FB_CTRL_TRANSFERFUNCTION_2
This function block calculates a discrete transfer function with the second standard form shown below. The transfer function here can be of any order, n.
The coefficients for the following transfer functions are stored in the parameter arrays:
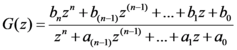
Description of the transfer behavior
The internal calculation is performed in each sampling step according to the second standard form, for which the following block diagram applies:
The transfer function can be brought into the form shown in the block diagram by means of some transformations:
The coefficients of the counter polynomial are calculated according to the following rule:
The programmer must create the following arrays in the PLC if this function block is to be used:
aNumArray : ARRAY[0..nTfOrder] OF FLOAT;
aDenArray : ARRAY[0..nTfOrder] OF FLOAT;
aStTfData : ARRAY[0..nTfOrder] OF ST_CTRL_TRANSFERFUNCTION_2_DATA;
The coefficients "b0" to "bn" are stored in the array aNumArray
. This must be organized as follows:
aNumArray[0]:= b0;
aNumArray[1]:= b1;
...
aNumArray[n-1] := bn-1;
aNumArray[n] := bn;
The coefficients "a0" to "an" are stored in the array aDenArray
. This must be organized as follows:
aDenArray[0]:= a0;
aDenArray[1]:= a1;
...
aDenArray[n-1] := an-1;
aDenArray[n] := an;
The internal data required by the function block is stored in the array aStTfData
. This data must never be modified from within the PLC program. This procedure is also illustrated in the sample program listed below.
Inputs
VAR_INPUT
fIn : FLOAT;
fManValue : FLOAT;
eMode : E_CTRL_MODE;
END_VAR
Name | Type | Description |
---|---|---|
fIn | FLOAT | Input to the transfer function |
fManValue | FLOAT | Input whose value is present at the output in manual mode. |
eMode | E_CTRL_MODE | Input that specifies the operation mode of the function block. |
Outputs
VAR_OUTPUT
fOut : FLOAT;
eState : E_CTRL_STATE;
bError : BOOL;
eErrorId : E_CTRL_ERRORCODES;
END_VAR
Name | Type | Description |
---|---|---|
fOut | FLOAT | Output from the transfer function |
eState | E_CTRL_STATE | State of the function block |
bError | BOOL | Supplies the error number when the output |
eErrorId | E_CTRL_ERRORCODES | Becomes TRUE, as soon as an error occurs. |
Inputs/ outputs
VAR_IN_OUT
stParams : ST_CTRL_TRANSFERFUNCTION_2_PARAMS;
END_VAR
Name | Type | Description |
---|---|---|
stParams | ST_CTRL_TRANSFERFUNCTION_2_PARAMS | Parameter structure of the function block |
stParams
consists of the following elements:
TYPE
ST_CTRL_TRANSFERFUNCTION_2_PARAMS:
STRUCT
tTaskCycleTime : TIME;
tCtrlCycleTime : TIME := T#0ms;
nOrderOfTheTransferfunction : USINT;
pNumeratorArray_ADR : POINTER TO FLOAT := 0;
nNumeratorArray_SIZEOF : UINT;
pDenominatorArray_ADR : POINTER TO FLOAT := 0;
nDenomiantorArray_SIZEOF : UINT;
pTransferfunction2Data_ADR : POINTER TO
ST_CTRL_TRANSFERFUNCTION_2_DATA;
nTransferfunction2Data_SIZEOF : UINT;
END_STRUCT
END_TYPE
Name | Type | Description |
---|---|---|
tTaskCycleTime | TIME | Cycle time with which the function block is called. If the function block is called in every cycle this corresponds to the task cycle time of the calling task. |
tCtrlCycleTime | TIME | Cycle time with which the control loop is processed. This must be greater than or equal to the TaskCycleTime. The function block uses this input value to calculate internally whether the state and the output values have to be updated in the current cycle. |
nOrderOfTheTransferfunction | USINT | Order of the transfer function [0...] |
pNumeratorArray_ADR | POINTER TO FLOAT | Address of the array containing the numerator coefficients |
nNumeratorArray_SIZEOF | UINT | Size of the array containing the numerator coefficients, in bytes |
pDenominatorArray_ADR | POINTER TO FLOAT | Address of the array containing the denominator coefficients |
nDenomiantorArray_SIZEOF | UINT | Size of the array containing the denominator coefficients, in bytes |
pTransferfunction2Data_ADR | POINTER TO | Address of the data array |
nTransferfunction2Data_SIZEOF | UINT | Size of the data array in bytes |
Sample:
PROGRAM PRG_TRANSFERFUNCTION_2_TEST
VAR CONSTANT
nTfOrder : USINT := 2;
END_VAR
VAR
aNumArray : ARRAY[0..nTfOrder] OF FLOAT;
aDenArray : ARRAY[0..nTfOrder] OF FLOAT;
aStTfData : ARRAY[0..nTfOrder] OF ST_CTRL_TRANSFERFUNCTION_2_DATA;
eMode : E_CTRL_MODE;
stParams : ST_CTRL_TRANSFERFUNCTION_2_PARAMS;
eErrorId : E_CTRL_ERRORCODES;
bError : BOOL;
fbTansferfunction : FB_CTRL_TRANSFERFUNCTION_2;
bInit : BOOL := TRUE;
fIn : FLOAT := 0;
fOut : FLOAT;
b_0, b_1, b_2 : FLOAT;
a_0,a_1,a_2 : FLOAT;
END_VAR
IF bInit THEN
aNumArray[0] := 1.24906304658218E-007;
aNumArray[1] := 2.49812609316437E-007;
aNumArray[2] := 1.24906304658218E-007;
aDenArray[0] := 0.998501124344101;
aDenArray[1] := -1.99850062471888;
aDenArray[2] := 1.0;
stParams.tTaskCycleTime := T#2ms;
stParams.tCtrlCycleTime := T#2ms;
stParams.nOrderOfTheTransferfunction := nTfOrder;
eMode := eCTRL_MODE_ACTIVE;
bInit := FALSE;
END_IF
stParams.pNumeratorArray_ADR := ADR(aNumArray);
stParams.nNumeratorArray_SIZEOF := SIZEOF(aNumArray);
stParams.pDenominatorArray_ADR := ADR(aDenArray );
stParams.nDenominatorArray_SIZEOF := SIZEOF(aDenArray );
stParams.pTransferfunction2Data_ADR := ADR(aStTfData );
stParams.nTransferfunction2Data_SIZEOF := SIZEOF(aStTfData);
fbTansferfunction (fIn := fIn,
eMode := eMode,
stParams := stParams,
fOut => fOut,
eErrorId => eErrorId,
bError => bError);