Overview
The following example shows an implementation of an "echo" client/server. The client sends a test string to the server at certain intervals (e.g. every second). The remote server then immediately resends the same string to the client.
In this sample, the client is implemented in the PLC and as a .NET application written in C#. The PLC client can create several instances of the communication, simulating several TCP connections at once. The .NET sample client only establishes one concurrent connection. The server is able to communicate with several clients.
In addition, several instances of the server may be created. Each server instance is then addressed via a different port number which can be used by the client to connect to a specific server instance. The server implementation is more difficult if the server has to communicate with more than one client.
Feel free to use and customize this sample to your needs.
System requirements
- TwinCAT 3 Build 3093 or higher
- TwinCAT 3 Function TF6310 TCP/IP
- If two computers are used to execute the sample (one client and one server), the Function TF6310 needs to be installed on both computers
- If one computer is used to execute the sample, e.g. client and server running in two separate PLC runtimes, both PLC runtimes need to run in separate tasks
- To run the .NET sample client, only .NET Framework 4.0 is needed
Project downloads
https://github.com/Beckhoff/TF6310_Samples/tree/master/PLC/TCP/Sample01
https://github.com/Beckhoff/TF6310_Samples/tree/master/C%23/SampleClient
Project description
The following links provide documentation for the three components. Additionally, an own article explains how to start the PLC samples with step-by-step instructions.
- Integration in TwinCAT and Test (Starting the PLC samples)
- PLC Client (PLC client documentation: FB_LocalClient function block)
- PLC Server (PLC serve documentation: FB_LocalServer function block)
- .NET client (.NET client documentation: .NET sample client)
Additional functions of the PLC sample projects
Some functions, constants and function blocks are used in the sample projects, which are briefly described below:
LogError function
FUNCTION LogError : DINT
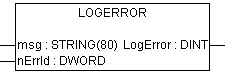
The function writes a message with the error code into the logbook of the operating system (Event Viewer). The global variable bLogDebugMessages must first be set to TRUE.
LogMessage function
FUNCTION LogMessage : DINT
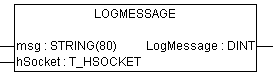
The function writes a message into the logbook of the operating system (Event Viewer) if a new socket was opened or closed. The global variable bLogDebugMessages must first be set to TRUE.
SCODE_CODE function
FUNCTION SCODE_CODE : DWORD
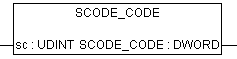
The function masks the least significant 16 bits of a Win32 error code returns them.
Global variables
Name | Default value | Description |
---|---|---|
bLogDebugMessages | TRUE | Activates/deactivates writing of messages into the log book of the operating system |
MAX_CLIENT_CONNECTIONS | 5 | Max. number of remote clients, that can connect to the server at the same time. |
MAX_PLCPRJ_RXBUFFER_SIZE | 1000 | Max. length of the internal receive buffer |
PLCPRJ_RECONNECT_TIME | T#3s | Once this time has elapsed, the local client will attempt to re-establish the connection with the remote server |
PLCPRJ_SEND_CYCLE_TIME | T#1s | The test string is sent cyclically at these intervals from the local client to the remote server |
PLCPRJ_RECEIVE_POLLING_TIME | T#1s | The client reads (polls) data from the server using this cycle |
PLCPRJ_RECEIVE_TIMEOUT | T#10s | After this time has elapsed, the local client aborts the reception if no data bytes could be received during this time |
PLCPRJ_ERROR_RECEIVE_BUFFER_OVERFLOW | 16#8101 | Sample project error code: Too many characters without zero termination were received |
PLCPRJ_ERROR_RECEIVE_TIMEOUT | 16#8102 | Sample project error code: No new data could be received within the timeout time (PLCPRJ_RECEIVE_TIMEOUT) |