User-defined color format
As another example of the Color Control configuration, an RGB color format with a value range between 0 and 1 is considered. To display this in the Color Control, the channels must be set in the ColorChannel
attribute as float
and with a maxValue
of 1
.
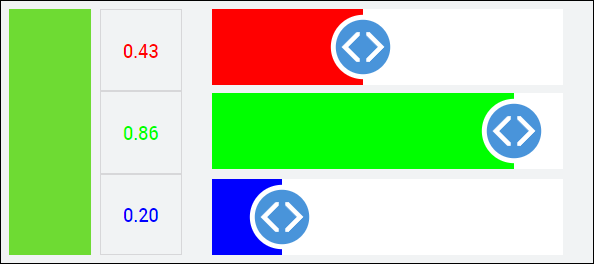
The values of the ColorChannels
attribute can be written by script as follows:
[
{
"color": {
"color": "rgba(255, 0, 0, 1)"
},
"float": true,
"maxValue": 1.0
},
{
"color": {
"color": "rgba(0, 255, 0, 1)"
},
"float": true,
"maxValue": 1.0
},
{
"color": {
"color": "rgba(0, 0, 255, 1)"
},
"float": true,
"maxValue": 1.0
}
]
ColorBoxFormatting function
In order for the color box to display the correct color despite the changed value range, the BoxColorConversion
attribute must be adjusted. Unlike the standard conversions, there is no ready-made function for this. Instead, the creation of a separate function is necessary. The following sample shows how such a function is created for the use case mentioned above and which interfaces it must have.
![]() | The general use of functions is explained in the HMI documentation. |
After creating the TypeScript function, the WaitMode must be changed to Asynchronous. As a result, the parameter "ctx" is created, which is required for the return. Two parameters must then be created. The first one gets the color value to be converted and therefore must be created of type TcHmi.Controls.Beckhoff.Vision.Vector4
. The second parameter specifies the conversion direction and must be created as Boolean
. With True
the values are converted to RGB and with False
from RGB back to the user-defined color format. It should be noted that these passed parameters may differ from the values of the corresponding control properties at the time of function execution for timing reasons.
The function must return a value of type TcHmi.Controls.Beckhoff.Vision.TcHmiVnColor.ColorValue
, which is then displayed in the color box.
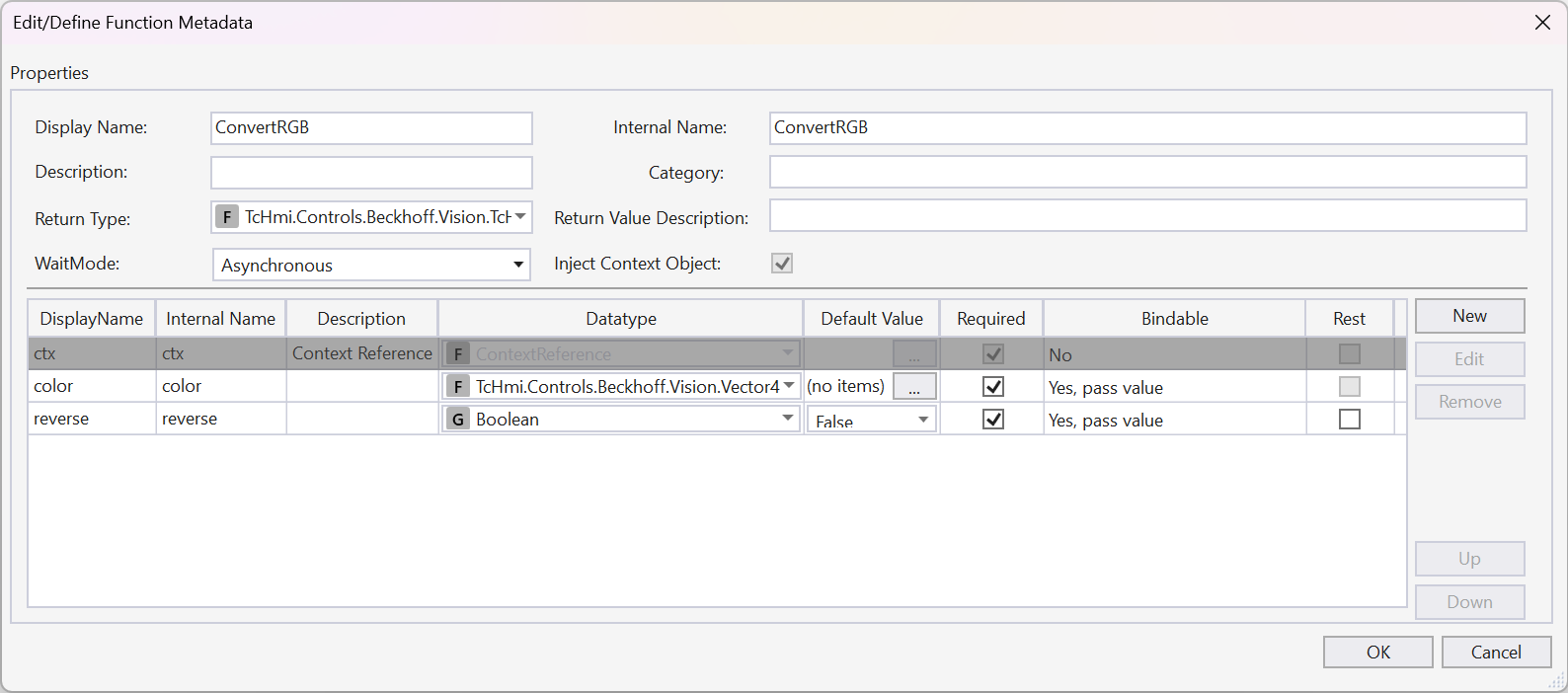
In the following code sample, the scaling of the color values is adjusted. If the parameter is reverse = false
, the color values are transferred from the control to the function in the user-defined format. The color format must then be converted to RGB(a) in order to be displayed in the ColorBox. The color values transferred in the "color" parameter are always 4-channel. If no transparent color is to be displayed in the ColorBox, the returned color values must be 3-channel. Alternatively, the fourth channel can also be set to 255.
If the parameter is reverse = true
, color values from the color selection dialog are transferred to the function in RGBa format. The color format must then be converted to the user-defined color format. The fourth channel is taken from the color values of the control because the color selection dialog does not support transparency.
namespace TcHmi {
export namespace Functions {
export namespace TcHmiProject {
export function ConvertRGB(ctx: any, color: any, reverse: any) {
// Important: color has 4 channels. If the ColorBox should be RGB, return a 3 channel color or set the fourth channel (a) to 255
if (!reverse) {
ctx.success(color.slice(0, 3).map((value: number) => value * 255));
}
else {
ctx.success(color.slice(0, 3).map((value: number) => value / 255));
}
}
}
}
}
TcHmi.Functions.registerFunctionEx('ConvertRGB', 'TcHmi.Functions.TcHmiProject', TcHmi.Functions.TcHmiProject.ConvertRGB);
This ensures that the color box displays the correct color and that manually changing the color in the color box results in correct color values.