FB_IotCommunicator
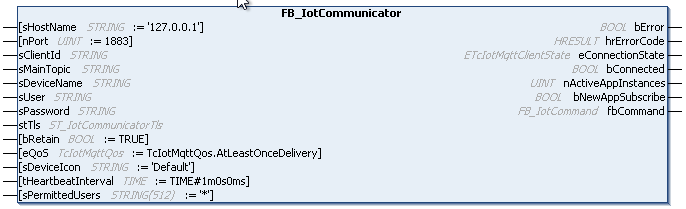
The function block enables communication with an MQTT broker.
An FB_IotCommunicator function block deals with the connection to precisely one broker and with sending and receiving of data for precisely one device. To ensure the background communication to this broker and thus enable sending and receiving of data and messages, the Execute method of the function block must be called cyclically.
All connection parameters exist as input parameters and are evaluated when a connection is established.
Syntax
Definition:
FUNCTION_BLOCK FB_IotCommunicator
VAR_INPUT
sHostName : STRING := '127.0.0.1';
nPort : UINT := 1883;
sClientId : STRING;
sMainTopic : STRING;
sDeviceName : STRING;
sUser : STRING;
sPassword : STRING;
stTls : ST_IotCommunicatorTls;
bRetain : BOOL := TRUE;
eQoS : TcIotMqttQos := TcIotMqttQos.AtLeastOnceDelivery;
sDeviceIcon : STRING;
tHeartbeatInterval : TIME := TIME#1m0s0ms;
sPermittedUsers : STRING(512) := '*';
END_VAR
VAR_OUTPUT
bError : BOOL;
hrErrorCode : HRESULT;
eConnectionState : ETcIotMqttClientState;
bConnected : BOOL;
nActiveAppInstances : UINT;
bNewAppSubscribe : BOOL;
fbCommand : FB_IoTCommand;
END_VAR
Inputs
Name | Type | Description |
---|---|---|
sHostName | STRING | sHostName can be specified as the host name or as the IP address. If no information is provided, the local host is used. |
nPort | UINT | The host port is specified here. (Default: 1883) |
sClientId | STRING | The client ID can be specified individually. If no ID is specified, it is generated. |
sMainTopic | STRING | Here you specify the main topic in which the data and messages are sent. |
sDeviceName | STRING | Here you can enter the name of the device to which the data and messages belong. |
sUser | STRING | Optionally, a user name can be specified. |
sPassword | STRING | A password for the user name can be entered here. |
stTLS | Parameter structure | |
bRetain | BOOL | By default, the broker stores the current data and the last 255 messages, together with the current device status. If this is not desirable, bRetain can be set to FALSE. |
eQoS | TcIotMqttQos | The Quality of Service (QoS for short) can be set with this setting. |
sDeviceIcon | STRING | This setting can be used to change the icon of the Communicator function block. If the setting is not set, the TwinCAT CD is used as icon by default. The list of available icons can be found at List of available icons. |
tHeartbeatInterval | TIME | The timespan after which the online information of this function block is updated. The online information is visible at the top level in the app, where you can see the overview of the individual function blocks. The default value is 60 seconds. |
sPermittedUsers | STRING(512) | This variable can be used to specify the users who are authorized to see a device. The users are separated by a comma. With the default value '*', all users are authorized to see the device. |
Outputs
Name | Type | Description |
---|---|---|
bError | BOOL | TRUE if an error situation occurs. |
hrErrorCode | HRESULT | Returns an error code if the bError output is set. |
eConnectionState | ETcIotMqttClientState | Indicates the state of the connection between client and broker as enumeration ETcIotMqttClientState. |
bConnected | BOOL | TRUE if there is a connection between the client and the broker. |
nActiveAppInstances | UINT | Shows the number of currently connected app instances. |
bNewAppSubscribe | BOOL | Goes TRUE for one cycle when a new app instance has connected. Can be used when using OnChange functionalities to publish the current status of the data again as a retain message during the connection. |
fbCommand | Provides all the necessary functionality to evaluate received data ("Commands"). |
Methods
Name | Description |
---|---|
Method for background communication with the TwinCAT driver. This method must be called cyclically. | |
Method for sending data to the specified MQTT message broker as a retain message. | |
Method for sending a (push) message to the specified MQTT message broker. | |
Method for sending data to the specified MQTT message broker where the JSON document is transferred directly. | |
Method for sending OnChange data to the specified MQTT message broker. | |
Method for sending OnChange data to the specified MQTT message broker where the JSON document is transferred directly. |
![]() | Strings in UTF-8 format The variables of type STRING used here are based on the UTF-8 format. This STRING formatting is common for MQTT communication. In order to be able to receive special characters and texts from a wide range of languages, the character set in the Tc3_IotCommunicator library is not limited to the typical character set of the data type STRING. Instead, the Unicode character set in UTF-8 format is used in conjunction with the data type STRING. If the ASCII character set is used, there is no difference between the typical formatting of a STRING and the UTF-8 formatting of a STRING. |
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.4022.0 | IPC or CX (x86, x64, ARM) | Tc3_IotCommunicator |