Dynamic weighing
This sample shows how the dynamic weighing process works with the Weighing PLC library.
Download: SampleDynamicWeighing (*.tnzip)
Description
The input signal, a noisy trapezoidal signal, is generated in the MAIN program using the method GenerateInputs()
with a signal generator. The simulated signal is transferred to the function block FB_DynamicWeighing (fbDynamicWeighing
), which forwards it internally to other function blocks. This includes filtering with the function block FB_WG_ComboFilter (fbComboFilter
), scaling with the function block FB_WG_Scaling (fbScale
) and evaluation with the function block FB_WG_Weighing (fbWeighing
).
Program parameters
The table below shows a list of important parameters for configuring the function blocks used.
Variable | Description | Default value |
---|---|---|
fRawAmplitudeSignal | Amplitude of the trapezoidal signal | 1000.0 |
fAbsoluteNoise | Absolute noise amount | 200.0 |
fFrequency | Base frequency of the trapezoidal signal | 1.0 Hz |
bActivateSlope | A drifting test signal is activated/deactivated | FALSE |
bAddWeight | Adds an offset value to the test signal once | FALSE |
eAutoTareType | Selection for automatic taring | E_WG_AutoTareType.eContinously |
The following global constants are defined.
Variable | Description | Default value |
---|---|---|
cOversamples | Number of oversamples of the input channel | 10 |
cSamplingRate | Sample rate of the input channel in Hz | 1000 |
Implementation:
First, the corresponding structures and function blocks are declared and initialized:
// Filter
stParamsComboFilter : ST_WG_ComboFilter :=
(
nOrder : =6,
fCutoff : =10.0,
fSamplingRate : =TO_LREAL(cSamplingRate),
nSamplesToFilter : =200,
bReset := FALSE
);
fbComboFilter := FB_WG_ComboFilter:=(stConfig := stParamsComboFilter);
// Scaling
stParamsScale : ST_WG_Scaling : =
(
fRawLow : =0.0,
fReferenceLow : =0.0,
fRawHigh : =1000.0,
fReferenceHigh : =100.0
);
fbScale : FB_WG_Scaling : =(stConfig : =stParamsScale, eTraceLevel : =TcEventSeverity.Info);
// Weighing
stParamsWeighing : ST_WG_Weighing : =
(
nWindowLength : =100,
Validation:=(nValidationSamples : =100, fThresholdWeight : =20.0, fMaxStd : =5.0, fMaxWeightDeviation : =0.0),
AutoTare : =(nValidationSamples : =100, fThresholdWeight : =10.0, fMaxStd:=1.0, fMaxWeightDeviation : =0.0)
);
fbWeighing : FB_WG_Weighing : =(stConfig : =stParamsWeighing);
In the implementation part, the function block instances are executed using the corresponding Call()
methods.
// Execute weighing
IF NOT fbComboFilter.Call(ADR(aInput), SIZEOF(aInput), ADR(aOutFilter), SIZEOF(aOutFilter)) THEN
SetError(fbComboFilter);
END_IF
IF NOT fbScale.Call(ADR(aOutFilter), SIZEOF(aOutFilter), ADR(aOutScaling), SIZEOF(aOutScaling)) THEN
SetError(fbScale);
END_IF
IF NOT fbWeighing.Call(ADR(aOutScaling), SIZEOF(aOutScaling)) THEN
SetError(fbWeighing);
END_IF
Automatic taring:
The instance fbScale
can be automatically tared via the fbWeighing
instance as follows:
// Execute AutoTare
IF NOT fbWeighing.AutoTare(fbScale, eAutoTareType)THEN
SetError(fbWeighing);
END_IF
Depending on the eAutoTareType initialization value, there is the following case distinction:
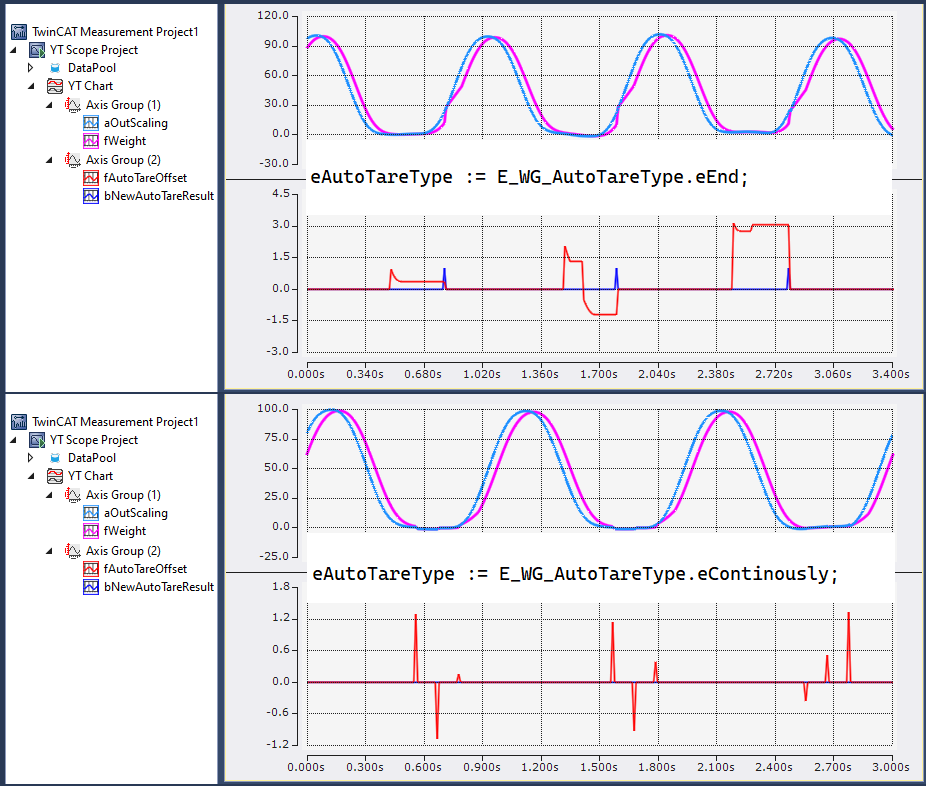
E_WG_AutoTareType.eEnd
(above): fbScale
is tared with the value fAutoTareOffset
if bNewAutoTareResult
is equal to TRUE
. E_WG_AutoTareType.eContinously
(below): fbScale
is tared with the value fAutoTareOffset
if fAutoTareOffset
is not equal to 0. After taring, a fbWeighing.Reset()
is automatically executed each time.