FB_IotMqttClient
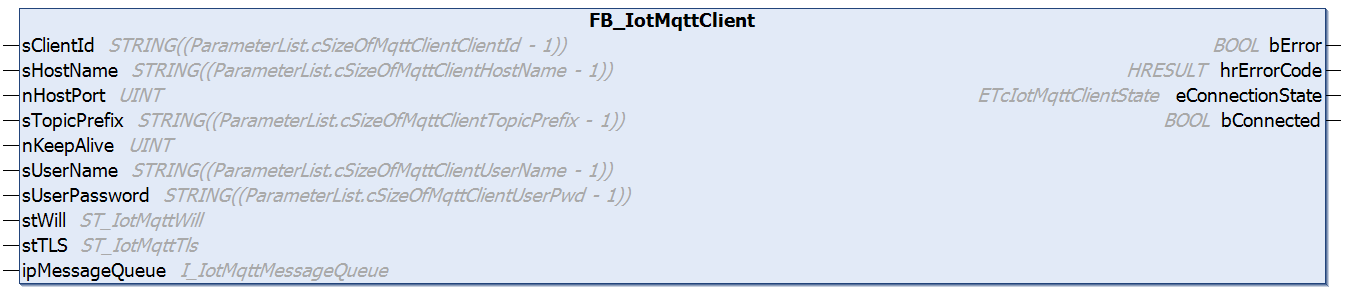
The function block enables communication to a message broker based on MQTT version 3.1.1.
A client function block is responsible for the connection to precisely one broker. The Execute() method of the function block must be called cyclically in order to ensure the background communication with this broker and facilitate receiving of messages.
All connection parameters exist as input parameters and are evaluated when a connection is established.
Syntax
Definition:
FUNCTION_BLOCK FB_IotMqttClient
VAR_INPUT
sClientId : STRING(255); // default is generated during initialization
sHostName : STRING(255) := '127.0.0.1'; // default is local host
nHostPort : UINT := 1883; // default is 1883
sTopicPrefix : STRING(255); // topic prefix for pub and sub of this client (handled internally)
nKeepAlive : UINT := 60; // in seconds
sUserName : STRING(255); // optional parameter
sUserPassword : STRING(255); // optional parameter
stWill : ST_IotMqttWill; // optional parameter
stTLS : ST_IotMqttTls; // optional parameter
ipMessageQueue : I_IotMqttMessageQueue; // if received messages should be queued during call of Execute()
END_VAR
VAR_OUTPUT
bError : BOOL;
hrErrorCode : HRESULT;
eConnectionState : ETcIotMqttClientState;
bConnected : BOOL; // TRUE if connection to host is established
END_VAR
Inputs
Name | Type | Description |
---|---|---|
sClientId | STRING(255) | The client ID can be specified individually and must be unique for most message brokers. If not specified, an ID based on the PLC project name is automatically generated by the TwinCAT driver. |
sHostName | STRING(255) | sHostName can be specified as name or as IP address. If no information is provided, the local host is used. |
nHostPort | UINT | The host port is specified here. The default is 1883. |
sTopicPrefix | STRING(255) | Here you can specify a topic prefix that will be added automatically for all publish and subscribe commands. |
nKeepAlive | UINT | Here you can specify the watchdog time (in seconds), with which the connection between client and broker is monitored. |
sUserName | STRING(255) | Optionally, a user name can be specified. |
sUserPassword | STRING(255) | A password for the user name can be entered here. |
stWill | If the client is disconnected from the broker irregularly, a last preconfigured message can optionally be sent from the broker to the so-called “will topic”. This message is specified here. | |
stTLS | If the broker offers a TLS-secured connection, the required configuration can be implemented here. | |
ipMessageQueue | I_IotMqttMessageQueue | An instance of FB_IotMqttMessageQueue can optionally be assigned here. This input parameter can be used for storing incoming new messages in the message queue without implementing the callback method. (See also IotMqttSampleUsingQueue) If you want to evaluate incoming new messages without a message queue by implementing the callback method yourself, this input parameter is not needed. (See also IotMqttSampleUsingCallback) No message queue is used if the callback method is used or overwritten, irrespective of whether ipMessageQueue was set. |
Outputs
Name | Type | Description |
---|---|---|
bError | BOOL | Becomes TRUE as soon as an error situation occurs. |
hrErrorCode | HRESULT | Returns an error code if the bError output is set. An explanation of the possible error codes can be found in the Appendix. |
eConnectionState | Indicates the state of the connection between client and broker as enumeration ETcIotMqttClientState. | |
bConnected | BOOL | This output is TRUE if a connection exists between client and broker. |
Methods
Name | Description |
---|---|
Method for background communication with the TwinCAT driver. The method must be called cyclically. | |
Method for executing a publish operation to a MQTT message broker. | |
Method for establishing a subscription. | |
Method for removing a subscription. | |
Activates the exponential backoff function | |
Deactivates the exponential backoff function | |
Method for querying the time (in ms) since the last message from the message broker. |
Event-driven methods (callback methods)
Name | Description |
---|---|
Callback method used by the TwinCAT driver when a subscription to a topic was established and incoming messages are received. |
![]() | Message payload formatting Note that the data type and the formatting of the content must be known to the sender and receiver side, particularly when binary information (alignment) or strings (with or without zero termination) are sent. |
![]() | Strings in UTF-8 format The variables of type STRING used here are based on the UTF-8 format. This STRING formatting is common for MQTT communication as well as for JSON documents. In order to be able to receive special characters and texts from a wide range of languages, the character set in the Tc3_IotBase and Tc3_JsonXml libraries is not limited to the typical character set of the data type STRING. Instead, the Unicode character set in UTF-8 format is used in conjunction with the data type STRING. If the ASCII character set is used, there is no difference between the typical formatting of a STRING and the UTF-8 formatting of a STRING. Further information on the UTF-8 STRING format and available display and conversion options can be found in the documentation for the Tc2_Utilities PLC library. |
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.4022.0 | IPC or CX (x86, x64, ARM) | Tc3_IotBase |