Calculation of individual spectral values
This sample implements as an example the possible uses of the function block FB_CMA_SparseSpectrum. Various window functions and scalings are presented for the possible calculations of DFT, magnitude and power values. Numerical effects in the detection are shown in two frequency portions of a generated signal: The first frequency corresponds to a multiple of the numerical resolution in the spectral range, while the second lies between two such values.
The source code for the sample is available for download from here: SparseSpectrum_Sample.zip
Block diagram
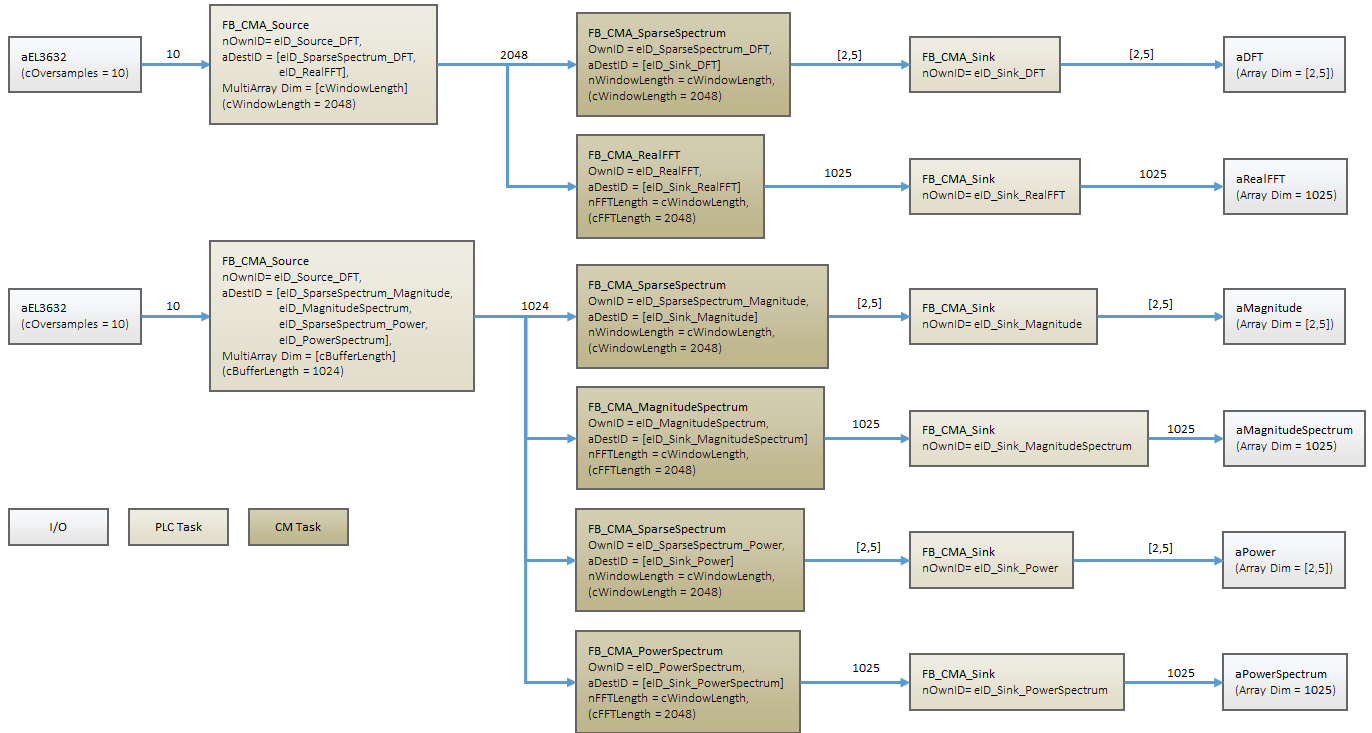
Program parameters
The table below shows a list of the important parameters for configuring the function blocks.
FFT-length | 2048 / 2048 / 2048 |
Window size | 2048 / 2048 / 2048 |
Buffer size | 2048 / 1024 / 1024 |
Window type | eCM_RectangularWindow / eCM_HannWindow / eCM_HannWindow |
Scaling method | eCM_NoScaling / eCM_PeakAmplitude / eCM_PeakAmplitude |
Type of spectral values | eCM_DFT / eCM_Magnitude / eCM_Power |
Configuration of the frequency bands
The GVL_Constants define the central parameters for initializing the algorithm and the properties of the generated signal.
VAR_GLOBAL CONSTANT
cSampleRate : LREAL := 10000; // Sample rate of input signal.
cWindowLength : UDINT := 2048; // Internal buffer size with 50% overlapping
cResolution : LREAL := cSamplerate / cWindowLength; // Frequency resolution
cBands : UDINT := 2; // Number of bands
cSetFrequency : ARRAY[1..cBands] OF LREAL := [ 41*cResolution, 413 ]; // Frequency in Hz; [ exact, intermediate ]
cSetAmplitude : ARRAY[1..cBands] OF LREAL := [ 1.0, 2.0 ]; // Peak amplitudes of sine signals
cBandWidth : UDINT := 5; // Computed bins per frequency.
cDFTBins : UDINT := cBandWidth * cBands; // Number of spectral bins, cBandWidth bins per frequency
END_VAR
The generated signal (MAIN.aBuffer
) consists of two frequency components. One is chosen with respect to the numerical resolution, i.e. it is a multiple of f = 10000 Hz/2048 = 4.8828125 Hz.
The second is chosen such that the peak lies between two spectral values and thus cannot be precisely represented. In order to be able to illustrate the numerical effects, a further four values are calculated around the respectively sought spectral values. The configuration takes place in der MAIN_CM
.
// Compute parameters, adjust if cDFTBins is changed.
FOR i := 1 TO cBands DO
k := LREAL_TO_DINT(cSetFrequency[i] / cResolution);
aDFTBins[(i-1) * cBandWidth + 1] := DINT_TO_UDINT(MAX(k-2,1));
aDFTBins[(i-1) * cBandWidth + 2] := DINT_TO_UDINT(MAX(k-1,1));
aDFTBins[(i-1) * cBandWidth + 3] := DINT_TO_UDINT(MIN(k+0,nyquist));
aDFTBins[(i-1) * cBandWidth + 4] := DINT_TO_UDINT(MIN(k+1,nyquist));
aDFTBins[(i-1) * cBandWidth + 5] := DINT_TO_UDINT(MIN(k+2,nyquist));
END_FOR
Visualization of the results
The sample includes an extensive TwinCAT measurement project in which the calculated spectral values from the function block FB_CMA_SparseSpectrum are compared with the reference algorithms (FB_CMA_RealFFT, FB_CMA_MagnitudeSpectrum, FB_CMA_PowerSpectrum). The difference between an excitation as a multiple of the numeric frequency resolution and a value between two such values is illustrated. The visualized frequency bands can be considered as a "zoom" of the corresponding area.
The following illustrations show the results from the comparison of the function blocks FB_CMA_RealFFT and FB_CMA_MagnitudeSpectrum.
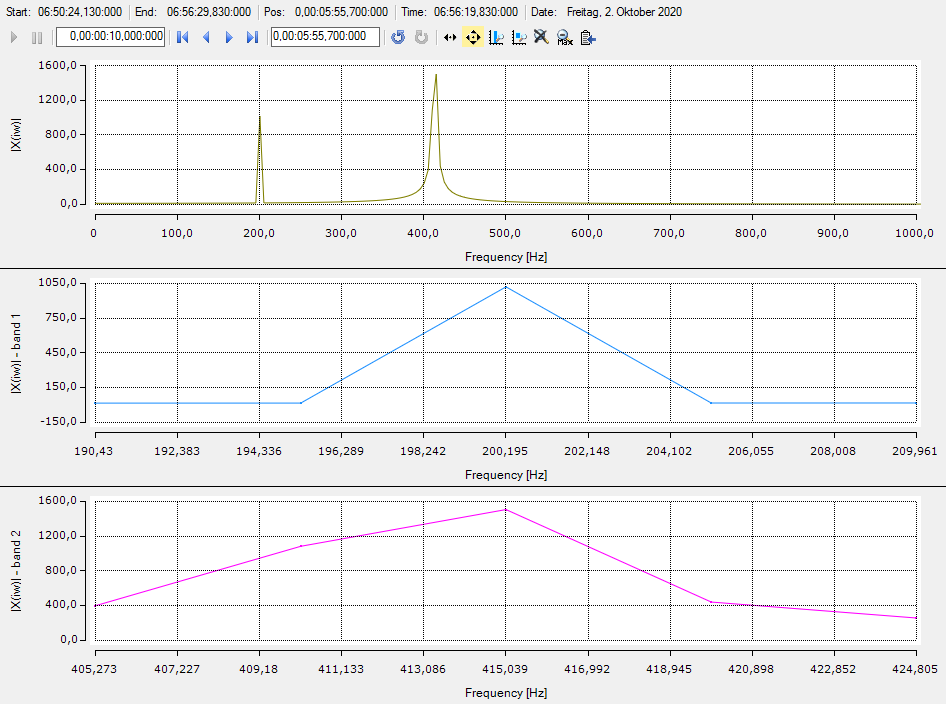
Spectrum of the FB_RealFFT (top) and the spectral values of bands 1 (center) and 2 (bottom).
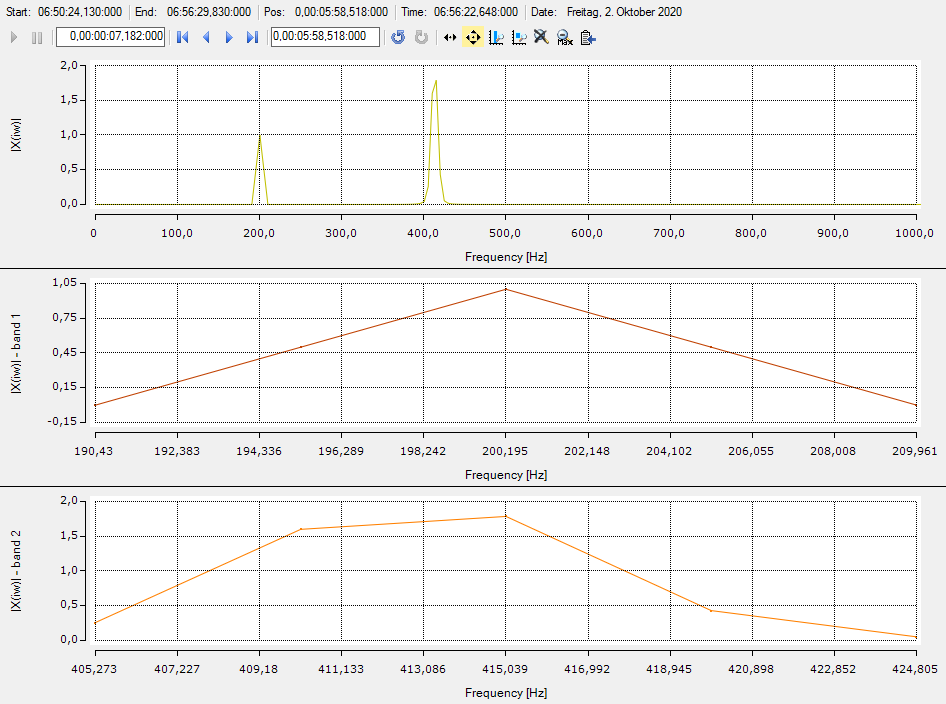
Magnitude spectrum of the function block FB_MagnitudeSpectrum (top) as well as the spectral values of bands 1 (center) and 2 (bottom).
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.4022.25 | PC or CX (x86, x64) | Tc3_CM, Tc3_CM_Base, Tc3_MultiArray |