Check Color Range with RGB range
In this sample, the function F_VN_CheckColorRange searches for red, green and blue objects in the image and labels them according to their color. The function is applied to RGB images. It is also possible to apply the function to images in other color spaces such as HSV, Lab and BGR, in which case the parameterization must be adapted accordingly.
Variables
hr : HRESULT;
// Images
ipImageIn : ITcVnImage;
ipImageInDisp : ITcVnDisplayableImage;
ipImageWorkCol : ITcVnImage;
ipImageWorkColDisp : ARRAY [0..2] OF ITcVnDisplayableImage;
ipImageRes : ITcVnImage;
ipImageResDisp : ITcVnDisplayableImage;
// Colors
iColor : INT;
aColorTxt : ARRAY[0..2] OF STRING := [ 'RED', 'GREEN', 'BLUE' ];
aColor : ARRAY[0..2] OF TcVnVector4_LREAL := [ [150, 0, 0], [0, 255, 0], [0, 0, 255] ];
aColorRefLow : ARRAY[0..2] OF TcVnVector4_LREAL := [ [150, 50, 20], [35, 90, 60], [20, 40, 130] ];
aColorRefUp : ARRAY[0..2] OF TcVnVector4_LREAL := [ [255, 120, 100], [100, 200, 140], [60, 160, 255] ];
// Contours
ipContourList : ITcVnContainer;
ipIterator : ITcVnForwardIterator;
ipContour : ITcVnContainer;
fArea : LREAL;
aCenter : TcVnPoint2_LREAL;
Code
// Attention: With other images another color space transformation could be necessary
hr := F_VN_ConvertColorSpace(ipImageIn, ipImageRes, TCVN_CST_Bayer_RG_TO_RGB, hr);
FOR iColor := 0 TO 2 DO
// Apply a "Color-Threshold" on the image
hr := F_VN_CheckColorRange( ipSrcImage := ipImageRes,
ipDestImage := ipImageWorkCol,
aLowerBounds := aColorRefLow[iColor],
aUpperBounds := aColorRefUp[iColor],
hrPrev := hr );
// Find all objects / contours in the black and white image
hr := F_VN_FindContours(ipImageWorkCol, ipContourList, hr);
hr := F_VN_GetForwardIterator(ipContourList, ipIterator, hr);
// Filter the objects by size and draw the contours
WHILE SUCCEEDED(hr) AND_THEN ipIterator.CheckIfEnd() <> S_OK DO
hr := F_VN_GetContainer(ipIterator, ipContour, hr);
hr := F_VN_IncrementIterator(ipIterator, hr);
// Filter contours by size
hr := F_VN_ContourArea(ipContour, fArea, hr);
IF fArea > 5000 THEN
// Draw Results into an Image
hr := F_VN_DrawContours(ipContour, -1, ipImageRes, aColor[iColor], 3, hr);
hr := F_VN_ContourCenterOfMass(ipContour, aCenter, hr);
hr := F_VN_PutText(aColorTxt[iColor], ipImageRes, LREAL_TO_UDINT(aCenter[0])-30, LREAL_TO_UDINT(aCenter[1])+10, TCVN_FT_HERSHEY_PLAIN, 2, aColor[iColor], hr);
END_IF
END_WHILE
END_FOR
Result
The input image in Bayer RG format.
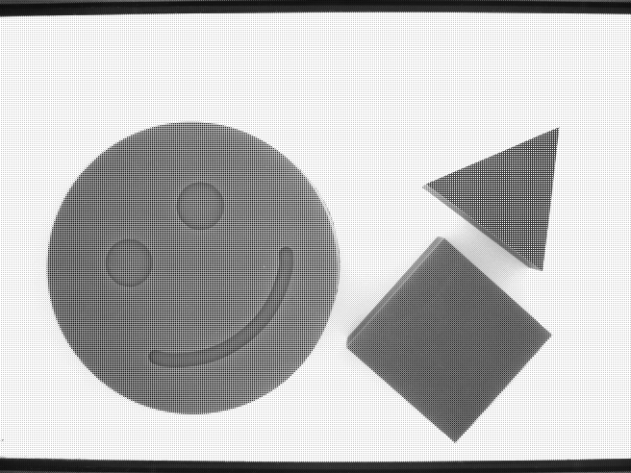
The input image is converted into an RGB image with the function F_VN_ConvertColorSpace.
hr := F_VN_ConvertColorSpace(ipImageIn, ipImageRes, TCVN_CST_Bayer_RG_TO_RGB, hr);
The function F_VN_CheckColorRange can then be used with the respective upper and lower threshold values.
hr := F_VN_CheckColorRange(ipImageRes, ipImageWorkCol, aColorRefLow[iColor], aColorRefUp[iColor], hr);
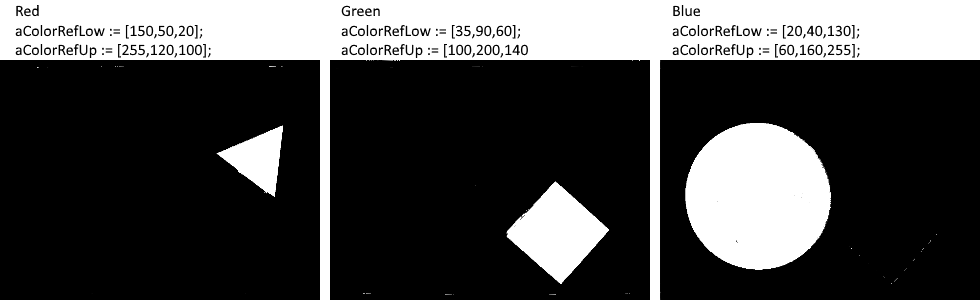
The result image, after subsequent detection
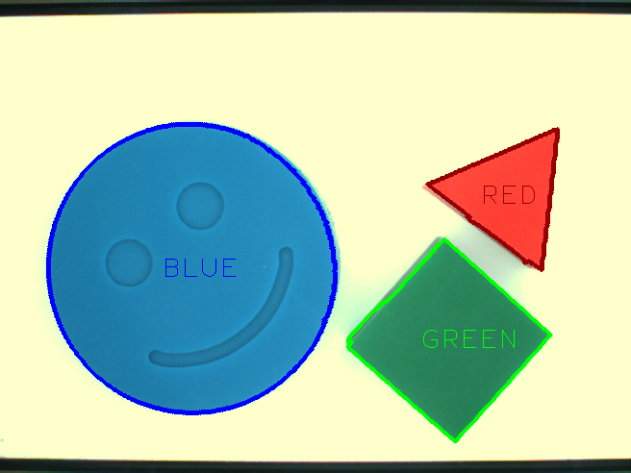