Structures
![]() | Requirements This functionality is only available for Data Access devices based on TwinCAT 3 and the import of TMC symbol files. |
The TwinCAT OPC UA Server enables the use of so-called StructuredTypes for structures from the TwinCAT 3 PLC. StructuredTypes allow structures to be read or written in a data-consistent manner and the type description of the structure to be made available to the client.
You can use a special pragma to define a structure as a StructuredType and make it available. If this pragma is not set, the structure is not loaded into the server's address space as a StructuredType, but is displayed as a FolderType. The member variables of the structure are displayed as separate nodes that can be accessed.
The following sample of a structure in the TwinCAT 3 PLC is given:
TYPE ST_Communication :
STRUCT
a : INT;
b : INT;
c : INT;
END_STRUCT
END_TYPE
This structure is now instantiated in the MAIN program and enabled for OPC UA via a pragma, as described in the chapter Enabling (PLC) symbols.
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA' := '1'}
stCommunication : ST_Communication;
END_VAR
The structure instance is now provided in the server as follows by default:
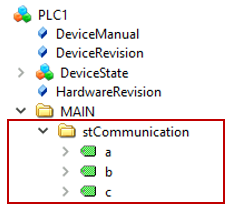
The individual member variables of the structure can now be accessed, but not the root element of the structure. As a result, data-consistent access to the entire structure may not be possible.
Using another pragma, this structure instance is now defined as a StructuredType.
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA' := '1'}
{attribute 'OPC.UA.DA.StructuredType' := '1'}
stCommunication : ST_Communication;
END_VAR
The instance is then provided in the TwinCAT OPC UA Server as follows:
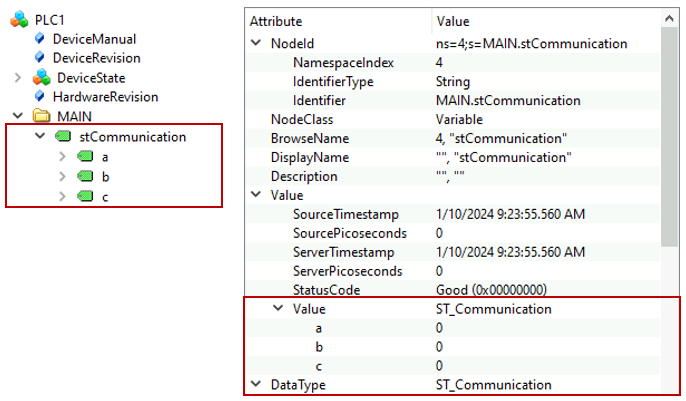
When the root element is read, the structure information is provided in a data-consistent manner via OPC UA and can be processed by a client. In addition, the member variables are also displayed as separate nodes and can be accessed. You can deactivate this via a special attribute. The member variables can then only be accessed via the StructuredType, as shown in the following sample:
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA' := '2'}
{attribute 'OPC.UA.DA.StructuredType' := '1'}
stCommunication : ST_Communication;
END_VAR
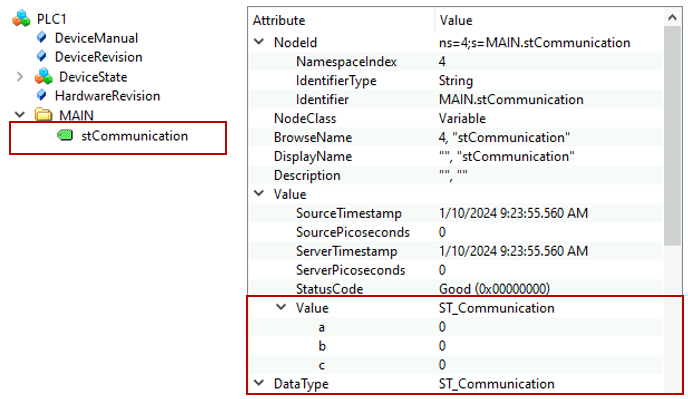
This is primarily a memory optimization of the server, as also described in the chapter Optimizations, because main memory must be allocated for each node in the address space.
You do not necessarily have to place the pragmas at every instance of a structure, but can also do this once at the structure definition. In this case, all instances of this structure are automatically enabled for OPC UA as StructuredType.
{attribute 'OPC.UA.DA' := '1'}
{attribute 'OPC.UA.DA.StructuredType' := '1'}
TYPE ST_Communication :
STRUCT
a : INT;
b : INT;
c : INT;
END_STRUCT
END_TYPE
In this case, you can also explicitly deactivate the StructuredType definition for certain instances by using the following attribute:
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA.StructuredType' := '0'}
stCommunication : ST_Communication;
END_VAR
StructuredType for function blocks
You can also use StructuredTypes with PLC function blocks. In this case, a function block instance receives a child node called "FunctionBlock", which represents the entire function block as a StructuredType.
Consider the following sample:
FUNCTION_BLOCK FB_FunctionBlock
VAR_INPUT
Input1 : INT;
Input2 : LREAL;
END_VAR
VAR_OUTPUT
Output1 : LREAL;
END_VAR
An instance of the function block is created in the MAIN program and both enabled for OPC UA and defined as a StructuredType via pragmas.
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA' := '1'}
{attribute 'OPC.UA.DA.StructuredType' := '1'}
fbFunctionBlock : FB_FunctionBlock;
END_VAR
The TwinCAT OPC UA then provides the function block instance as follows:
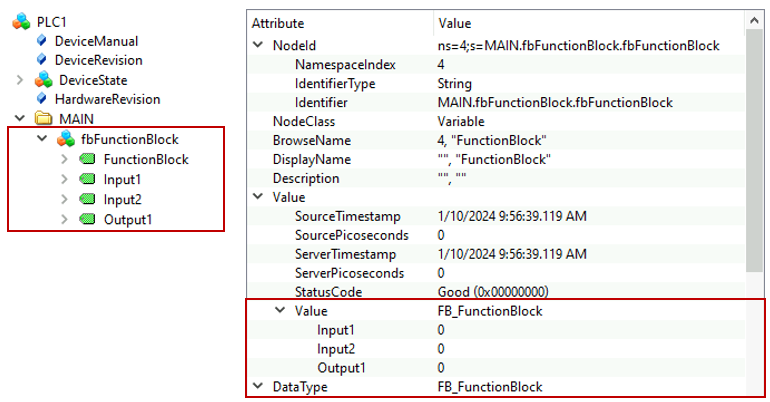
The definition locations of pragmas mentioned above for structures also apply to function blocks. You can also explicitly hide the member variables of a function block here.
Restrictions
The following restrictions apply when using StructuredTypes.
![]() | Pointers and references If pointer and reference types are used in the structure, they cannot be converted into a StructuredType. The OPC UA Server then illustrates these structures as regular FolderTypes with the corresponding member variables. |
![]() | Maximum size of the structure The maximum size of a structure is limited to 16 kB by default. If the size of the structure exceeds this limit, structure instances are displayed as FolderType. You can increase this limit if necessary. The background is described in more detail in the chapter Optimizations. Each STRUCT constantly exchanges data with the basic ADS device, i.e. a large ADS message is sent with each read/write command of a StructuredType. To prevent the ADS router from being flooded with large messages, the maximum size is limited. |