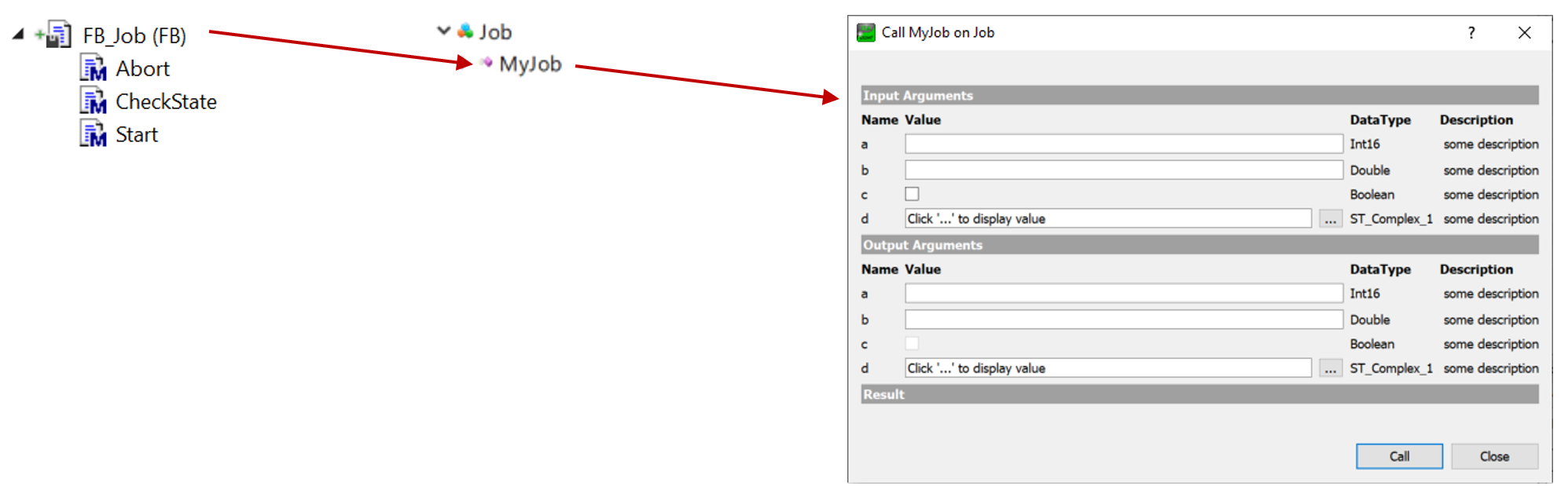
The function block declaration contains the attribute OPC.UA.DA.JobMethod and the name of the OPC UA method to be used as its value.
{attribute 'OPC.UA.DA.JobMethod' := 'MyJob'}
FUNCTION_BLOCK FB_Job
VAR
END_VAR
The three methods Abort, CheckState and Start contain the attribute TcRpcEnable in their declaration (so that the methods can also be called via ADS). Example:
{attribute 'TcRpcEnable' := '1'}
METHOD Start : HRESULT
{attribute 'TcRpcEnable' := '1'}
METHOD Abort : HRESULT
{attribute 'TcRpcEnable' := '1'}
METHOD CheckState : HRESULT
The input parameters of the OPC UA method are declared as VAR_INPUT in the PLC method Start(). Comments after the variables are used as a description of the respective OPC UA input parameter. Example:
{attribute 'TcRpcEnable' := '1'}
METHOD Start : HRESULT
VAR_INPUT
a : INT; // some description
b : LREAL; // some description
c : BOOL; // some description
d : ST_Complex_1; // some description
END_VAR
VAR_OUTPUT
hdl : UDINT; // handle, can be used for concurrent calls
END_VAR
The output parameters of the OPC UA method are declared as VAR_OUTPUT in the PLC method CheckState(). Comments after the variables are used as a description of the respective OPC UA output parameter. Example:
{attribute 'TcRpcEnable' := '1'}
METHOD CheckState : HRESULT
VAR_INPUT
hdl : UDINT; // handle, can be used for concurrent calls
END_VAR
VAR_OUTPUT
bBusy : BOOL; // required
a : INT; // some description
b : LREAL; // some description
c : BOOL; // some description
d : ST_Complex_1; // some description
END_VAR
(In this example, the values of the input parameters are applied 1:1 to the output parameters for illustrative purposes. The input and output parameters are therefore identical.)
Simultaneous method calls
Simultaneous method calls can be managed via the PLC application. For this purpose, a handle (output variable hdl) can be returned to the server via a return value of the Start() method, which is then used as an input variable for all further calls to the CheckState() method. This enables the PLC application to determine an execution context for the respective method call. In summary, the workflow is as follows:
- The Start() method determines the execution context and returns a handle to the server via the output variable “hdl”.
- The server now uses this handle for the cyclic CheckState() calls and lets the PLC application know which execution context is involved.