ONNX export of XGBoost
![]() | Download Python samples A Zip archive containing all samples can be found here: Samples of ONNX export |
![]() | Limitation of the version of XGBoost The supported versions of XGBoost are limited due to the ONNX export behavior: version <= 1.5.2 or >= 1.7.4. |
XGB Regressor
# # Important Requirement: XGBoost version must be 1.7.4 or higher (otherwise onnxmltools 1.11.1 does not match)
import xgboost as xgb
from xgboost import XGBRegressor
from sklearn.datasets import make_regression
from skl2onnx.common.data_types import FloatTensorType
from onnxmltools.convert import convert_xgboost
import onnx
# # Generate data for regression
X, y = make_regression(n_samples=300, n_features=10, n_informative=10, n_targets=1)
# # Construct XGB-Model
model = XGBRegressor(objective='reg:squarederror',booster='gbtree', max_depth=3, learning_rate=0.08, n_estimators=300)
model.fit(X,y)
# # Convert model to ONNX
onnxfile = 'xgb-regressor.onnx'
initial_type = [('float_input', FloatTensorType([None, X.shape[1]]))]
onnx_model = convert_xgboost(model, initial_types=initial_type, target_opset=12)
onnx_model.graph.doc_string = "Converted from XGBoost ver."+xgb.__version__
# # Export to ONNX file
onnx.checker.check_model(onnx_model)
with open(onnxfile, "wb") as f:
f.write( onnx_model.SerializeToString())
f.close()
exit()
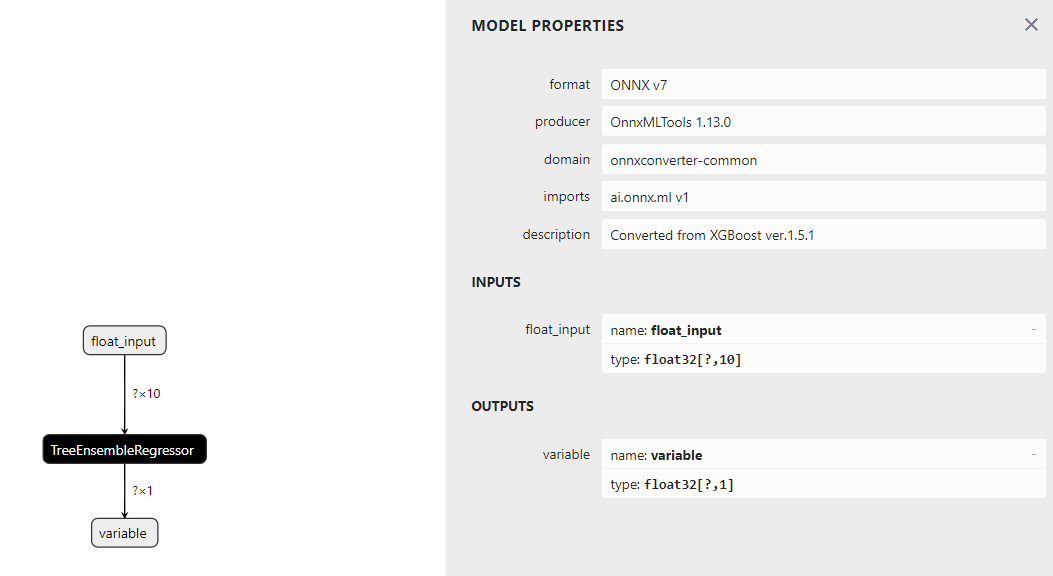
XGB Classifier
import onnx
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
import xgboost as xgb # # Important Requirement: XGBoost version must be 1.7.4 or higher (otherwise onnxmltools 1.11.1 does not match)
from xgboost import XGBClassifier
from skl2onnx.common.data_types import FloatTensorType
from onnxmltools.convert import convert_xgboost
X, y = load_iris(return_X_y = True)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size = 0.1)
model = XGBClassifier(objective= 'multi:softmax', learning_rate=0.03, max_depth=3, n_estimators=300, eval_metric='mlogloss', early_stopping_rounds=20, verbosity=1, use_label_encoder=False)
model.fit(X_train,y_train, eval_set=[(X_train, y_train), (X_test, y_test)], verbose=True)
onnxfile = 'xgb-iris.onnx'
# # Convert model to ONNX
initial_type = [('float_input', FloatTensorType([None, X.shape[1]]))]
onnx_model = convert_xgboost(model, initial_types=initial_type, target_opset=12)
onnx_model.graph.doc_string = "Converted from XGBoost ver."+xgb.__version__
# # Export to ONNX file
onnx.checker.check_model(onnx_model)
with open(onnxfile, "wb") as f:
f.write( onnx_model.SerializeToString())
f.close()
exit()
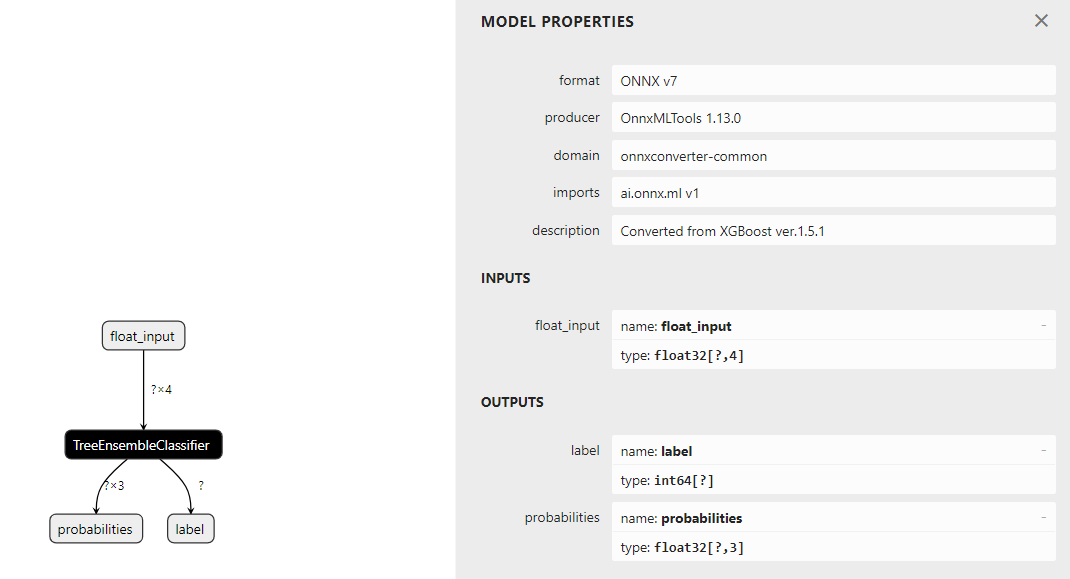
XGB binary classifier
from skl2onnx.common.data_types import FloatTensorType
from sklearn.model_selection import train_test_split
from sklearn.datasets import make_moons, make_circles, make_classification
import onnx
# # Important Requirement: XGBoost version must be 1.7.4 or higher (otherwise onnxmltools 1.11.1 does not match)
import xgboost as xgb
from xgboost import XGBClassifier
from onnxmltools.convert import convert_xgboost
# # Generate data for binary classification
X, y = make_moons(n_samples=300, noise=0.3, random_state=1)
# X, y = make_circles(n_samples=300, shuffle=True, noise=0.3, random_state=1, factor=0.8)
# # Construct XGB-Model
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size = 0.1)
model = XGBClassifier(objective= 'binary:logistic', learning_rate=0.03, max_depth=3, n_estimators=300, eval_metric='auc', early_stopping_rounds=20, verbosity=1, use_label_encoder=False)
model.fit(X_train,y_train, eval_set=[(X_train, y_train), (X_test, y_test)], verbose=True)
# # Convert model to ONNX
onnxfile = 'xgb-binary.onnx'
initial_type = [('float_input', FloatTensorType([None, X.shape[1]]))]
onnx_model = convert_xgboost(model, initial_types=initial_type, target_opset=12)
onnx_model.graph.doc_string = "Converted from XGBoost ver."+xgb.__version__
# # Export to ONNX file
onnx.checker.check_model(onnx_model)
with open(onnxfile, "wb") as f:
f.write( onnx_model.SerializeToString())
f.close()
exit()
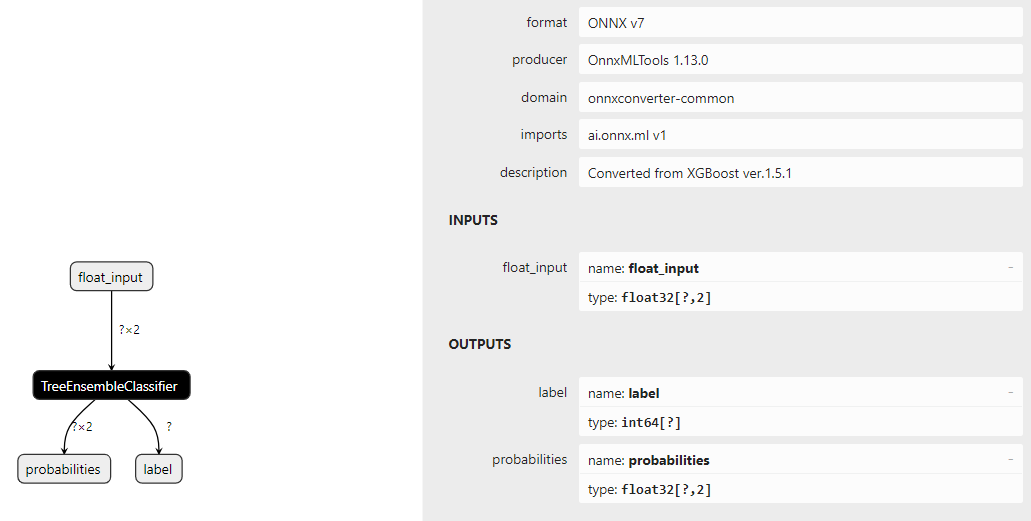