Copy image areas
In this sample, two images are copied to another image using different methods:
- Using the function F_VN_CopyImageRegionToRegion,
- Selecting a ROI and copying an image, and
- Selecting a ROI and adding two images using the function F_VN_AddImages.
Explanation
The methods shown below can be used to swap rectangular image areas between images. If required, this can also be done in a partially transparent manner with the aid of arithmetic image operations.
The function F_VN_CopyImageRegionToRegion takes two images and two image regions and copies the contents of one image region to the other. Both image regions have the same size and must be fully present in the image. The function is used in the sample to copy the red image to the mixed image. However, to copy the blue image, a programmed version with the same functionality is used. The advantage of this version is that instead of copying, it is also possible to mix the blue and the mixed image.
In the sample, the position of the two copied images can be changed using the variables aPositionRed
and aPositionBlue
. The parameter bCopyBlue
determines whether the blue image is copied or mixed.
Variables
hr : HRESULT;
ipImageMerge : ITcVnImage;
ipImageRed : ITcVnImage;
ipImageBlue : ITcVnImage;
ipImageMergeDisp : ITcVnDisplayableImage;
aBlack : TcVnVector4_LREAL := [0, 0, 0];
aRed : TcVnVector4_LREAL := [255, 0, 0];
aBlue : TcVnVector4_LREAL := [0, 0, 255];
aPositionRed : TcVnPoint := [20, 20];
aPositionBlue : TcVnPoint := [480, 480];
bCopyBlue : BOOL := FALSE;
Program
// create images & set colors
hr := F_VN_CreateImageAndSetPixels(ipImageMerge, 1000, 1000, TCVN_ET_USINT, 3, aBlack, hr);
hr := F_VN_CreateImageAndSetPixels(ipImageRed, 500, 500, TCVN_ET_USINT, 3, aRed, hr);
hr := F_VN_CreateImageAndSetPixels(ipImageBlue, 500, 500, TCVN_ET_USINT, 3, aBlue, hr);
// Copy red image to selected region in merge-image
hr := F_VN_CopyImageRegionToRegion(
ipSrcImage := ipImageRed,
nXSrc := 0, nYSrc := 0,
nWidth := 500, nHeight := 500,
ipDestImage := ipImageMerge,
nXDest := aPositionRed[0],
nYDest := aPositionRed[1],
hrPrev := hr
);
// Copy or mix blue image to selected region in merge-image
hr := F_VN_SetRoi(aPositionBlue[0], aPositionBlue[1], 500, 500, ipImageMerge, hr);
IF bCopyBlue THEN
hr := F_VN_CopyImage(ipImageBlue, ipImageMerge, hr);
ELSE
hr := F_VN_AddImages(ipImageBlue, ipImageMerge, ipImageMerge, hr);
END_IF
hr := F_VN_ResetRoi(ipImageMerge, hr);
hr := F_VN_TransformIntoDisplayableImage(ipImageMerge, ipImageMergeDisp, hr);
Result
The following are samples of the program results. In the images on the left and in the center, the blue square was added, in the image on the right it was copied. In addition, the target positions of the squares are different.
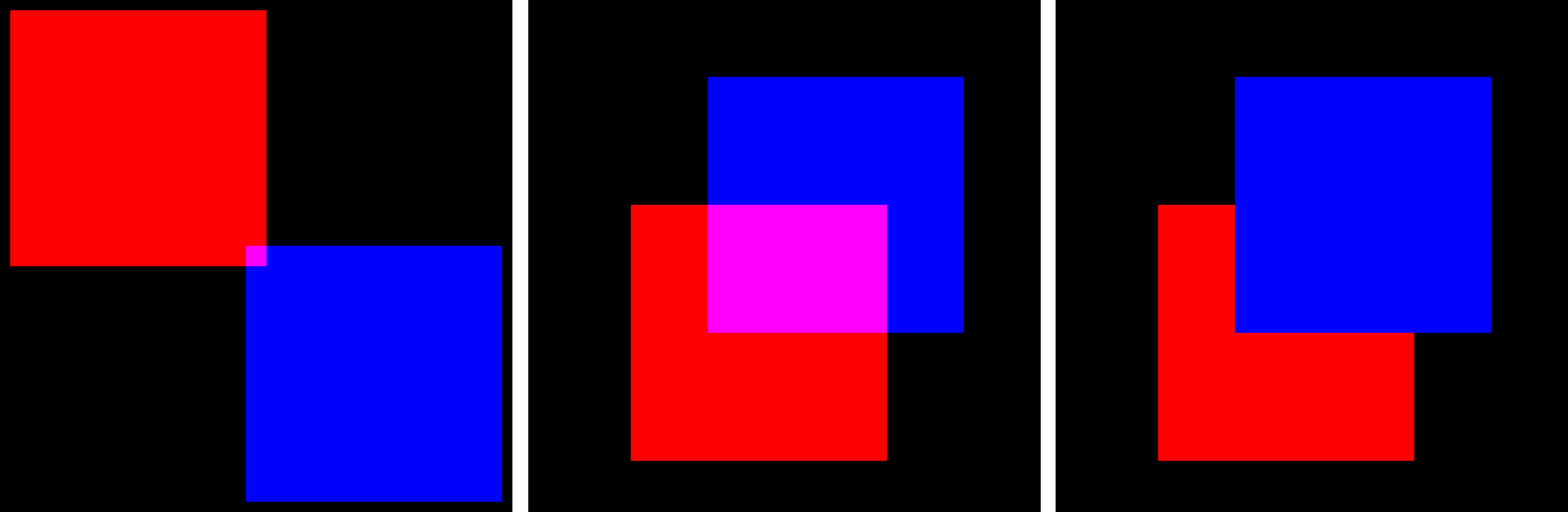