Reading/writing a register
All camera parameters that can be read or written via the Configuration Assistant in Config mode can also be read from the PLC via the function blocks FB_VN_ReadRegister_UDINT and FB_VN_ReadRegister_REAL or written via FB_VN_WriteRegister_UDINT and FB_VN_WriteRegister_REAL. The function block required depends on the data type of the parameter and can be read in the Property list on the Register Type. In this sample, the exposure time is written and read as an integer.
fbReadValue : FB_VN_ReadRegister_UDINT;
fbWriteValue : FB_VN_WriteRegister_UDINT;
Both function blocks require the address of the parameter and an indication of the byte sequence (endianness). The Write function block additionally requires the parameter value that is to be written to the camera. As the value displayed or entered under Value need not directly conform to how the camera processes it internally, e.g. in the case of enums or other byte sequences, this can be read under the Value register.
This information can be read from the Configuration Assistant:
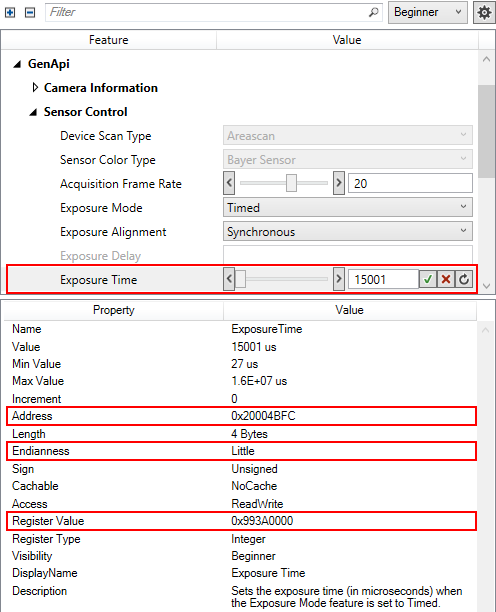
Notice | |
Camera-specific parameters The parameter names, properties and values shown here are vendor-specific or camera-specific and firmware-specific and may therefore differ. |
Variables
bTriggerReadValue : BOOL;
nReadValue : UDINT;
fbReadValue : FB_VN_ReadRegister_UDINT;
nReturnCodeRead : UDINT;
bTriggerWriteValue : BOOL;
nWriteValue : UDINT := 5000;
fbWriteValue : FB_VN_WriteRegister_UDINT;
nReturnCodeWrite : UDINT;
Code
fbReadValue(
nAddress := 16#20004BFC, // Check the Address of the Camera Parameter
nEndian := 1, // 0 = Big, 1 = Little
bRead := bTriggerReadValue,
nTimeout := T#5S,
nValue => nReadValue);
fbWriteValue(
nAddress := 16#20004BFC, // Check the Address of the Camera Parameter
nValue := nWriteValue, // Check the right Input Format
nEndian := 1, // 0 = Big, 1 = Little
bWrite := bTriggerWriteValue,
nTimeout := T#5S);
![]() | Conditions for the reading and writing of parameters Parameters can generally only be read or written with an opened camera connection ( |
Both function blocks must be initialized with the Image Provider of the camera:
